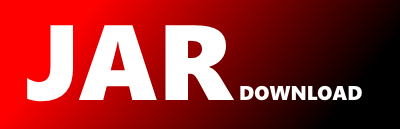
de.tsl2.nano.specification.Workflow Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of tsl2.nano.specification Show documentation
Show all versions of tsl2.nano.specification Show documentation
TSL2 Framework Specification (Pools of descripted and runnable Actions and Rules, Generic Tree)
The newest version!
package de.tsl2.nano.specification;
import java.io.BufferedWriter;
import java.io.File;
import java.util.Collection;
import java.util.LinkedList;
import java.util.Map;
import java.util.concurrent.ScheduledFuture;
import java.util.concurrent.TimeUnit;
import java.util.function.Consumer;
import org.simpleframework.xml.Attribute;
import de.tsl2.nano.bean.BeanUtil;
import de.tsl2.nano.bean.def.Bean;
import de.tsl2.nano.core.ENV;
import de.tsl2.nano.core.cls.BeanClass;
import de.tsl2.nano.core.util.DateUtil;
import de.tsl2.nano.core.util.FilePath;
import de.tsl2.nano.core.util.FileUtil;
import de.tsl2.nano.core.util.MapUtil;
import de.tsl2.nano.core.util.Util;
import de.tsl2.nano.execution.IPRunnable;
import de.tsl2.nano.util.Flow;
import de.tsl2.nano.util.Flow.ITask;
import de.tsl2.nano.util.SchedulerUtil;
/**
* workflow on a {@link Flow} starting through schedule expression (delay/period/end/TimeUnit) and loading data through given query rule.
* Each data row will go through flow of given type.
*
* @author ts
*/
public class Workflow implements Runnable {
@Attribute
String flowType;
@Attribute
String flowFileName;
@Attribute
String schedule;
@Attribute
String queryOrFileName;
Map context;
transient Collection flows = new LinkedList<>();
protected Workflow() {}
public Workflow(String flowFileName, String schedule, String queryOrFileName) {
this(Task.class.getName(), flowFileName, schedule, queryOrFileName);
}
public Workflow(String flowType, String flowFileName, String schedule, String queryOrFileName) {
super();
this.flowFileName = flowFileName;
this.flowType = flowType;
this.schedule = schedule;
this.queryOrFileName = queryOrFileName;
}
public ScheduledFuture> activate() {
if (schedule == null || schedule.equals("now")) {
run();
return null;
} else {
String[] time = schedule.split("[/]");
return SchedulerUtil.runAt(Integer.valueOf(time[0]), Integer.valueOf(time[1]), Integer.valueOf(time[2]), TimeUnit.valueOf(time[3]), this);
}
}
@Override
public void run() {
Class extends ITask> flowClass = BeanClass.load(flowType);
log("starting workflow [" + flowClass.getName() + "]");
Collection
© 2015 - 2025 Weber Informatics LLC | Privacy Policy