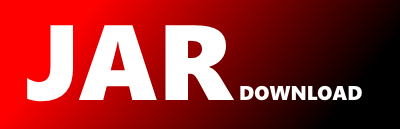
jbase.controlflow.JbaseBranchingStatementDetector Maven / Gradle / Ivy
package jbase.controlflow;
import java.util.Arrays;
import jbase.jbase.XJBranchingStatement;
import jbase.jbase.XJSemicolonStatement;
import org.eclipse.xtext.xbase.XBlockExpression;
import org.eclipse.xtext.xbase.XExpression;
import org.eclipse.xtext.xbase.XIfExpression;
import org.eclipse.xtext.xbase.lib.Functions.Function1;
import org.eclipse.xtext.xbase.lib.IterableExtensions;
/**
* Whether in the passed expression a branching statement like break
* and continue is surely executed.
*
* @author Lorenzo Bettini
*/
@SuppressWarnings("all")
public class JbaseBranchingStatementDetector {
public boolean isSureBranchStatement(final XExpression e) {
if ((e == null)) {
return false;
}
return this.sureBranch(e);
}
protected boolean _sureBranch(final XExpression e) {
return false;
}
protected boolean _sureBranch(final XJBranchingStatement e) {
return true;
}
protected boolean _sureBranch(final XIfExpression e) {
return (this.isSureBranchStatement(e.getThen()) && ((e.getElse() == null) || this.isSureBranchStatement(e.getElse())));
}
protected boolean _sureBranch(final XBlockExpression e) {
final Function1 _function = new Function1() {
@Override
public Boolean apply(final XExpression it) {
return Boolean.valueOf(JbaseBranchingStatementDetector.this.isSureBranchStatement(it));
}
};
return IterableExtensions.exists(e.getExpressions(), _function);
}
protected boolean _sureBranch(final XJSemicolonStatement e) {
return this.isSureBranchStatement(e.getExpression());
}
protected boolean sureBranch(final XExpression e) {
if (e instanceof XJBranchingStatement) {
return _sureBranch((XJBranchingStatement)e);
} else if (e instanceof XJSemicolonStatement) {
return _sureBranch((XJSemicolonStatement)e);
} else if (e instanceof XBlockExpression) {
return _sureBranch((XBlockExpression)e);
} else if (e instanceof XIfExpression) {
return _sureBranch((XIfExpression)e);
} else if (e != null) {
return _sureBranch(e);
} else {
throw new IllegalArgumentException("Unhandled parameter types: " +
Arrays.
© 2015 - 2025 Weber Informatics LLC | Privacy Policy