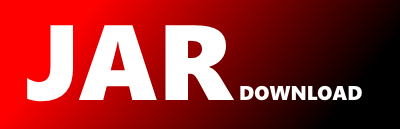
de.tsl2.nano.serialization.FileServiceBeanx Maven / Gradle / Ivy
/*
* File: $HeadURL$
* Id : $Id$
*
* created by: ts, Thomas Schneider
* created on: 12.07.2013
*
* Copyright: (c) Thomas Schneider 2013, all rights reserved
*/
package de.tsl2.nano.serialization;
import java.util.Collection;
import java.util.Hashtable;
import java.util.List;
import java.util.Map;
import java.util.Properties;
import javax.security.auth.Subject;
import de.tsl2.nano.core.ENV;
import de.tsl2.nano.core.util.FileUtil;
import de.tsl2.nano.service.util.IGenericService;
import de.tsl2.nano.service.util.batch.Part;
import de.tsl2.nano.service.util.finder.AbstractFinder;
/**
* Serialization Service for mocking or local storages. only some accessors are implemented
*
* @author ts, Thomas Schneider
* @version $Revision$
*/
@SuppressWarnings({ "rawtypes", "unchecked" })
public class FileServiceBean implements IGenericService {
Map, Collection>> entityCache = new Hashtable, Collection>>();
/**
* {@inheritDoc}
*/
@Override
public Collection findAll(Class beanType, Class... lazyRelations) {
return findAll(beanType, 0, Integer.MAX_VALUE, lazyRelations);
}
/**
* {@inheritDoc}
*/
@Override
public Collection findAll(Class beanType, int startIndex, int maxResult, Class... lazyRelations) {
Collection> allEntities = entityCache.get(beanType);
if (allEntities == null) {
allEntities = (Collection>) FileUtil.load(ENV.getConfigPath(beanType) + ".dat");
entityCache.put(beanType, allEntities);
}
return (Collection) allEntities;
}
/**
* {@inheritDoc}
*/
@Override
public Collection findMembers(H holder, Class beanType, String attributeName, Class... lazyRelations) {
throw new UnsupportedOperationException();
}
/**
* {@inheritDoc}
*/
@Override
public Collection findHolders(T member, Class holderType, String attributeName, Class... lazyRelations) {
throw new UnsupportedOperationException();
}
/**
* {@inheritDoc}
*/
@Override
public T findByExample(T exampleBean, Class... lazyRelations) {
//TODO: constrain result
return (T) findAll(exampleBean.getClass());
}
/**
* {@inheritDoc}
*/
@Override
public Collection findByExample(T exampleBean, boolean caseInsensitive, Class... lazyRelations) {
//TODO: constrain result
return (Collection) findAll(exampleBean.getClass());
}
/**
* {@inheritDoc}
*/
@Override
public Collection findByExampleLike(T exampleBean,
boolean caseInsensitive,
int startIndex,
int maxResult,
Class... lazyRelations) {
//TODO: constrain result
return (Collection) findAll(exampleBean.getClass(), startIndex, maxResult);
}
/**
* {@inheritDoc}
*/
@Override
public T findById(Class beanType, Object id, Class... lazyRelations) {
Collection all = findAll(beanType);
for (T bean : all) {
if (bean.hashCode() == (Integer) id) {
return bean;
}
}
return null;
}
/**
* {@inheritDoc}
*/
@Override
public Collection findBetween(T firstBean, T secondBean, Class... lazyRelations) {
//TODO: constrain result
return (Collection) findAll(firstBean.getClass());
}
/**
* {@inheritDoc}
*/
@Override
public Collection findBetween(T firstBean, T secondBean, boolean caseInsensitive, int startIndex,
int maxResult, Class... lazyRelations) {
//TODO: constrain result
return (Collection) findAll(firstBean.getClass());
}
/**
* {@inheritDoc}
*/
@Override
public int executeQuery(String queryString, boolean nativeQuery, Object[] args) {
throw new UnsupportedOperationException();
}
/**
* {@inheritDoc}
*/
@Override
public Collection> findByQuery(String queryString, boolean nativeQuery, Object[] args, Class... lazyRelations) {
throw new UnsupportedOperationException();
}
/**
* {@inheritDoc}
*/
@Override
public Collection> findByQuery(String queryString,
boolean nativeQuery,
int startIndex,
int maxresult,
Object[] args,
Map hints,
Class... lazyRelations) {
throw new UnsupportedOperationException();
}
/**
* {@inheritDoc}
*/
@Override
public Collection> findByQuery(String queryString,
boolean nativeQuery,
Map args,
Class... lazyRelations) {
throw new UnsupportedOperationException();
}
/**
* {@inheritDoc}
*/
@Override
public Object findItemByQuery(String queryString, boolean nativeQuery, Object[] args, Class... lazyRelations) {
throw new UnsupportedOperationException();
}
/**
* {@inheritDoc}
*/
@Override
public Object findValueByQuery(String queryString, boolean nativeQuery, Object... args) {
throw new UnsupportedOperationException();
}
/**
* {@inheritDoc}
*/
@Override
public T persist(T bean, Class... lazyRelations) {
return persist(bean, true, true);
}
/**
* {@inheritDoc}
*/
@Override
public T persist(T bean, boolean refreshBean, boolean flush, Class... lazyRelations) {
return persistNoTransaction(bean, true, true);
}
/**
* {@inheritDoc}
*/
@Override
public T persistNoTransaction(T bean, boolean refreshBean, boolean flush, Class... lazyRelations) {
Class> entityType = bean.getClass();
FileUtil.save(ENV.getConfigPath(entityType) + ".dat", this.entityCache.get(entityType));
return bean;
}
/**
* {@inheritDoc}
*/
@Override
public Collection persistCollection(Collection beans, Class... lazyRelations) {
throw new UnsupportedOperationException();
}
/**
* {@inheritDoc}
*/
@Override
public Object[] persistAll(Object... beans) {
for (int i = 0; i < beans.length; i++) {
persistNoTransaction(beans[i], true, true);
}
return beans;
}
/**
* {@inheritDoc}
*/
@Override
public T refresh(T bean) {
throw new UnsupportedOperationException();
}
/**
* {@inheritDoc}
*/
@Override
public void remove(Object bean) {
//save current list to file
persist(bean);
}
/**
* {@inheritDoc}
*/
@Override
public void removeCollection(Collection
© 2015 - 2025 Weber Informatics LLC | Privacy Policy