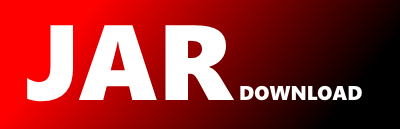
net.sf.xolite.impl.FlowXMLSerializer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of xo-lite Show documentation
Show all versions of xo-lite Show documentation
This project provides a lightweight framework to
serialize/deserialize (or marshall/unmarshall) java objects into
XML. The implementation is based on standard SAX (Simple Api for
Xml) but it follows a original approach based on the strong data
encapsulation paradigm of Object Oriented (OO)
programming.
The newest version!
/*-------------------------------------------------------------------------
Copyright 2006 Olivier Berlanger
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
-------------------------------------------------------------------------*/
package net.sf.xolite.impl;
import java.io.IOException;
import net.sf.xolite.XMLSerializeException;
/**
* A XMLSerializer
base implementation for all serializer writing the XML in a byte or character stream.
*
* @author Olivier Berlanger
* @see BaseXMLSerializer
*/
public abstract class FlowXMLSerializer extends BaseXMLSerializer {
private static final String TAB_STRING = "\t";
private int indentation = 4;
private boolean insertTabs = false;
private boolean insertLineBreaks = true;
private boolean breakFirstAttribute = false;
private boolean breakBetweenAttributes = false;
private boolean writeXmlHeader = true;
private String indentationString = "";
public void setFormatting(boolean useLineBreaks, boolean useTabs, int indentationSpaceCount) {
insertTabs = useTabs;
indentation = indentationSpaceCount;
insertLineBreaks = useLineBreaks;
}
public void setAttributeFormatting(boolean breakFirst, boolean breakOthers) {
breakFirstAttribute = breakFirst;
breakBetweenAttributes = breakOthers;
}
public int getIndentationSpaceCount() {
return indentation;
}
public boolean isUsingTabs() {
return insertTabs;
}
public boolean isUsingLineBreaks() {
return insertLineBreaks;
}
public boolean isBreakingForFirstAttribute() {
return breakFirstAttribute;
}
public boolean isBreakingBetweenAttributes() {
return breakBetweenAttributes;
}
public boolean getWriteXmlHeader() {
return writeXmlHeader;
}
public void setWriteXmlHeader(boolean newWriteXmlHeader) {
writeXmlHeader = newWriteXmlHeader;
}
// -------------------------------------- BaseXMLSerializer Implementation ---------------------------------------------
protected void startDocumentImpl() throws IOException {
if (writeXmlHeader) {
writeString("");
if (insertLineBreaks) writeNewLine();
}
}
protected void startElementImpl(String uri, String prefix, String tag) throws IOException, XMLSerializeException {
writeIndentation(getLevel());
writeString("<");
writePrefixedName(prefix, tag);
}
protected void attributeImpl(String uri, String prefix, String name, String value, int index) throws IOException {
boolean breakLine = ((index == 0) ? breakFirstAttribute : breakBetweenAttributes) && insertLineBreaks;
if (breakLine) writeAttributeBreak(breakFirstAttribute);
else writeString(" ");
writePrefixedName(prefix, name);
writeString("=\"");
writeDataCharacters(value);
writeString("\"");
}
protected void closeElementStartImpl(boolean complexContent) throws IOException {
writeString(">");
if (complexContent && insertLineBreaks) writeNewLine();
}
protected void charactersImpl(String text, boolean multiLine) throws IOException {
if (multiLine && insertLineBreaks) {
if (text != null) {
String[] lines = text.split("\n");
int nbrLines = lines.length;
for (int i = 0; i < nbrLines; i++) {
writeNewLine();
writeIndentation(getLevel() + 1);
writeDataCharacters(lines[i]);
}
}
} else {
writeDataCharacters(text);
}
}
protected void endInlineElementImpl(String uri, String prefix, String tag) throws Exception {
writeString("/>");
if (insertLineBreaks) writeNewLine();
}
protected void endTextElementImpl(String uri, String prefix, String tag, boolean multiLine) throws Exception {
if (multiLine && insertLineBreaks) {
writeNewLine();
writeIndentation(getLevel());
}
writeString("");
writePrefixedName(prefix, tag);
writeString(">");
if (insertLineBreaks) writeNewLine();
}
protected void endComplexElementImpl(String uri, String prefix, String tag) throws Exception {
writeIndentation(getLevel());
writeString("");
writePrefixedName(prefix, tag);
writeString(">");
if (insertLineBreaks) writeNewLine();
}
protected void writePrefixedName(String prefix, String tag) throws IOException {
if ((prefix != null) && (!prefix.equals(""))) {
writeString(prefix);
writeString(":");
}
writeString(tag);
}
protected void endDocumentImpl() throws Exception {
}
// ---------------------------------- implementation --------------------------------------------------
private String getIndentString() {
if (insertTabs) return TAB_STRING;
if (indentationString.length() == indentation) return indentationString;
StringBuffer sb = new StringBuffer();
for (int i = 0; i < indentation; i++)
sb.append(' ');
indentationString = sb.toString();
return indentationString;
}
protected void writeIndentation(int level) throws IOException {
if (insertLineBreaks && (insertTabs || (indentation > 0))) {
String str = getIndentString();
for (int i = 0; i < level; i++) {
writeString(str);
}
}
}
protected void writeAttributeBreak(boolean simpleIndent) throws IOException {
writeNewLine();
if (simpleIndent) {
writeIndentation(getLevel() + 2);
} else {
writeIndentation(getLevel());
int len = getLastElementLength() + 2;
for (int i = 0; i < len; i++)
writeCharacter(' ');
}
}
protected void writeDataCharacters(String text) throws IOException {
int len = (text == null) ? 0 : text.length();
char ch;
for (int i = 0; i < len; i++) {
ch = text.charAt(i);
switch (ch) {
case '&':
writeString("&");
break;
case '<':
writeString("<");
break;
case '>':
writeString(">");
break;
case '"':
writeString(""");
break;
case '\'':
writeString("'");
break;
default:
writeCharacter(ch);
}
}
}
// ---------------------------------- Methods to implements --------------------------------------------------
protected abstract void writeNewLine() throws IOException;
protected abstract void writeString(String text) throws IOException;
protected abstract void writeCharacter(char ch) throws IOException;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy