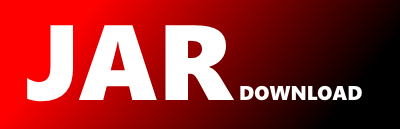
codegen.presenterfull.vm Maven / Gradle / Ivy
/*
* File: $HeadURL$
* Id : $Id$
*
* created by: Generated through velocity template (presenter.vm)
* created on: ----/--/-- (not filled with property 'time' to simplify version-diffs)
*
* ${copyright}
*/
package ${package};
#define($attKey) KEY_${util.toUpperCase(${att.Name})}#end
#define($attKeyTooltip) KEY_TOOLTIP_${util.toUpperCase(${att.Name})}#end
#define($attName) ATTR_${util.toUpperCase(${att.Name})}#end
import java.util.Collection;
import java.util.Hashtable;
import java.util.LinkedHashMap;
import java.util.LinkedList;
import java.util.Map;
import java.util.List;
import org.eclipse.core.databinding.observable.value.IObservableValue;
import org.eclipse.jface.viewers.Viewer;
import org.eclipse.swt.SWT;
import org.eclipse.swt.layout.GridData;
import org.eclipse.swt.graphics.Point;
import ${class.Clazz.CanonicalName};
import ${constClass};
import de.tsl2.nano.core.Messages;
import de.tsl2.nano.action.IAction;
import de.tsl2.nano.ui.presenter.IBeanController;
import de.tsl2.nano.ui.presenter.IBeanEditor;
import de.tsl2.nano.ui.presenter.IBeanLayout;
import de.tsl2.nano.ui.presenter.IBeanTableLayout;
import de.tsl2.nano.fielddescriptor.FieldDependency;
import de.tsl2.nano.fielddescriptor.FField;
import de.tsl2.nano.fielddescriptor.IComponentDescriptor;
import de.tsl2.nano.fielddescriptor.IFieldDescriptor;
import de.tsl2.nano.fielddescriptor.IFieldDependency;
import de.tsl2.nano.validator.MandatoryValidator;
import de.tsl2.nano.validator.AbstractValidator;
import de.tsl2.nano.validator.ObjectInListValidator;
import de.tsl2.nano.validator.ObjectInTableValidator;
import de.tsl2.nano.util.RegExpFormat;
import de.tsl2.nano.component.StructuredComponent;
import de.tsl2.nano.component.binding.Observables;
/**
* Default presenter for bean ${class.Name}!
*
* Extend this class to create a presenter for your special view.
*
* Generated do not modify!!!
*
* @author ts 22.11.2008
* @version $Revision$
*/
public class ${class.Name}UI implements ${class.Name}Const, IBeanEditor, IBeanLayout, IBeanTableLayout, IBeanController<${class.Name}> {
/** data bean */
protected ${class.Name} data;
/** all editors to modify the data bean */
protected Map editors;
/** all editor dependencies (dependencies between two editors) */
protected Map dependencies;
/** all actions to create in a form for the given bean */
protected Collection actions;
/** all visible columns, if the bean is shown in a list of a table */
protected Map columns;
/** layoutConstraints */
protected Map layoutConstraints;
/** filters all elements with the given attribute names */
protected Collection attributeNameFilter;
/** inverts the filter */
protected boolean useInverseFilter = false;
/**
* Constructor with specific bean data.
*
* @param data bean instance
*/
public ${class.Name}UI (${class.Name} data) {
this(data, new LinkedList());
}
/**
* Constructor with specific bean data.
*
* @param data bean instance
* @param attributeNameFilter attribute names to filter.
*/
public ${class.Name}UI (${class.Name} data, Collection attributeNameFilter) {
this(data, attributeNameFilter, false);
}
/**
* Constructor with specific bean data.
*
* @param data bean instance
* @param attributeNameFilter attribute names to filter.
* @param useInversFilter if true, only elements, that are not contained in the filter will be shown.
*/
public ${class.Name}UI (${class.Name} data, Collection attributeNameFilter,
boolean useInversFilter) {
this.data = data;
columns = new LinkedHashMap();
editors = new LinkedHashMap();
dependencies = new LinkedHashMap();
actions = new LinkedList();
layoutConstraints = new LinkedHashMap();
if (attributeNameFilter == null)
attributeNameFilter = new LinkedList();
this.attributeNameFilter = attributeNameFilter;
this.useInverseFilter = useInversFilter;
init(data);
//if an attribute filter was defined, use its order
if (!useInversFilter && attributeNameFilter instanceof List && ((List) attributeNameFilter).size() > 0)
swapToFilterOrder();
}
/**
* recreates the columns and editors map to use the order given by the attributeFilterNames. using a sortedmap
* doesn't work, so we have to recreate the map.
*/
protected void swapToFilterOrder() {
LinkedHashMap c = new LinkedHashMap(columns.size());
// key, value --> value, key (the column keys are the column headers! the attributenames are optional values)
LinkedHashMap c1 = new LinkedHashMap(columns.size());
for (String col : columns.keySet()) {
c1.put(columns.get(col), col);
}
LinkedHashMap e = new LinkedHashMap(editors.size());
for (String attributeName : attributeNameFilter) {
String attributeKey = getAttributeKey(attributeName);
c.put(c1.get(attributeKey), attributeName);
e.put(attributeKey, editors.get(attributeKey));
}
columns = c;
editors = e;
}
/**
* usable to map the simple attribute name to the combined key string
*
* @param attributeName attribute name
* @return key of attribute
*/
private String getAttributeKey(String attributeName) {
String className = data != null ? data.getClass().getSimpleName() : getClass().getSimpleName().substring(0,
getClass().getSimpleName().length() - 2);
return BeanAttribute.toFirstLower(className) + "." + attributeName;
}
/**
* this method creates and fills all collections to provide all gui descriptions
* for the given bean.
*
* Overwrite and "copy" initialization of the needed GUI components.
* Also initialize your own "dependencies" and "actions".
*
* @param data the bean instance
* @see #editors
* @see #dependencies
* @see #actions
* @see #columns
*/
protected void init(${class.Name} data) {
//table informations
#foreach( $att in $class.Attributes)
if (!hideAttribute(${attName}))
columns.put(Messages.getString(${attKey}), ${attName});
#end
//editor descriptions
if (data != null) {
#foreach( $att in $class.Attributes)
if (!hideAttribute(${attName})) {
IComponentDescriptor e = new FField(${attKey}, data, ${attName},
${util.getFormatter(${att})},
Messages.getString(${attKey}),
SWT.NONE, Messages.getString(${attKeyTooltip}),
${util.getEditorTypeString(${att})},
${util.getValidator(${att})},
null, null, true, true);
editors.put(${attKey}, e);
if (FField.isListType(e)) {
e.setValidator(evaluateRelations(e, null));
// e.setDefaultValue(StructuredComponent.DEFAULT_FIRSTITEM);
}
}
#end
}
}
/**
* Check if an attribute is hidden.
*
* @param name attributename the attribute name
* @return true, if there is a filter (size>0) and it does not contain the given name
*/
private boolean hideAttribute(String name) {
return (!useInverseFilter && attributeNameFilter.size() > 0 && !attributeNameFilter.contains(name))
|| (useInverseFilter && attributeNameFilter.contains(name));
}
/**
* {@inheritDoc}
*/
public Collection getActions() {
return actions;
}
/**
* {@inheritDoc}
*/
public Map getEditorDependencies() {
return dependencies;
}
/**
* {@inheritDoc}
*/
public Map getEditors() {
return editors;
}
/**
* {@inheritDoc}
*/
public IComponentDescriptor getAttributeEditor(String attributeName) {
return editors.get(attributeName);
}
/**
* Overwrite and return a proper {@link Viewer}.
*
* @param attributeName the attribute name
* @return always null
* @see de.tsl2.nano.data.ui.IBeanLayout#getAttributeComponent(String)
*/
public Viewer getAttributeComponent(String attributeName) {
return null;
}
/**
* Get the Layout constraints.
*
* @return Returns the layoutConstraints.
*/
public Map getLayoutConstraints() {
return layoutConstraints;
}
/**
* Overwrite and return a proper {@link GridData}.
*
* @param attributeName the attribute name
* @return always null
* @see de.tsl2.nano.data.ui.IBeanLayout#getAttributeLayout(String)
*/
public GridData getAttributeLayout(String attributeName) {
return layoutConstraints.get(attributeName);
}
/**
* {@inheritDoc}
*/
public Map getColumnAttributes() {
return columns;
}
/**
* {@inheritDoc}
*/
public Collection getEditorDependencyValues() {
return getEditorDependencies().values();
}
/**
* {@inheritDoc}
*/
public Collection getEditorValues() {
return getEditors().values();
}
/**
* {@inheritDoc}
*/
public Collection getColumns() {
return getColumnAttributes().keySet();
}
/**
* {@inheritDoc}
*/
public Map getColumnSort() {
return null;
}
/**
* {@inheritDoc}
*/
public ${class.Name} getBean() {
return data;
}
/**
* {@inheritDoc}
*/
public ${class.Name} save() {
${util.get("PERSIST_INSTANCE")}
return null;
}
/**
* Overwrite and implement this method to evaluate the list for the given editor.
*
* @param editor editor of type list to evaluate the relations for.
* @param tableLayout optional instance of a bean table layout to define the table columns.
* @return validator
*/
protected AbstractValidator evaluateRelations(IComponentDescriptor editor, IBeanTableLayout tableLayout) {
${util.get("INSTANTIATE_LAZY_RELATION")}
//OneToMany --> the relationObservable returns a collection
IObservableValue relationObservable = Observables.instance().getObservableValue(editor.getBean(),
editor.getBeanAttributeName());
//manyToOne --> call the service
// Class> type = (Class>) relationObservable.getValueType();
// if (!Collection.class.isAssignableFrom(type)) {
// if (!BeanUtil.isStandardType(type)) {
// IGenericService service = ServiceFactory.instance().getService(IGenericService.class);
// relationObservable = Observables.instance().getObservableValue(service.findAll(type));
// }
// }
//create the validator
if (FField.isDblClickType(editor)) {
if (tableLayout == null)
tableLayout = new IBeanTableLayout() {
public Collection getColumns() {
return new LinkedList();
}
public Map getColumnSort() {
return new Hashtable();
}
public Map getColumnAttributes() {
return new Hashtable();
}
};
return new ObjectInTableValidator(editor.getID(), relationObservable, tableLayout.getColumnAttributes());
} else {
return new ObjectInListValidator(editor.getID(), relationObservable);
}
}
/**
* {@inheritDoc}
*/
public Point getActionBounds() {
return null;
}
}