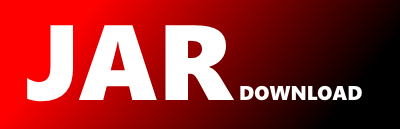
de.tsl2.nano.util.operation.NumericConditionOperator Maven / Gradle / Ivy
/*
* File: $HeadURL$
* Id : $Id$
*
* created by: Tom
* created on: 30.11.2013
*
* Copyright: (c) Thomas Schneider 2013, all rights reserved
*/
package de.tsl2.nano.util.operation;
import java.math.BigDecimal;
import java.util.Map;
import de.tsl2.nano.core.ENV;
import de.tsl2.nano.core.util.RegExUtil;
/**
* Combination of conditioning (including booleans) and numeric operator. result has to be of type {@link BigDecimal}.
* This implementation disables type generics of super classes. uses {@link Function} to calculate inside functions
* before.
*
* @author Tom
* @version $Revision$
*/
public class NumericConditionOperator extends ConditionOperator
© 2015 - 2025 Weber Informatics LLC | Privacy Policy