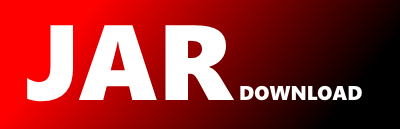
de.tsl2.nano.modelkit.impl.Group Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of tsl2.nano.modelkit Show documentation
Show all versions of tsl2.nano.modelkit Show documentation
TSL2 Framework to provide and use a structure of elements referenced by unique names - to declare a kit of logic in a json/yaml/xml text file
The newest version!
/*
* File: $HeadURL$
* Id : $Id$
*
* created by: Tom
* created on: 31.03.2017
*
* Copyright: (c) Thomas Schneider 2017, all rights reserved
*/
package de.tsl2.nano.modelkit.impl;
import java.util.Arrays;
import java.util.Comparator;
import java.util.List;
import java.util.Objects;
import java.util.function.BiFunction;
import java.util.stream.Collectors;
import de.tsl2.nano.modelkit.Identified;
import de.tsl2.nano.modelkit.Selectable;
import lombok.Getter;
import lombok.Setter;
/**
* Group, selecting its items through a selector and providing a
* comparator chain. Each comparator is asked, if it is the
* right one (canSelect) and can have sub-comparators used on compare-equality.
* For more informations,
*
* see {@link #Group(String, int, String, int, String, boolean, String...)}
* see {@link #sort(List)}
*/
public class Group extends AIdentified
implements Comparator, Selectable, BiFunction, List> {
static {
ModelKitLoader.registereElement(Group.class);
}
@Getter
@Setter
String selectorFact;
@Getter
@Setter
private List passFuncs;
@Getter
@Setter
private List compNames;
protected Group() {
}
/**
* creates a new Group. a group does actions in several passes on given items
*
* @param name unique name
* @param propertyNames any identified definitions/comparators, facts, funcs to be used as properties for your action.
*/
public Group(String name, String selectorFact, String... passFuncs) {
super(name);
this.selectorFact = selectorFact;
this.passFuncs = Arrays.asList(passFuncs);
}
public Group setComparators(String... compNames) {
this.compNames = Arrays.asList(compNames);
return this;
}
public Integer getPassCount() {
return passFuncs.size();
}
@Override
public List apply(Integer pass, List items) {
if (pass >= passFuncs.size())
return items;
return (List) get(passFuncs.get(pass), Func.class).eval(this, items);
}
@Override
public void validate() {
Objects.requireNonNull(passFuncs, "funcName must not be null: " + toString());
checkExistence(Func.class, passFuncs);
checkExistence(selectorFact, Fact.class);
checkExistence(Comp.class, compNames);
}
@Override
public void tagNames(String parent) {
super.tagNames(parent);
selectorFact = tag(parent, selectorFact);
tag(parent, passFuncs);
tag(parent, compNames);
}
@Override
public boolean canSelect(T item) {
return canSelect(selectorFact, item);
}
/**
* sorts its filtered items through its comparators
*/
public List sort(List items) {
List groupItems = filter(items);
groupItems.sort((c1, c2) -> compare(c1, c2));
return groupItems;
}
@Override
public int compare(T o1, T o2) {
int result = 0;
// IMPROVE: not performance optimized, searching each time through a list
List comparators = get(Comp.class);
for (String name : compNames) {
Comp comparator = Identified.get(comparators, name);
if (comparator.canSelect(Arrays.asList(o1, o2))) {
result = comparator.compare(o1, o2);
if (result != 0) {
break;
}
}
}
return result;
}
public List filter(List items) {
return items.stream().filter(t -> canSelect(t)).collect(Collectors.toList());
}
@Override
public String toString() {
return super.toString() + " (" + selectorFact + " -> " + compNames.toString() + ")";
}
String describe(String prefix) {
StringBuilder b = new StringBuilder(
prefix + super.toString() + " (if:" + selectorFact + " -> " + passFuncs + ")\n");
compNames.forEach(c -> b.append(get(c, Comp.class).describe(prefix + "\t\t")));
return b.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy