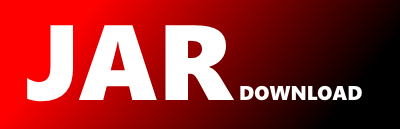
de.tsl2.nano.configuration.ConfigServiceBeanx Maven / Gradle / Ivy
/*
* File: $HeadURL$
* Id : $Id$
*
* created by: ts, Thomas Schneider
* created on: 12.07.2013
*
* Copyright: (c) Thomas Schneider 2013, all rights reserved
*/
package de.tsl2.nano.configuration;
import java.util.Collection;
import java.util.Hashtable;
import java.util.List;
import java.util.Map;
import java.util.Properties;
import javax.security.auth.Subject;
import de.tsl2.nano.bean.def.Bean;
import de.tsl2.nano.core.cls.BeanAttribute;
import de.tsl2.nano.core.cls.IAttribute;
import de.tsl2.nano.service.util.IGenericService;
import de.tsl2.nano.service.util.batch.Part;
import de.tsl2.nano.service.util.finder.AbstractFinder;
/**
* Configuration Service to configure bean types. only findBetween is implemented - only for the beanType
* {@link BeanAttribute}, to return a full list of available attributes.
*
* @author ts, Thomas Schneider
* @version $Revision$
*/
@SuppressWarnings("rawtypes")
public class ConfigServiceBean implements IGenericService {
Map, Collection>> entityCache = new Hashtable, Collection>>();
/**
* {@inheritDoc}
*/
@Override
public Collection findAll(Class beanType, Class... lazyRelations) {
return findAll(beanType, 0, Integer.MAX_VALUE, lazyRelations);
}
/**
* {@inheritDoc}
*/
@Override
public Collection findAll(Class beanType, int startIndex, int maxResult, Class... lazyRelations) {
throw new UnsupportedOperationException();
}
/**
* {@inheritDoc}
*/
@Override
public Collection findMembers(H holder, Class beanType, String attributeName, Class... lazyRelations) {
throw new UnsupportedOperationException();
}
/**
* {@inheritDoc}
*/
@Override
public Collection findHolders(T member, Class holderType, String attributeName, Class... lazyRelations) {
throw new UnsupportedOperationException();
}
/**
* {@inheritDoc}
*/
@Override
public T findByExample(T exampleBean, Class... lazyRelations) {
//TODO: constrain result
return (T) findAll(exampleBean.getClass()).iterator().next();
}
/**
* {@inheritDoc}
*/
@Override
public Collection findByExample(T exampleBean, boolean caseInsensitive, Class... lazyRelations) {
//TODO: constrain result
return (Collection) findAll(exampleBean.getClass());
}
/**
* {@inheritDoc}
*/
@Override
public Collection findByExampleLike(T exampleBean, boolean caseInsensitive, int startIndex, int maxResult, Class... lazyRelations) {
//TODO: constrain result
return (Collection) findAll(exampleBean.getClass(), startIndex, maxResult);
}
/**
* {@inheritDoc}
*/
@Override
public T findById(Class beanType, Object id, Class... lazyRelations) {
Collection all = findAll(beanType);
for (T bean : all) {
if (bean.hashCode() == (Integer) id) {
return bean;
}
}
return null;
}
/**
* {@inheritDoc}
*/
@Override
public Collection findBetween(T firstBean, T secondBean, Class... lazyRelations) {
return findBetween(firstBean, secondBean, true, 0, -1, lazyRelations);
}
/**
* {@inheritDoc}
*/
@SuppressWarnings("unchecked")
@Override
public Collection findBetween(T firstBean,
T secondBean,
boolean caseInsensitive,
int startIndex,
int maxResult,
Class... lazyRelations) {
if (firstBean instanceof IAttribute) {
Class declaringClass = ((IAttribute) firstBean).getDeclaringClass();
Bean bean = new Bean(declaringClass);
return bean.getAttributes();
}
return null;
}
/**
* {@inheritDoc}
*/
@Override
public int executeQuery(String queryString, boolean nativeQuery, Object[] args) {
throw new UnsupportedOperationException();
}
/**
* {@inheritDoc}
*/
@Override
public Collection> findByQuery(String queryString, boolean nativeQuery, Object[] args, Class... lazyRelations) {
throw new UnsupportedOperationException();
}
/**
* {@inheritDoc}
*/
@Override
public Collection> findByQuery(String queryString,
boolean nativeQuery,
int startIndex,
int maxresult,
Object[] args,
Map hints,
Class... lazyRelations) {
throw new UnsupportedOperationException();
}
/**
* {@inheritDoc}
*/
@Override
public Collection> findByQuery(String queryString,
boolean nativeQuery,
Map args,
Class... lazyRelations) {
throw new UnsupportedOperationException();
}
/**
* {@inheritDoc}
*/
@Override
public Object findItemByQuery(String queryString, boolean nativeQuery, Object[] args, Class... lazyRelations) {
throw new UnsupportedOperationException();
}
/**
* {@inheritDoc}
*/
@Override
public Object findValueByQuery(String queryString, boolean nativeQuery, Object... args) {
throw new UnsupportedOperationException();
}
/**
* {@inheritDoc}
*/
@Override
public T persist(T bean, Class... lazyRelations) {
throw new UnsupportedOperationException();
}
/**
* {@inheritDoc}
*/
@Override
public T persist(T bean, boolean refreshBean, boolean flush, Class... lazyRelations) {
throw new UnsupportedOperationException();
}
/**
* {@inheritDoc}
*/
@Override
public T persistNoTransaction(T bean, boolean refreshBean, boolean flush, Class... lazyRelations) {
throw new UnsupportedOperationException();
}
/**
* {@inheritDoc}
*/
@Override
public Collection persistCollection(Collection beans, Class... lazyRelations) {
throw new UnsupportedOperationException();
}
/**
* {@inheritDoc}
*/
@Override
public Object[] persistAll(Object... beans) {
throw new UnsupportedOperationException();
}
/**
* {@inheritDoc}
*/
@Override
public T refresh(T bean) {
throw new UnsupportedOperationException();
}
/**
* {@inheritDoc}
*/
@Override
public void remove(Object bean) {
}
/**
* {@inheritDoc}
*/
@Override
public void removeCollection(Collection
© 2015 - 2025 Weber Informatics LLC | Privacy Policy