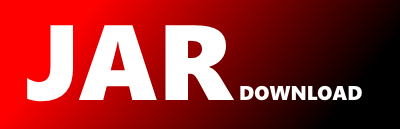
net.sf.tweety.logics.commons.analysis.AbstractMusEnumerator Maven / Gradle / Ivy
/*
* This file is part of "Tweety", a collection of Java libraries for
* logical aspects of artificial intelligence and knowledge representation.
*
* Tweety is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License version 3 as
* published by the Free Software Foundation.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this program. If not, see .
*
* Copyright 2016 The Tweety Project Team
*/
package net.sf.tweety.logics.commons.analysis;
import java.util.Collection;
import java.util.Collections;
import java.util.HashSet;
import java.util.LinkedList;
import java.util.List;
import java.util.Set;
import net.sf.tweety.commons.BeliefSet;
import net.sf.tweety.commons.Formula;
import net.sf.tweety.commons.util.SetTools;
/**
* Abstract implementation for MUes enumerators.
*
* @author Matthias Thimm
*
* @param the type of formulas
*/
public abstract class AbstractMusEnumerator implements MusEnumerator {
/* (non-Javadoc)
* @see net.sf.tweety.logics.commons.analysis.MusEnumerator#minimalInconsistentSubsets(java.util.Collection)
*/
@Override
public abstract Collection> minimalInconsistentSubsets(Collection formulas);
/* (non-Javadoc)
* @see net.sf.tweety.logics.commons.analysis.MusEnumerator#minimalCorrectionSubsets(java.util.Collection)
*/
public Set> minimalCorrectionSubsets(Collection formulas){
// we use the duality of minimal inconsistent subsets, minimal correction sets, and maximal consistent
// subsets to compute the set of maximal consistent subsets
Collection> mis = this.minimalInconsistentSubsets(formulas);
// makes sets out of this
Set> mi_sets = new HashSet>();
for(Collection m: mis)
mi_sets.add(new HashSet(m));
SetTools settools = new SetTools();
// get the minimal correction sets, i.e. irreducible hitting sets of minimal inconsistent subsets
Set> md_sets = settools.irreducibleHittingSets(mi_sets);
return md_sets;
}
/* (non-Javadoc)
* @see net.sf.tweety.logics.commons.analysis.MusEnumerator#maximalConsistentSubsets(java.util.Collection)
*/
@Override
public Collection> maximalConsistentSubsets(Collection formulas){
Set> md_sets = this.minimalCorrectionSubsets(formulas);
// every maximal consistent subset is the complement of a minimal correction set
Set> result = new HashSet>();;
Set tmp;
for(Collection ms: md_sets){
tmp = new HashSet(formulas);
tmp.removeAll(ms);
result.add(tmp);
}
return result;
}
/* (non-Javadoc)
* @see net.sf.tweety.logics.commons.analysis.MusEnumerator#isConsistent(net.sf.tweety.BeliefSet)
*/
@Override
public boolean isConsistent(BeliefSet beliefSet){
return this.isConsistent((Collection) beliefSet);
}
/* (non-Javadoc)
* @see net.sf.tweety.logics.commons.analysis.MusEnumerator#isConsistent(net.sf.tweety.Formula)
*/
@Override
public boolean isConsistent(S formula){
Collection c = new HashSet();
c.add(formula);
return this.isConsistent(c);
}
/* (non-Javadoc)
* @see net.sf.tweety.logics.commons.analysis.MusEnumerator#isConsistent(java.util.Collection)
*/
@Override
public boolean isConsistent(Collection formulas){
return this.minimalInconsistentSubsets(formulas).isEmpty();
}
/* (non-Javadoc)
* @see net.sf.tweety.logics.commons.analysis.MusEnumerator#getMiComponents(java.util.Collection)
*/
public Collection> getMiComponents(Collection formulas){
List> comp = new LinkedList>(this.minimalInconsistentSubsets(formulas));
boolean changed;
do{
changed = false;
for(int i = 0; i < comp.size(); i++){
for(int j = i+1; j< comp.size(); j++){
if(!Collections.disjoint(comp.get(i), comp.get(j))){
changed = true;
comp.get(i).addAll(comp.get(j));
comp.remove(j);
break;
}
}
if(changed) break;
}
}while(changed);
return comp;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy