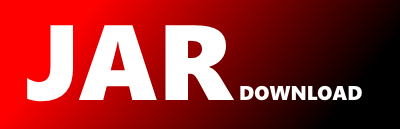
de.tsl2.nano.util.operation.NumericOperator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of tsl2.nano.operation Show documentation
Show all versions of tsl2.nano.operation Show documentation
TSL2 Framework Operation (abstract parser, conditioned functional or boolean operations - abstract structure for rule-engines)
/*
* File: $HeadURL$
* Id : $Id$
*
* created by: Tom
* created on: 22.10.2013
*
* Copyright: (c) Thomas Schneider 2013, all rights reserved
*/
package de.tsl2.nano.util.operation;
import java.math.BigDecimal;
import java.text.DecimalFormat;
import java.text.NumberFormat;
import java.util.HashMap;
import java.util.Map;
import de.tsl2.nano.action.CommonAction;
import de.tsl2.nano.action.IAction;
/**
* Numeric Operator as a sample implementation of {@link Operator}. Is able to do math operations on decimals (including
* currency symbols). Override {@link #createOperations()} to have more operation definitions.
*
* @author Tom
* @version $Revision$
*/
public class NumericOperator extends SOperator {
/**
* constructor
*/
public NumericOperator() {
this(null);
}
/**
* constructor
* @param values
*/
public NumericOperator(
Map values) {
super(CharSequence.class, createConverter(), values);
}
protected static IConverter createConverter() {
DecimalFormat fmt = (DecimalFormat) NumberFormat.getInstance();
fmt.setParseBigDecimal(true);
return new FromCharSequenceConverter(fmt);
}
/**
* define all possible operations. see {@link #operationDefs}
*/
@Override
@SuppressWarnings("serial")
protected void createOperations() {
syntax.put(KEY_OPERATION, "[-+*/%^]");
syntax.put(KEY_HIGH_OPERATION, "[*/%^]");
// syntax.put(KEY_DEFAULT_OPERAND, "0");
// syntax.put(KEY_DEFAULT_OPERATOR, "+");
operationDefs = new HashMap>();
addOperation("+", new CommonAction() {
@Override
public BigDecimal action() throws Exception {
return ((BigDecimal) parameters().getValue(0)).add(((BigDecimal) parameters().getValue(1)));
}
});
addOperation("-", new CommonAction() {
@Override
public BigDecimal action() throws Exception {
return ((BigDecimal) parameters().getValue(0)).subtract(((BigDecimal) parameters().getValue(1)));
}
});
addOperation("*", new CommonAction() {
@Override
public BigDecimal action() throws Exception {
return ((BigDecimal) parameters().getValue(0)).multiply(((BigDecimal) parameters().getValue(1)));
}
});
addOperation("/", new CommonAction() {
@Override
public BigDecimal action() throws Exception {
return ((BigDecimal) parameters().getValue(0)).divide(((BigDecimal) parameters().getValue(1)));
}
});
addOperation("%", new CommonAction() {
@Override
public BigDecimal action() throws Exception {
return new BigDecimal(((BigDecimal)parameters().getValue(0)).intValueExact() % ((BigDecimal)parameters().getValue(1)).intValueExact());
}
});
addOperation("^", new CommonAction() {
@Override
public BigDecimal action() throws Exception {
return ((BigDecimal) parameters().getValue(0)).pow(((BigDecimal)parameters().getValue(1)).intValueExact());
}
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy