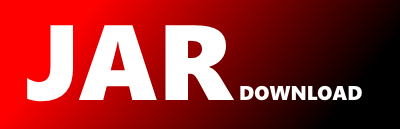
net.sf.xolite.utils.XMLSerializationTester Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of xo-lite Show documentation
Show all versions of xo-lite Show documentation
This project provides a lightweight framework to
serialize/deserialize (or marshall/unmarshall) java objects into
XML. The implementation is based on standard SAX (Simple Api for
Xml) but it follows a original approach based on the strong data
encapsulation paradigm of Object Oriented (OO)
programming.
The newest version!
/*-------------------------------------------------------------------------
Copyright 2012 Olivier Berlanger
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
-------------------------------------------------------------------------*/
package net.sf.xolite.utils;
import java.io.ByteArrayInputStream;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.UnsupportedEncodingException;
import net.sf.xolite.XMLObjectFactory;
import net.sf.xolite.XMLSerializable;
import net.sf.xolite.dom.DomUtils;
import net.sf.xolite.dom.DomXMLEventParser;
import net.sf.xolite.dom.DomXMLSerializer;
import net.sf.xolite.sax.SaxXMLEventParser;
import org.w3c.dom.Document;
import org.xml.sax.InputSource;
/**
* Helper class to test your XML serialization.
* Give it an input stream containing a serialized XML and a XMLSerializable representing the same XML. When you call
* testXMLSerialization()
, this tester class will try several transformations (using X-O lite APIs and standard DOM
* APIs) from both sources and check that the result are matching.
*
* Note: for the purpose of the test this class will create several copies of the initial document in memory (as objects, DOM
* and byte[]). So it's not suited for very big documents.
*
*
* @author Olivier Berlanger
*/
public class XMLSerializationTester {
private byte[] xmlSource;
private XMLSerializable root;
private String schemaLocations;
private XMLObjectFactory factory;
private int comparisonFlags;
public XMLSerializationTester() {
}
public XMLSerializationTester(InputStream xmlSourceStream, XMLSerializable rootObject) {
setXmlSource(xmlSourceStream);
setRootObject(rootObject);
}
public void setRootObject(XMLSerializable rootObject) {
root = rootObject;
}
public void setXmlSource(InputStream xmlSourceStream) {
try {
ByteArrayOutputStream baos = new ByteArrayOutputStream();
byte[] buffer = new byte[1024];
int readCount;
while ((readCount = xmlSourceStream.read(buffer)) > 0) {
baos.write(buffer, 0, readCount);
}
xmlSourceStream.close();
baos.close();
xmlSource = baos.toByteArray();
} catch (IOException ioe) {
throw new IllegalArgumentException("Unable to read xml source stream", ioe);
}
}
public void setXmlSource(String xmlSourceString) {
setXmlSource(xmlSourceString, "UTF-8");
}
public void setXmlSource(String xmlSourceString, String encoding) {
try {
xmlSource = xmlSourceString.getBytes(encoding);
} catch (UnsupportedEncodingException ex) {
throw new IllegalArgumentException("Unsupported encoding", ex);
}
}
public void setSchemaLocations(String newSchemaLocations) {
schemaLocations = newSchemaLocations;
}
public void setXMLObjectFactory(XMLObjectFactory newFactory) {
factory = newFactory;
}
public void setComparisonFlags(int newComparisonFlags) {
comparisonFlags = newComparisonFlags;
}
public void testXMLSerialization() throws Exception {
// get a DOM document for comparison
Document referenceDomDocument = DomUtils.streamToDom(new ByteArrayInputStream(xmlSource));
checkWithSaxRoundtrip(referenceDomDocument);
checkWithDomRoundtrip(referenceDomDocument);
}
private void checkWithSaxRoundtrip(Document referenceDomDocument) throws Exception {
// parse the XML source using SAX
if (root == null) root = new RootHolder();
InputSource src = new InputSource(new ByteArrayInputStream(xmlSource));
SaxXMLEventParser saxParser;
if (schemaLocations == null) saxParser = new SaxXMLEventParser();
else saxParser = new SaxXMLEventParser(schemaLocations);
if (factory != null) saxParser.setFactory(factory);
saxParser.parse(src, root);
// serialize using StreamXMLSerializer
ByteArrayOutputStream baos = new ByteArrayOutputStream();
StreamXMLSerializer streamSerializer = new StreamXMLSerializer(baos);
streamSerializer.serializeObject(root);
baos.close();
byte[] saxRoundtripResult = baos.toByteArray();
// transform the result to DOM and compare with the reference DOM
Document saxRoundtripDocument = DomUtils.streamToDom(new ByteArrayInputStream(saxRoundtripResult));
DomUtils.documentsAreEquals(saxRoundtripDocument, referenceDomDocument, comparisonFlags);
}
private void checkWithDomRoundtrip(Document referenceDomDocument) throws Exception {
// parse the referenceDomDocument to object
if (root == null) root = new RootHolder();
DomXMLEventParser domParser = new DomXMLEventParser();
if (factory != null) domParser.setFactory(factory);
domParser.parse(referenceDomDocument, root);
// serialize back to DOM using DomXMLSerializer
DomXMLSerializer serializer = new DomXMLSerializer();
Document domRoundtripDocument = serializer.serializeToDOM(root);
// compare the two DOMs
DomUtils.documentsAreEquals(domRoundtripDocument, referenceDomDocument, comparisonFlags);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy