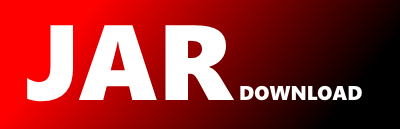
de.tsl2.nano.incubation.specification.AbstractRunnable Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of tsl2.nano.specification Show documentation
Show all versions of tsl2.nano.specification Show documentation
TSL2 Framework Specification (Pools of descripted and runnable Actions and Rules, Generic Tree)
package de.tsl2.nano.incubation.specification;
import java.util.ArrayList;
import java.util.Collection;
import java.util.HashMap;
import java.util.Iterator;
import java.util.LinkedHashMap;
import java.util.LinkedList;
import java.util.List;
import java.util.Map;
import java.util.Set;
import org.apache.commons.logging.Log;
import org.simpleframework.xml.Attribute;
import org.simpleframework.xml.Element;
import org.simpleframework.xml.ElementList;
import org.simpleframework.xml.ElementMap;
import org.simpleframework.xml.core.Commit;
import de.tsl2.nano.bean.def.BeanDefinition;
import de.tsl2.nano.bean.def.Constraint;
import de.tsl2.nano.core.ENV;
import de.tsl2.nano.core.cls.BeanClass;
import de.tsl2.nano.core.cls.IAttribute;
import de.tsl2.nano.core.log.LogFactory;
import de.tsl2.nano.core.util.FileUtil;
import de.tsl2.nano.core.util.NetUtil;
import de.tsl2.nano.core.util.NumberUtil;
import de.tsl2.nano.execution.IPRunnable;
/**
* runnable checking its arguments against constraints. provides checks against a specification/assertions. if member
* operation is an url (e.g. starting with file:) the url content will be used as operation.
*
* @param result type
* @author Tom, Thomas Schneider
* @version $Revision$
*/
public abstract class AbstractRunnable implements IPRunnable>, IPrefixed {
/** serialVersionUID */
private static final long serialVersionUID = 1L;
private static final Log LOG = LogFactory.getLog(AbstractRunnable.class);
@Attribute
protected String name;
@ElementMap(entry = "parameter", attribute = true, inline = true, keyType = String.class, key = "name", valueType = ParType.class, value = "type", required = false)
protected LinkedHashMap parameter;
@ElementMap(entry = "constraint", attribute = true, inline = true, keyType = String.class, key = "name", value = "definition", valueType = Constraint.class, required = false)
protected Map> constraints;
@ElementList(required = false, inline = true, type = Specification.class)
protected Collection specifications;
@Element
protected String operation;
transient protected String operationContent;
/**
* add this key to your arguments, if you want to fill the parameter not through key-names but through a sequence
*/
static final String KEY_ARGUMENTS_AS_SEQUENCE = "arguments as sequence";
public AbstractRunnable() {
super();
}
/**
* constructor
*
* @param operation
* @param parameter
*/
public AbstractRunnable(String name, String operation, LinkedHashMap parameter) {
this.name = name;
this.operation = operation;
this.parameter = parameter;
initDeserializing();
}
/**
* @return Returns the name.
*/
@Override
public String getName() {
return name;
}
@SuppressWarnings({ "unchecked", "rawtypes" })
protected Map createParameters(Class declaringClass) {
BeanDefinition> def = BeanDefinition.getBeanDefinition(declaringClass);
List attrs = def.getAttributes(false);
Map par = new LinkedHashMap<>();
for (IAttribute a : attrs) {
par.put(a.getName(), new ParType(a.getType()));
}
return par;
}
public static LinkedHashMap parameters(String... names) {
return (LinkedHashMap) createSimpleParameters(names);
}
protected static Map createSimpleParameters(String... names) {
Map par = new LinkedHashMap<>();
for (int i = 0; i < names.length; i++) {
par.put(names[i], new ParType(Object.class));
}
return par;
}
/**
* checks arguments against defined parameter and returns new map of arguments, defined by parameters. Use
* {@link #KEY_ARGUMENTS_AS_SEQUENCE} to define a sequential iteration of arguments (without using key names)
*
* @param arguments to be checked and collected
* @param strict if true, arguments not defined as parameter will throw an {@link IllegalArgumentException}.
*/
@Override
public Map checkedArguments(Map arguments, boolean strict) {
boolean asSequence = asSequence(arguments);
Map args = new LinkedHashMap();
Set keySet = arguments.keySet();
Set defs = parameter != null ? parameter.keySet() : arguments.keySet();
Iterator
© 2015 - 2025 Weber Informatics LLC | Privacy Policy