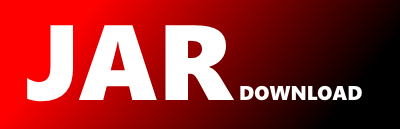
net.sf.xolite.ElementText Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of xo-lite Show documentation
Show all versions of xo-lite Show documentation
This project provides a lightweight framework to
serialize/deserialize (or marshall/unmarshall) java objects into
XML. The implementation is based on standard SAX (Simple Api for
Xml) but it follows a original approach based on the strong data
encapsulation paradigm of Object Oriented (OO)
programming.
The newest version!
/*-------------------------------------------------------------------------
Copyright 2006 Olivier Berlanger
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
-------------------------------------------------------------------------*/
package net.sf.xolite;
import java.awt.Dimension;
import java.awt.Point;
import java.awt.Rectangle;
import java.util.Date;
/**
* A helper class to get formatted value out of XML element text.
*
* @author Olivier Berlanger
*/
public class ElementText {
/**
* Get the current text from the given parser.
* If there is no current text or if this text is only made of white spaces, null is returned.
*
* @param parser
* the parser.
* @return the text of the current element of the given parser.
*/
public static String getString(XMLEventParser parser) throws XMLParseException {
return getString(null, parser, false);
}
/**
* Get the current text from the given parser.
* If there is no current text or if this text is only made of white spaces, the given default value is returned.
*
* @param defaultValue
* the value to return if no text is defined.
* @param parser
* the parser.
* @return the text of the current element of the given parser.
*/
public static String getString(String defaultValue, XMLEventParser parser) throws XMLParseException {
return getString(defaultValue, parser, false);
}
/**
* Get the current text from the given parser.
* If there is no current text or if this text is only made of white spaces, an exception is throw saying that this text is
* mandatory.
*
* @param parser
* the parser.
* @return the text of the current element of the given parser.
* @throws XMLParseException
* if no text is defined.
*/
public static String getMandatoryString(XMLEventParser parser) throws XMLParseException {
return getString(null, parser, true);
}
/**
* Get a multi-line text.
* This method removes from the given multi-line text the witespace that was added for indentation at the begin of each text
* line. This method does the invert operation of the XMLSerializer.charactersMultiLine(String text)
method.
*
* @param defaultValue
* the value to return if no text is defined.
* @param parser
* the parser.
* @return the text of the current element of the given parser.
* @see XMLSerializer#charactersMultiLine(String)
*/
public static String getMultilineText(String defaultValue, XMLEventParser parser) throws XMLParseException {
return getMultilineText(defaultValue, parser, false);
}
/**
* Get a mandatory multi-line text.
* This method removes from the given multi-line text the witespace that was added for indentation at the begin of each text
* line. This method does the invert operation of the XMLSerializer.charactersMultiLine(String text)
method.
*
* If there is no current text or if this text is only made of white spaces, an exception is throw saying that this text is
* mandatory.
*
*
* @param parser
* the parser.
* @return the text of the current element of the given parser.
* @throws XMLParseException
* if no text is defined.
* @see XMLSerializer#charactersMultiLine(String)
*/
public static String getMandatoryMultilineText(XMLEventParser parser) throws XMLParseException {
return getMultilineText(null, parser, true);
}
public static int getInt(int defaultValue, XMLEventParser parser) throws XMLParseException {
return getInt(defaultValue, parser, false);
}
public static int getMandatoryInt(XMLEventParser parser) throws XMLParseException {
return getInt(0, parser, true);
}
public static > T getEnum(T defaultValue, Class enumType, XMLEventParser parser)
throws XMLParseException {
return getEnum(defaultValue, enumType, parser, false);
}
public static > T getMandatoryEnum(Class enumType, XMLEventParser parser) throws XMLParseException {
return getEnum(null, enumType, parser, true);
}
public static double getDouble(double defaultValue, XMLEventParser parser) throws XMLParseException {
return getDouble(defaultValue, parser, false);
}
public static double getMandatoryDouble(XMLEventParser parser) throws XMLParseException {
return getDouble(0.0, parser, true);
}
public static int[] getIntArray(int[] defaultValue, XMLEventParser parser) throws XMLParseException {
return getIntArray(defaultValue, parser, false);
}
public static int[] getMandatoryIntArray(XMLEventParser parser) throws XMLParseException {
return getIntArray(null, parser, true);
}
public static long getLong(long defaultValue, XMLEventParser parser) throws XMLParseException {
return getLong(defaultValue, parser, false);
}
public static long getMandatoryLong(XMLEventParser parser) throws XMLParseException {
return getLong(0, parser, true);
}
public static boolean getBoolean(boolean defaultValue, XMLEventParser parser) throws XMLParseException {
return getBoolean(defaultValue, parser, false);
}
public static boolean getMandatoryBoolean(XMLEventParser parser) throws XMLParseException {
return getBoolean(false, parser, true);
}
public static Point getPoint(Point defaultValue, XMLEventParser parser) throws XMLParseException {
return getPoint(defaultValue, false, parser);
}
public static Point getMandatoryPoint(XMLEventParser parser) throws XMLParseException {
return getPoint(null, true, parser);
}
public static Dimension getDimension(Dimension defaultValue, XMLEventParser parser) throws XMLParseException {
return getDimension(defaultValue, false, parser);
}
public static Dimension getMandatoryDimension(XMLEventParser parser) throws XMLParseException {
return getDimension(null, true, parser);
}
public static Rectangle getRectangle(Rectangle defaultValue, XMLEventParser parser) throws XMLParseException {
return getRectangle(defaultValue, false, parser);
}
public static Rectangle getMandatoryRectangle(XMLEventParser parser) throws XMLParseException {
return getRectangle(null, true, parser);
}
public static Date getDate(String pattern, Date defaultValue, XMLEventParser parser) throws XMLParseException {
return getDate(pattern, defaultValue, false, parser);
}
public static Date getMandatoryDate(String pattern, XMLEventParser parser) throws XMLParseException {
return getDate(pattern, null, true, parser);
}
protected static String getString(String defaultValue, XMLEventParser parser, boolean mandatory) throws XMLParseException {
String text = defaultValue;
String elementText = getTrimmedText(mandatory, parser);
if (elementText != null) {
text = elementText;
}
return text;
}
protected static String getMultilineText(String defaultValue, XMLEventParser parser, boolean mandatory)
throws XMLParseException {
String multilineText = defaultValue;
String text = parser.getElementText();
if ((text != null) && text.trim().equals("")) text = null;
if (text == null) {
if (mandatory) {
parser.throwParseException("Element text is mandatory", null);
}
} else {
multilineText = trimMultiLine(text);
}
return multilineText;
}
protected static boolean getBoolean(boolean defaultValue, XMLEventParser parser, boolean mandatory)
throws XMLParseException {
boolean result = defaultValue;
String elementText = getTrimmedText(mandatory, parser);
if (elementText != null) {
result = Attributes.stringToBoolean(elementText);
}
return result;
}
protected static int getInt(int defaultValue, XMLEventParser parser, boolean mandatory) throws XMLParseException {
int result = defaultValue;
String elementText = getTrimmedText(mandatory, parser);
if (elementText != null) {
try {
result = Integer.parseInt(elementText);
} catch (Exception e) {
parser.throwParseException("Element text '" + elementText + "' must be a coma-separated list of integers", e);
}
}
return result;
}
protected static > T getEnum(T defaultValue, Class enumType, XMLEventParser parser, boolean mandatory)
throws XMLParseException {
T result = defaultValue;
String elementText = getTrimmedText(mandatory, parser);
if (elementText != null) {
try {
result = Enum.valueOf(enumType, elementText);
} catch (Exception e) {
parser.throwParseException("Element text '" + elementText + "' must a enum value of type " + enumType, e);
}
}
return result;
}
protected static double getDouble(double defaultValue, XMLEventParser parser, boolean mandatory) throws XMLParseException {
double result = defaultValue;
String elementText = getTrimmedText(mandatory, parser);
if (elementText != null) {
try {
result = Double.parseDouble(elementText);
} catch (Exception e) {
parser.throwParseException("Element text '" + elementText + "' must have an Double value.", e);
}
}
return result;
}
protected static int[] getIntArray(int[] defaultValue, XMLEventParser parser, boolean mandatory) throws XMLParseException {
int[] result = defaultValue;
String elementText = getTrimmedText(mandatory, parser);
if (elementText != null) {
try {
result = Attributes.stringToIntArray(elementText);
} catch (Exception e) {
parser.throwParseException("Element text '" + elementText + "' must be a coma-separated list of integers", e);
}
}
return result;
}
protected static long getLong(long defaultValue, XMLEventParser parser, boolean mandatory) throws XMLParseException {
long result = defaultValue;
String elementText = getTrimmedText(mandatory, parser);
if (elementText != null) {
try {
result = Long.parseLong(elementText);
} catch (Exception e) {
parser.throwParseException("Element text '" + elementText + "' must have an Long value.", e);
}
}
return result;
}
protected static Dimension getDimension(Dimension defaultValue, boolean mandatory, XMLEventParser parser)
throws XMLParseException {
Dimension result = defaultValue;
String elementText = getTrimmedText(mandatory, parser);
if (elementText != null) {
try {
int[] vals = Attributes.stringToIntArray(elementText);
if (vals.length != 2) {
throw new IllegalArgumentException("Bad dimension value --> it should be 2 integers, not " + vals.length);
}
result = new Dimension(vals[0], vals[1]);
} catch (Exception e) {
parser.throwParseException("Element text '" + elementText
+ "' must be a Dimension (coma-separated list of 2 integers)", e);
}
}
return result;
}
protected static Point getPoint(Point defaultValue, boolean mandatory, XMLEventParser parser) throws XMLParseException {
Point result = defaultValue;
String elementText = getTrimmedText(mandatory, parser);
if (elementText != null) {
try {
int[] vals = Attributes.stringToIntArray(elementText);
if (vals.length != 2) {
throw new IllegalArgumentException("Bad point value --> it should be 2 integers, not " + vals.length);
}
result = new Point(vals[0], vals[1]);
} catch (Exception e) {
parser.throwParseException("Element text '" + elementText
+ "' must be a Point (coma-separated list of 2 integers)", e);
}
}
return result;
}
protected static Rectangle getRectangle(Rectangle defaultValue, boolean mandatory, XMLEventParser parser)
throws XMLParseException {
Rectangle result = defaultValue;
String elementText = getTrimmedText(mandatory, parser);
if (elementText != null) {
try {
int[] vals = Attributes.stringToIntArray(elementText);
if (vals.length != 4) {
throw new IllegalArgumentException("Bad rectangle value --> it should be 4 integers, not " + vals.length);
}
result = new Rectangle(vals[0], vals[1], vals[2], vals[3]);
} catch (Exception e) {
parser.throwParseException("Element text '" + elementText
+ "' must be a Rectangle (coma-separated list of 4 integers)", e);
}
}
return result;
}
protected static Date getDate(String pattern, Date defaultValue, boolean mandatory, XMLEventParser parser)
throws XMLParseException {
Date result = defaultValue;
String elementText = getTrimmedText(mandatory, parser);
if (elementText != null) {
try {
result = Attributes.stringToDate(elementText, pattern);
} catch (Exception e) {
parser.throwParseException("Element text '" + elementText + "' must be a Date with pattern [" + pattern + "]",
e);
}
}
return result;
}
protected static String getTrimmedText(boolean mandatory, XMLEventParser parser) throws XMLParseException {
String text = parser.getElementText();
if (text != null) {
text = text.trim();
if (text.equals("")) text = null;
}
if ((text == null) && mandatory) {
parser.throwParseException("Element text is mandatory", null);
}
return text;
}
/**
* Remove extra whitespaces that were added by the XMLSerializer.charactersMultiLine(text) method.
* Each line of the string is 'smartly trimmed' to get back the input text. If the given string contains only one line (new
* 'new-line' characters) it is simply trimmed.
*
* @param str
* The multi-line string to be trimmed at each line.
* @return The input string trimmed at each line.
*/
public static final String trimMultiLine(String str) {
if (str == null) return null;
if (str.indexOf("\n") < 0) return str.trim();
String[] lines = str.split("\n");
int nbrLine = lines.length;
String line;
char ch;
// get start index
int startIndex = Integer.MAX_VALUE;
int j;
for (int i = 0; i < nbrLine; i++) {
line = lines[i];
int lineLen = line.length();
for (j = 0; j < lineLen; j++) {
if (line.charAt(j) > 32) {
if (((j > 0) || (i > 0)) && (startIndex > j)) startIndex = j;
break;
}
}
}
if (startIndex == Integer.MAX_VALUE) startIndex = 0;
// add trimmed lines to a buffer
StringBuffer sb = new StringBuffer(str.length());
// eat leading white lines
int start = 0;
for (int i = 0; i < nbrLine; i++) {
if (!Attributes.isEmpty(lines[i])) {
start = i;
break;
}
}
for (int i = start; i < nbrLine; i++) {
line = lines[i];
int lineLen = line.length();
// add new line
if (i > start) sb.append('\n');
// get the trimmed end index
int endIndex = lineLen;
for (j = lineLen - 1; j >= 0; j--) {
if (line.charAt(j) > 32) {
endIndex = j + 1;
break;
}
}
// add the line
for (j = 0; j < endIndex; j++) {
ch = line.charAt(j);
if (j < startIndex) {
if (ch > 32) sb.append(ch);
} else {
sb.append(ch);
}
}
}
return sb.toString();
}
public static void simpleElementMultiline(String uri, String tag, String text, XMLSerializer serilalizer)
throws XMLSerializeException {
serilalizer.startElement(uri, tag);
serilalizer.charactersMultiLine(text);
serilalizer.endElement(uri, tag);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy