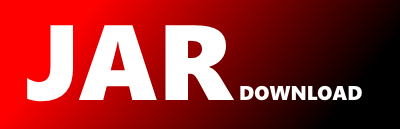
de.tsl2.nano.cursus.persistence.EGrex Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of tsl2.nano.cursus Show documentation
Show all versions of tsl2.nano.cursus Show documentation
repeatable course/process (delta-processing-engine, event sourcing)
package de.tsl2.nano.cursus.persistence;
import java.util.Collection;
import java.util.HashSet;
import java.util.Set;
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.Id;
import javax.persistence.JoinColumn;
import javax.persistence.OneToOne;
import de.tsl2.nano.bean.BeanContainer;
import de.tsl2.nano.bean.annotation.Attributes;
import de.tsl2.nano.bean.annotation.Presentable;
import de.tsl2.nano.bean.annotation.ValueExpression;
import de.tsl2.nano.bean.def.Bean;
import de.tsl2.nano.core.cls.BeanClass;
import de.tsl2.nano.cursus.Grex;
import de.tsl2.nano.service.util.IPersistable;
@Entity
@ValueExpression("{genRes}")
@Attributes(names = { "genRes", "validObjectIDs" })
@Presentable(label = "ΔGrex", icon = "icons/cascade.png")
public class EGrex extends Grex
© 2015 - 2025 Weber Informatics LLC | Privacy Policy