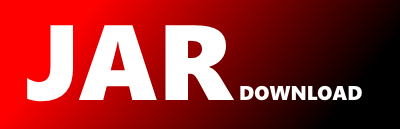
de.tsl2.nano.cursus.Grex Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of tsl2.nano.cursus Show documentation
Show all versions of tsl2.nano.cursus Show documentation
repeatable course/process (delta-processing-engine, event sourcing)
The newest version!
/*
* File: $HeadURL$
* Id : $Id$
*
* created by: Tom
* created on: 31.03.2017
*
* Copyright: (c) Thomas Schneider 2017, all rights reserved
*/
package de.tsl2.nano.cursus;
import java.io.Serializable;
import java.util.Collection;
import java.util.HashSet;
import java.util.Set;
import de.tsl2.nano.bean.BeanContainer;
import de.tsl2.nano.bean.BeanFindParameters;
import de.tsl2.nano.bean.def.Bean;
import de.tsl2.nano.core.util.MapUtil;
import de.tsl2.nano.core.util.Util;
/**
* Provides a generator for Res
* @author Tom
*
* @param instance type
* @param value type
*/
public class Grex implements Serializable {
private static final String WILDCARD = "*";
private static final long serialVersionUID = 1L;
protected Res genRes;
/** optional set of object ids */
protected Set
© 2015 - 2025 Weber Informatics LLC | Privacy Policy