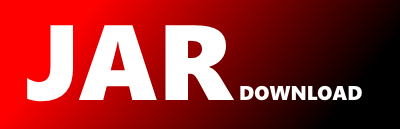
it.xsemantics.runtime.TraceUtils Maven / Gradle / Ivy
package it.xsemantics.runtime;
import com.google.common.base.Objects;
import com.google.common.collect.Lists;
import it.xsemantics.runtime.ErrorInformation;
import it.xsemantics.runtime.RuleApplicationTrace;
import it.xsemantics.runtime.RuleFailedException;
import java.util.LinkedList;
import java.util.List;
import org.eclipse.emf.ecore.EObject;
import org.eclipse.emf.ecore.EStructuralFeature;
import org.eclipse.xtext.nodemodel.ICompositeNode;
import org.eclipse.xtext.nodemodel.util.NodeModelUtils;
import org.eclipse.xtext.util.Strings;
import org.eclipse.xtext.xbase.lib.CollectionLiterals;
import org.eclipse.xtext.xbase.lib.Functions.Function1;
import org.eclipse.xtext.xbase.lib.IterableExtensions;
import org.eclipse.xtext.xbase.lib.ListExtensions;
/**
* Several utility methods that act on rule traces, rule failures, etc.
*
* @author Lorenzo Bettini - Initial contribution and API
* @since 1.6
*/
@SuppressWarnings("all")
public class TraceUtils {
public LinkedList failureAsList(final RuleFailedException e) {
LinkedList _xblockexpression = null;
{
final LinkedList list = CollectionLiterals.newLinkedList(e);
RuleFailedException ex = e.previous;
while ((!Objects.equal(ex, null))) {
{
String _message = ex.getMessage();
boolean _notEquals = (!Objects.equal(_message, null));
if (_notEquals) {
list.add(ex);
}
ex = ex.previous;
}
}
_xblockexpression = list;
}
return _xblockexpression;
}
public List failureTraceAsStrings(final RuleFailedException e) {
List _xblockexpression = null;
{
final StringBuffer indent = new StringBuffer("");
LinkedList _failureAsList = this.failureAsList(e);
final Function1 _function = new Function1() {
@Override
public String apply(final RuleFailedException it) {
String _xblockexpression = null;
{
String _string = indent.toString();
String _message = it.getMessage();
String _removeIndentation = TraceUtils.this.removeIndentation(_message);
final String listElem = (_string + _removeIndentation);
indent.append(" ");
_xblockexpression = listElem;
}
return _xblockexpression;
}
};
_xblockexpression = ListExtensions.map(_failureAsList, _function);
}
return _xblockexpression;
}
public String failureTraceAsString(final RuleFailedException e) {
List _failureTraceAsStrings = this.failureTraceAsStrings(e);
return IterableExtensions.join(_failureTraceAsStrings, "\n");
}
public LinkedList traceAsStrings(final RuleApplicationTrace ruleTrace) {
LinkedList _xblockexpression = null;
{
final LinkedList result = new LinkedList();
for (final Object e : ruleTrace.trace) {
this.buildTrace(result, e, 0);
}
_xblockexpression = result;
}
return _xblockexpression;
}
public void buildTrace(final List trace, final Object element, final int inc) {
if ((element instanceof RuleApplicationTrace)) {
for (final Object e : ((RuleApplicationTrace)element).trace) {
this.buildTrace(trace, e, (inc + 1));
}
} else {
String _increment = this.increment(inc);
String _string = element.toString();
String _removeIndentation = this.removeIndentation(_string);
String _plus = (_increment + _removeIndentation);
trace.add(_plus);
}
}
public String traceAsString(final RuleApplicationTrace ruleTrace) {
LinkedList _traceAsStrings = this.traceAsStrings(ruleTrace);
return IterableExtensions.join(_traceAsStrings, "\n");
}
public String increment(final int inc) {
String _xblockexpression = null;
{
StringBuffer buffer = new StringBuffer();
int i = 0;
while ((i < inc)) {
{
buffer.append(" ");
i = (i + 1);
}
}
_xblockexpression = buffer.toString();
}
return _xblockexpression;
}
public LinkedList allErrorInformation(final RuleFailedException e) {
LinkedList _xblockexpression = null;
{
final LinkedList list = Lists.newLinkedList(e.errorInformations);
RuleFailedException ex = e.previous;
while ((!Objects.equal(ex, null))) {
{
list.addAll(ex.errorInformations);
ex = ex.previous;
}
}
_xblockexpression = list;
}
return _xblockexpression;
}
public List removeDuplicateErrorInformation(final Iterable errorInformations) {
List _xblockexpression = null;
{
final List noDuplicates = new LinkedList();
for (final ErrorInformation errorInformation : errorInformations) {
final Function1 _function = new Function1() {
@Override
public Boolean apply(final ErrorInformation it) {
boolean _and = false;
boolean _and_1 = false;
EObject _source = it.getSource();
EObject _source_1 = errorInformation.getSource();
boolean _equals = Objects.equal(_source, _source_1);
if (!_equals) {
_and_1 = false;
} else {
EStructuralFeature _feature = it.getFeature();
EStructuralFeature _feature_1 = errorInformation.getFeature();
boolean _equals_1 = Objects.equal(_feature, _feature_1);
_and_1 = _equals_1;
}
if (!_and_1) {
_and = false;
} else {
Object _data = it.getData();
Object _data_1 = errorInformation.getData();
boolean _equals_2 = Objects.equal(_data, _data_1);
_and = _equals_2;
}
return Boolean.valueOf(_and);
}
};
boolean _exists = IterableExtensions.exists(noDuplicates, _function);
boolean _not = (!_exists);
if (_not) {
noDuplicates.add(errorInformation);
}
}
_xblockexpression = noDuplicates;
}
return _xblockexpression;
}
public LinkedList removeNonNodeModelSources(final Iterable errorInformations) {
final Function1 _function = new Function1() {
@Override
public Boolean apply(final ErrorInformation it) {
EObject _source = it.getSource();
ICompositeNode _node = NodeModelUtils.getNode(_source);
return Boolean.valueOf((!Objects.equal(_node, null)));
}
};
Iterable _filter = IterableExtensions.filter(errorInformations, _function);
return Lists.newLinkedList(_filter);
}
public LinkedList filteredErrorInformation(final RuleFailedException e) {
LinkedList _allErrorInformation = this.allErrorInformation(e);
List _removeDuplicateErrorInformation = this.removeDuplicateErrorInformation(_allErrorInformation);
return this.removeNonNodeModelSources(_removeDuplicateErrorInformation);
}
public RuleFailedException innermostRuleFailedExceptionWithNodeModelSources(final RuleFailedException e) {
LinkedList _failureAsList = this.failureAsList(e);
final Function1 _function = new Function1() {
@Override
public Boolean apply(final RuleFailedException it) {
LinkedList _filteredErrorInformation = TraceUtils.this.filteredErrorInformation(it);
boolean _isEmpty = _filteredErrorInformation.isEmpty();
return Boolean.valueOf((!_isEmpty));
}
};
return IterableExtensions.findLast(_failureAsList, _function);
}
public String removeIndentation(final String s) {
return Strings.removeLeadingWhitespace(s);
}
/**
* Returns the last element in the trace that is not a RuleApplicationTrace
*/
public Object lastElementNotTrace(final RuleApplicationTrace trace) {
final Function1
© 2015 - 2025 Weber Informatics LLC | Privacy Policy