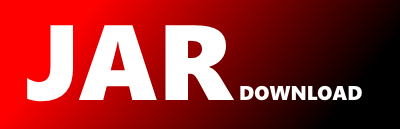
de.tsl2.nano.modelkit.Identified Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of tsl2.nano.modelkit Show documentation
Show all versions of tsl2.nano.modelkit Show documentation
TSL2 Framework to provide and use a structure of elements referenced by unique names - to declare a kit of logic in a json/yaml/xml text file
The newest version!
package de.tsl2.nano.modelkit;
import java.util.Arrays;
import java.util.List;
import java.util.Objects;
/**
* defines basics for name identifying.
*/
public interface Identified {
String getName();
default boolean equals0(Object o) {
return getClass().equals(o.getClass()) && hashCode() == o.hashCode();
}
default int hashCode0() {
return getName().hashCode();
}
static I get(List list, String name) {
Objects.requireNonNull(list, () -> "configuration error: no element with right type for '" + name + "' found");
// IMPROVE: to optimize performance use a hashmap instead...
// using loop instead of stream to enhance performance...
I e;
for (int i = 0; i < list.size(); i++) {
e = list.get(i);
if (e.getName().equals(name)) {
return e;
}
}
throw new IllegalStateException(name + " not found in list: " + Arrays.toString(list.toArray()));
}
void tagNames(String parent);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy