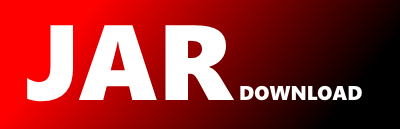
net.sf.xmlform.util.TimeUtils Maven / Gradle / Ivy
package net.sf.xmlform.util;
import java.sql.Date;
import java.sql.Time;
import java.sql.Timestamp;
import java.time.LocalDate;
import java.time.LocalDateTime;
import java.time.LocalTime;
import java.time.YearMonth;
import java.time.format.DateTimeFormatter;
public class TimeUtils {
private static DateTimeFormatter localDateParseFormatter=DateTimeFormatter.ofPattern("yyyy-M-d");
private static DateTimeFormatter localDateFormatter=DateTimeFormatter.ofPattern("yyyy-MM-dd");
private static DateTimeFormatter localTimeParseFormatter=DateTimeFormatter.ofPattern("H:m:s");
private static DateTimeFormatter localTimeMicroParseFormatter=DateTimeFormatter.ofPattern("H:m:s.SSS");
private static DateTimeFormatter localTimeFormatter=DateTimeFormatter.ofPattern("HH:mm:ss");
private static DateTimeFormatter localDateTimeParseFormatter=DateTimeFormatter.ofPattern("yyyy-M-d H:m:s");
private static DateTimeFormatter localDateTimeTParseFormatter=DateTimeFormatter.ofPattern("yyyy-M-d'T'H:m:s");
private static DateTimeFormatter localDateTimeMicroParseFormatter=DateTimeFormatter.ofPattern("yyyy-M-d H:m:s.SSS");
private static DateTimeFormatter localDateTimeMicroTParseFormatter=DateTimeFormatter.ofPattern("yyyy-M-d'T'H:m:s.SSS");
private static DateTimeFormatter localDateTimeFormatter=DateTimeFormatter.ofPattern("yyyy-MM-dd HH:mm:ss");
private static DateTimeFormatter localYearMonthParseFormatter=DateTimeFormatter.ofPattern("yyyy-M");
private static DateTimeFormatter localYearMonthFormatter=DateTimeFormatter.ofPattern("yyyy-MM");
static public LocalDate parseLocalDate(String source){
if(source==null||source.length()==0)
return null;
return LocalDate.parse(source, localDateParseFormatter);
}
static public String formatLocalDate(LocalDate date){
if(date==null)
return null;
return date.format(localDateFormatter);
}
static public LocalDate convertToLocalDate(Object value) {
if(value==null)
return null;
LocalDate localDate=null;
if(value instanceof LocalDate) {
localDate=(LocalDate)value;
} else if(value instanceof Timestamp){
Timestamp ts=(Timestamp)value;
localDate=ts.toLocalDateTime().toLocalDate();
}else if(value instanceof Date) {
Date date=(Date)value;
localDate=date.toLocalDate();
}else {
throw new IllegalArgumentException("Invalid date "+value);
}
return localDate;
}
static public boolean isLocalDate(String source){
try{
parseLocalDate(source);
return true;
}catch(Exception e){
return false;
}
}
static public LocalTime parseLocalTime(String source){
if(source==null||source.length()==0)
return null;
if(source.indexOf(".")>0)
return LocalTime.parse(source, localTimeMicroParseFormatter);
return LocalTime.parse(source, localTimeParseFormatter);
}
static public String formatLocalTime(LocalTime time){
if(time==null)
return null;
return time.format(localTimeFormatter);
}
static public boolean isLocalTime(String source){
try{
parseLocalTime(source);
return true;
}catch(Exception e){
return false;
}
}
static public LocalTime convertToLocalTime(Object value) {
if(value==null)
return null;
LocalTime localTime=null;
if(value instanceof LocalTime) {
localTime=(LocalTime)value;
} else if(value instanceof Time){
Time ts=(Time)value;
localTime=ts.toLocalTime();
}else {
throw new IllegalArgumentException("Invalid time "+value);
}
return localTime;
}
static public LocalDateTime parseLocalDateTime(String source){
if(source==null||source.length()==0)
return null;
if(source.indexOf(".")>0) {
if(source.indexOf('T')>0)
return LocalDateTime.parse(source, localDateTimeMicroTParseFormatter);
return LocalDateTime.parse(source, localDateTimeMicroParseFormatter);
}
if(source.indexOf('T')>0)
return LocalDateTime.parse(source, localDateTimeTParseFormatter);
return LocalDateTime.parse(source, localDateTimeParseFormatter);
}
static public String formatLocalDateTime(LocalDateTime time){
if(time==null)
return null;
return time.format(localDateTimeFormatter);
}
static public boolean isLocalDateTime(String source){
try{
parseLocalDateTime(source);
return true;
}catch(Exception e){
return false;
}
}
static public LocalDateTime convertToLocalDateTime(Object value) {
if(value==null)
return null;
LocalDateTime localDate=null;
if(value instanceof LocalDateTime) {
localDate=(LocalDateTime)value;
} else if(value instanceof Timestamp){
Timestamp ts=(Timestamp)value;
localDate=ts.toLocalDateTime();
}else {
throw new IllegalArgumentException("Invalid datetime "+value);
}
return localDate;
}
static public YearMonth parseYearMonth(String source){
if(source==null||source.length()==0)
return null;
return YearMonth.parse(source, localYearMonthParseFormatter);
}
static public String formatYearMonth(YearMonth time){
if(time==null)
return null;
return time.format(localYearMonthFormatter);
}
static public boolean isYearMonth(String source){
try{
parseYearMonth(source);
return true;
}catch(Exception e){
return false;
}
}
static public YearMonth convertToYearMonth(Object value) {
if(value==null)
return null;
YearMonth yearMonth=null;
if(value instanceof YearMonth) {
yearMonth=(YearMonth)value;
} else if(value instanceof String){
return parseYearMonth((String)value);
}else {
throw new IllegalArgumentException("Invalid date "+value);
}
return yearMonth;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy