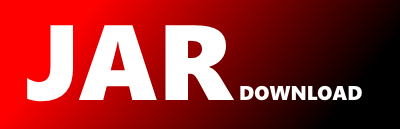
net.sf.xolite.XMLSerializer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of xo-lite Show documentation
Show all versions of xo-lite Show documentation
This project provides a lightweight framework to
serialize/deserialize (or marshall/unmarshall) java objects into
XML. The implementation is based on standard SAX (Simple Api for
Xml) but it follows a original approach based on the strong data
encapsulation paradigm of Object Oriented (OO)
programming.
The newest version!
/*-------------------------------------------------------------------------
Copyright 2006 Olivier Berlanger
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
-------------------------------------------------------------------------*/
package net.sf.xolite;
/**
* Interface of support object used to serialize XMLSerializable objects to XML.
* The XMLSerializable tells what to generate to this XMLSerializer using events similar to SAX events. So, this interface is
* similar to the org.xml.sax.ContentHandler interface. It's simply because this interface does similar job as SAX
* ContentHandler but just in the other direction (serialize from java objects to a stream). Fortunately, using the event-based
* SAX model is very easy when you are on the provider part. So, interacting with this XMLSerializer is pretty straightforward.
*
* You can also view this interface as a Visitor pattern specialized for XML. This object plays the "visitor" role while objects
* implementing the XMLSerializable
interface play the role of "element" and the "accept" method is called
* serialize
.
*
*/
public interface XMLSerializer {
/**
* Start an XML document.
*/
public void startDocument() throws XMLSerializeException;
/**
* Define a prefix mapping. This method associate the given prefix to the given namespace. So when a node of the given
* namespace will be serialized, the given prefix will be used to denote the namespace (and the prefix declaration will be
* inserted when necessary).
*
* This method should be called for each namespace URI before the first time they are used.
*
*
* If the given namespace is already associated to another prefix (or the same prefix), this method call is ignored (i.e.
* first association wins).
*
*
* If the given prefix is already associated to an other namespace, a new prefix is generated and mapped at the place of the
* given one.
*
*
* @param prefix
* a prefix (can be "" for default prefix mapping)
* @param namespaceUri
* a namespace URI
* @throws XMLSerializeException
* if prefix or namespaceUri is null or if required action cannot be done (implementation dependent).
*/
public void startPrefixMapping(String prefix, String namespaceUri) throws XMLSerializeException;
/**
* Start an XML element.
*
* @param namespaceUri
* The element namespace URI (can be null if namespace are not used)
* @param localName
* The element local name (a.k.a. NCName)
* @throws XMLSerializeException
* if required action cannot be done (implementation dependent).
*/
public void startElement(String namespaceUri, String localName) throws XMLSerializeException;
/**
* Add an attribute to the element that was just started. All the call to this method must immediately follow a
* startElement
call. attribute. This attribute use no namespace.
*
* @param localName
* The attribute local name (a.k.a. NCName)
* @param value
* the attribute value (null is treated as empty string).
* @throws XMLSerializeException
* if you don't call this method just after startElement
or if required action cannot be done
* (implementation dependent).
*/
public void attribute(String localName, String value) throws XMLSerializeException;
/**
* Add an attribute to the element that was just started. All the call to this method must immediately follow a
* startElement
call. attribute
*
* @param namespaceUri
* The attribute namespace URI. This URI is usually null because most of the time, attributes don't use a
* namespace.
* @param localName
* The attribute local name (a.k.a. NCName)
* @param value
* the attribute value (null is treated as empty string).
* @throws XMLSerializeException
* if you don't call this method just after startElement
or if required action cannot be done
* (implementation dependent).
*/
public void attribute(String namespaceUri, String localName, String value) throws XMLSerializeException;
/**
* End an XML element.
*
* @param namespaceUri
* The element namespace URI (can be null if namespace are not used)
* @param localName
* The element local name (a.k.a. NCName)
* @throws XMLSerializeException
* if required action cannot be done (implementation dependent).
*/
public void endElement(String namespaceUri, String localName) throws XMLSerializeException;
/**
* Add character content of an element. This method must be called between startElement
(possibly followed by
* attribute
) and endElement
. If this method is called several times for the same element, the
* texts are concatenated.
*
* @param text
* text of the currently started element.
* @throws XMLSerializeException
* if you don't call this method just beteween startElement
and endElement
or if
* required action cannot be done (implementation dependent).
*/
public void characters(String text) throws XMLSerializeException;
/**
* Same as characters but the text (supposed to be multi-line) is formatted to be an indented text block.
* The result will look like:
*
*
* <tag>
* some text
* spanning multiple
* lines.
* </tag>
*
*
* While usual output would have given:
*
*
* <tag>some text
* spanning multiple
* lines.</tag>
*
*
* The original text can be retrieved (when parsing) with the ElementText.getMultilineText(..)
method. This
* method will automatically remove the added space.
*
* @param text
* the text to serialize in XML
* @throws XMLSerializeException
* @see ElementText#getMultilineText(String, XMLEventParser)
*/
public void charactersMultiLine(String text) throws XMLSerializeException;
/**
* This is a helper method useful to add a simpleType element in one call. It is strictly equivalent to the following
* sequence:
*
*
* startElement(namespaceUri, localName);
* characters(text);
* endElement(namespaceUri, localName);
*
*
* @param namespaceUri
* The element namespace URI (can be null if namespace are not used)
* @param localName
* The element local name (a.k.a. NCName)
* @param text
* text of the element.
* @throws XMLSerializeException
*/
public void simpleElement(String namespaceUri, String localName, String text) throws XMLSerializeException;
/**
* End the current XML document.
*/
public void endDocument() throws XMLSerializeException;
/**
* Put a custom object in an internal Map.
* It can be retrieved later with getCustomObject(key)
method.
*
* @param key
* key of the custom object.
* @param value
* custom object.
*/
public void putCustomObject(Object key, Object value);
/**
* Get back any custom object that was put in with the putCustomObject(key, value)
method.
*
* @param key
* key of the custom object.
* @return the custom object.
*/
public Object getCustomObject(Object key);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy