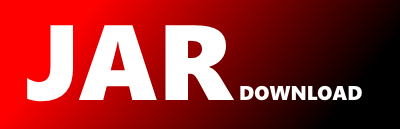
net.sf.xmlform.expression.ExpressionParser Maven / Gradle / Ivy
package net.sf.xmlform.expression;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
import net.sf.xmlform.expression.impl.formExpressionLexer;
import net.sf.xmlform.expression.impl.formExpressionParser;
import org.antlr.v4.runtime.BaseErrorListener;
import org.antlr.v4.runtime.CharStreams;
import org.antlr.v4.runtime.CodePointCharStream;
import org.antlr.v4.runtime.CommonTokenStream;
import org.antlr.v4.runtime.RecognitionException;
import org.antlr.v4.runtime.Recognizer;
/**
* @author Liu Zhikun
*/
public class ExpressionParser {
static public Factor parse(String expression) throws Exception{
if(expression==null||expression.length()==0){
Str str=new Str();
str.setString("\"\"");
return str;
}
StringBuilder errorSb=new StringBuilder();
BaseErrorListener errorListener=new InnerErrorListener(errorSb);
CodePointCharStream input = CharStreams.fromString(expression);
formExpressionLexer lexer = new formExpressionLexer(input);
lexer.removeErrorListeners();
lexer.addErrorListener(errorListener);
CommonTokenStream tokens = new CommonTokenStream(lexer);
formExpressionParser parser = new formExpressionParser(tokens);
parser.setBuildParseTree(false);
parser.removeErrorListeners();
parser.addErrorListener(errorListener);
try {
Factor factor=parser.program().factor;
if(errorSb.length()>0) {
throw new ExpressionException("Expression ["+expression+"] "+errorSb.toString()+". ");
}
return factor;
} catch (RecognitionException e) {
throw new ExpressionException(e.getLocalizedMessage(),e);
}
}
static public Set getDependFields(Factor factor){
Set box=new HashSet();
getDepends(factor,box);
return box;
}
static private void getDepends(Factor factor,Set box){
if(factor instanceof Field)
box.add(((Field)factor).getName());
else if(factor instanceof Function)
getDependsFromFun((Function)factor,box);
}
static private void getDependsFromFun(Function fun,Set box){
List args=fun.getArguments();
int size=args.size();
for(int i=0;i recognizer, Object offendingSymbol, int line,
int charPositionInLine, String msg, RecognitionException e) {
if(errorSb.length()>0)
errorSb.append("; ");
errorSb.append("line: "+line+", pos: "+charPositionInLine+": "+msg);
}
};
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy