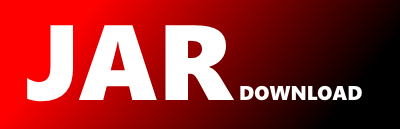
net.sf.xolite.utils.ClassObjectFactory Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of xo-lite Show documentation
Show all versions of xo-lite Show documentation
This project provides a lightweight framework to
serialize/deserialize (or marshall/unmarshall) java objects into
XML. The implementation is based on standard SAX (Simple Api for
Xml) but it follows a original approach based on the strong data
encapsulation paradigm of Object Oriented (OO)
programming.
The newest version!
/*-------------------------------------------------------------------------
Copyright 2006 Olivier Berlanger
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
-------------------------------------------------------------------------*/
package net.sf.xolite.utils;
import java.util.HashMap;
import java.util.Map;
import net.sf.xolite.XMLEventParser;
import net.sf.xolite.XMLObjectFactory;
import net.sf.xolite.XMLParseException;
import net.sf.xolite.XMLSerializable;
/**
* Simple implementation of XmlObjectFactory
based on class names.
* Using this factory implies that the objects constructed by the factory have public constructor with no parameters.
*/
public class ClassObjectFactory implements XMLObjectFactory {
private static final String NO_NAMESPACE = "@@null@@";
/** Map holding one sub-map per namespace, each sub-map contains mapping elementName-className. */
private Map> elementClasses = new HashMap>();
/**
* Add the definition of an element without namespace to this factory.
* The namespace information will be ignored by the factory for this element.
* Note: the given class must define a public constructor with no parameters and must implement the
* XMLSerializable
interface.
*
* @param localName
* the local name of the element.
* @param clazz
* the class of the object to be created.
*/
public void defineElement(String localName, Class> clazz) {
defineElement(null, localName, clazz);
}
/**
* Add the definition of an element without namespace to this factory.
* The namespace information will be ignored by the factory for this element.
* Note: the given class must define a public constructor with no parameters and must implement the
* XMLSerializable
interface.
*
* @param localName
* the local name of the element.
* @param className
* the fully qualified class name of the object to be created.
*/
public void defineElement(String localName, String className) {
defineElement(null, localName, className);
}
/**
* Add the definition of an element to this factory.
* Note: the given class must define a public constructor with no parameters and must implement the
* XMLSerializable
interface.
*
* @param namespaceUri
* the element namespace URI
* @param localName
* the local name of the element.
* @param clazz
* the class of the object to be created.
*/
public void defineElement(String namespaceUri, String localName, Class> clazz) {
if (!XMLSerializable.class.isAssignableFrom(clazz)) {
throw new IllegalArgumentException("The " + clazz + " must implement XMLSerializable interface");
}
defineElement(namespaceUri, localName, clazz.getName());
}
/**
* Add the definition of an element to this factory.
* Note: the given class must define a public constructor with no parameters and must implement the
* XMLSerializable
interface.
*
* @param namespaceUri
* the element namespace URI
* @param localName
* the local name of the element.
* @param className
* the fully qualified class name of the object to be created.
*/
public void defineElement(String namespaceUri, String localName, String className) {
String namespaceKey = isEmpty(namespaceUri) ? NO_NAMESPACE : namespaceUri;
Map namespaceClasses = elementClasses.get(namespaceKey);
if (namespaceClasses == null) {
namespaceClasses = new HashMap();
elementClasses.put(namespaceKey, namespaceClasses);
}
namespaceClasses.put(localName, className);
}
/**
* Create an object corresponding to the XML element of the given name.
*/
public XMLSerializable createObject(String namespaceUri, String localName, XMLEventParser parser) throws XMLParseException {
XMLSerializable result = null;
String className = null;
if (!isEmpty(namespaceUri)) {
className = getClassName(namespaceUri, localName);
}
if (className == null) {
className = getClassName(NO_NAMESPACE, localName);
}
if (className == null) {
throwException("Unknown element <", namespaceUri, localName, "> in ClassObjectFactory", parser, null);
}
try {
result = (XMLSerializable) Class.forName(className).newInstance();
} catch (ClassCastException cce) {
throwException("Element <", namespaceUri, localName, "> = " + className
+ " does not implement the XMLSerializable interface", parser, null);
} catch (Exception e) {
throwException("The element <", namespaceUri, localName, "> = " + className
+ " cannot be instantiated by ClassObjectFactory", parser, e);
}
return result;
}
private String getClassName(String namespaceUri, String localName) {
Map namspaceClasses = elementClasses.get(namespaceUri);
return (namspaceClasses == null) ? null : (String) namspaceClasses.get(localName);
}
private boolean isEmpty(String str) {
if (str == null) return true;
return str.trim().equals("");
}
private void throwException(String messagePrefix, String namespaceUri, String localName, String messagePostfix,
XMLEventParser parser, Exception cause) throws XMLParseException {
StringBuilder sb = new StringBuilder(messagePrefix);
if (!isEmpty(namespaceUri)) {
sb.append(namespaceUri);
sb.append(":");
}
sb.append(localName);
sb.append(messagePostfix);
String message = sb.toString();
if (parser != null) parser.throwParseException(message, cause);
else throw new XMLParseException(message, cause);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy