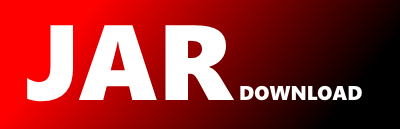
de.tsl2.nano.cursus.persistence.ERes Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of tsl2.nano.cursus Show documentation
Show all versions of tsl2.nano.cursus Show documentation
repeatable course/process (delta-processing-engine, event sourcing)
package de.tsl2.nano.cursus.persistence;
import java.util.Collection;
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.Id;
import javax.persistence.JoinColumn;
import javax.persistence.ManyToOne;
import javax.persistence.Transient;
import de.tsl2.nano.bean.BeanContainer;
import de.tsl2.nano.bean.annotation.Attributes;
import de.tsl2.nano.bean.annotation.Constraint;
import de.tsl2.nano.bean.annotation.ConstraintValueSet;
import de.tsl2.nano.bean.annotation.Presentable;
import de.tsl2.nano.bean.annotation.ValueExpression;
import de.tsl2.nano.core.cls.PrivateAccessor;
import de.tsl2.nano.core.util.Util;
import de.tsl2.nano.cursus.Res;
import de.tsl2.nano.service.util.IPersistable;
@Entity
@ValueExpression("@{typeName}:{objectid}->{path}")
@Attributes(names = { "typeName", "path", "objectid" })
@Presentable(label = "ΔRes", icon = "icons/images_all.png")
public class ERes extends Res
© 2015 - 2025 Weber Informatics LLC | Privacy Policy