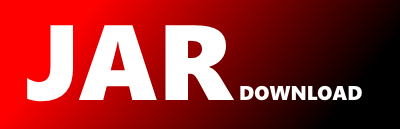
net.sf.statscm.SrcManager Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of stat-scm Show documentation
Show all versions of stat-scm Show documentation
Maven 2 Mojo Plugin that generates Source Code Management
Statistics reports as part of the mvn site command.
package net.sf.statscm;
/*
* Copyright 2006 Doug Culnane
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
import java.io.BufferedReader;
import java.io.DataInputStream;
import java.io.EOFException;
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import org.apache.maven.plugin.logging.Log;
/**
* Source Code Management Object, provides access to SCM system.
*
*
* @author DoCulnan
*
*/
public class SrcManager
{
/**
* Number of milisconds to sleep between polls of threads for state information.
*/
private static final int MILLISECONDS_BETEEW_THREAD_POLL = 200;
/**
* Get the log file.
*
* @todo Get the scm log in a more elgant way than with the command line.
* @param baseDir Base directory.
* @param log logger for writing user feedback.
* @return Success.
*/
public boolean log( File baseDir, Log log )
{
String[] commad;
if ( StatConf.isStatSVN() )
{
commad = new String[] { "svn", "log", baseDir.getAbsolutePath(), "-v", "--xml" };
}
else if ( StatConf.isStatCVS() )
{
commad = new String[] { "cvs", "log" };
}
else
{
// Give up if not supported.
log.warn( "Can not get log file for SCM Repository Type." );
return false;
}
File file = new File( StatConf.getLogFileName() );
try
{
log.info( "scm log > " + file.getAbsolutePath() );
Runtime rt = Runtime.getRuntime();
Process proc = rt.exec( commad );
ConsoleErrorStream errorGobbler = new ConsoleErrorStream( proc.getErrorStream(), log );
ConsoleFileStream logStream = new ConsoleFileStream( proc.getInputStream(), file, log );
errorGobbler.start();
logStream.start();
while ( errorGobbler.isAlive() || logStream.isAlive() )
{
Thread.sleep( MILLISECONDS_BETEEW_THREAD_POLL );
}
log.info( "scm log ...; exitValue: " + proc.waitFor() );
}
catch ( IOException e )
{
log.error( "Error Getting SCM log.", e );
}
catch ( InterruptedException e )
{
log.error( "Error Getting SCM log.", e );
}
catch ( Exception e )
{
log.error( "Error Getting SCM log.", e );
}
return true;
}
}
/**
* Thread class for collecting Console error output and logging it at WARN level.
*
* @author DoCulnan
*
*/
class ConsoleErrorStream extends Thread
{
/**
* Input Stream to read.
*/
private InputStream is;
/**
* Logger for user feedback.
*/
private Log log;
/**
* Create an thread ready to run.
*
* @param inputStream InputStream to read.
* @param logger Logger for user feedback.
*/
ConsoleErrorStream( InputStream inputStream, Log logger )
{
this.is = inputStream;
this.log = logger;
}
/**
* Run the thread until the InputStream reaches its end.
*/
public void run()
{
try
{
InputStreamReader isr = new InputStreamReader( is );
BufferedReader br = new BufferedReader( isr );
String line = null;
while ( ( line = br.readLine() ) != null )
{
log.warn( line );
}
}
catch ( IOException ioe )
{
log.error( ioe.getMessage(), ioe );
}
}
}
/**
* Thread class for collecting console output and writing it to a file.
*
* @author DoCulnan
*
*/
class ConsoleFileStream extends Thread
{
/**
* Logger for user feedback.
*/
private Log log;
/**
* File to write stream to.
*/
private File file;
/**
* Input Stream to read.
*/
private InputStream is;
/**
* Create an thread ready to run.
*
* @param inputStream InputStream to read.
* @param outputFile File to write to.
* @param logger Logger for user feedback.
*/
ConsoleFileStream( InputStream inputStream, File outputFile, Log logger )
{
this.is = inputStream;
this.file = outputFile;
this.log = logger;
}
/**
* Run the thread until the InputStream reaches its end.
*/
public void run()
{
FileOutputStream fos = null;
try
{
DataInputStream dis = new DataInputStream( is );
fos = new FileOutputStream( file, false );
while ( true )
{
fos.write( dis.readByte() );
}
}
catch ( EOFException eofe )
{
log.info( file.toString() + " EOF." );
}
catch ( IOException ioe )
{
log.error( ioe.getMessage(), ioe );
}
finally
{
try
{
fos.close();
}
catch ( IOException e )
{
log.error( e.getMessage(), e );
fos = null;
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy