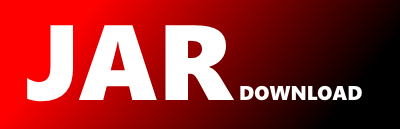
net.sf.statscm.StatConf Maven / Gradle / Ivy
package net.sf.statscm;
/*
* Copyright 2006 Doug Culnane
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
import java.io.File;
import java.util.Locale;
import net.sf.statcvs.output.ConfigurationException;
import net.sf.statcvs.output.ConfigurationOptions;
import org.apache.maven.model.Developer;
import org.apache.maven.project.MavenProject;
/**
* Central StatSCM Configuration object.
*
*
* @author DoCulnan
*
*/
public class StatConf extends ConfigurationOptions
{
/**
* Name of directory for HTML and xdoc files.
*/
protected static final String STATSCM_DIR_NAME = "statscm";
/**
* String identifier for Subversion connetion type
*/
protected static final String CONNECTION_TYPE_SVN = "svn";
/**
* String identifier for CVS connetion type
*/
protected static final String CONNECTION_TYPE_CVS = "cvs";
/**
* Conveniance variable to deal with the Unix (/) DOS (\) problem. This is a shortcut for
* System.getProperty("file.separator").
*/
protected String FILE_SEPARATOR = System.getProperty( "file.separator" );
/**
* Variable indicating the type of SCM system.
*/
private String connectionType;
/**
* Store the locale for this confuguration instance.
*/
private Locale locale = Locale.US;
/**
* Public constructor.
*/
StatConf()
{
}
/**
* Base Directory file. This is the root folder of the project.
*/
private File baseDirectory = new File( "." );
/**
* To be called by plugin. If this is not called early in the process then nothing will work.
*
* @param project
* Maven Project Object Model (pom.xml).
* @throws ConfigurationException
* Throws error if there is any problem with the configuration.
*/
public void configure( MavenProject project, Locale locale ) throws ConfigurationException
{
this.locale = locale;
if ( project.getBasedir() != null )
{
baseDirectory = project.getBasedir();
}
if ( project.getScm() == null )
{
throw new ConfigurationException( "There must be scm section in your pom.xml file." );
}
// Set output parameters.
setOutputFormat( "xdoc" );
// ? setDefaultCssFile("maven2-xdoc.css");
setOutputDir( baseDirectory.getAbsolutePath() + FILE_SEPARATOR + "target" + FILE_SEPARATOR + "generated-site"
+ FILE_SEPARATOR + "xdoc" + FILE_SEPARATOR + STATSCM_DIR_NAME );
// Set up connection type
connectionType = null;
if ( project.getScm().getConnection() != null )
{
connectionType = extractConnectionType( project.getScm().getConnection() );
}
if ( connectionType == null )
{
connectionType = extractConnectionType( project.getScm().getDeveloperConnection() );
}
if ( connectionType == null )
{
throw new ConfigurationException( "There must be scm connection url section in your pom.xml file for a supported SCM type." );
}
// Integration With SCM Browser
// TODO need to make up some better rules to try and get the type if SCM Browser server application.
if ( project.getScm().getUrl() != null )
{
if ( project.getScm().getUrl().toLowerCase( Locale.getDefault() ).indexOf( "viewvc" ) > -1 )
{
setViewVcURL( project.getScm().getUrl() );
}
else if ( project.getScm().getUrl().toLowerCase( Locale.getDefault() ).indexOf( "viewcvs" ) > -1 )
{
setViewCvsURL("");
}
else if ( project.getScm().getUrl().toLowerCase( Locale.getDefault() ).indexOf( "jcvs" ) > -1 )
{
setJCVSWebURL("");
}
else if ( project.getScm().getUrl().toLowerCase( Locale.getDefault() ).indexOf( "chora" ) > -1 )
{
setChoraURL("");
}
}
// Configure Issue Management.
if ( project.getIssueManagement() != null )
{
if ( project.getIssueManagement().getSystem() != null )
{
String bugSystem = project.getIssueManagement().getSystem().toLowerCase( Locale.getDefault() );
if ( "bugzilla".equals( bugSystem ) )
{
setBugzillaUrl( project.getIssueManagement().getUrl() );
}
else if ( "mantis".equals( bugSystem ) )
{
setMantisUrl( project.getIssueManagement().getUrl() );
}
else if ( "trac".equals( bugSystem ))
{
// Can only do this when we can add bug trackers and repos to stat-svn
// NOTE: Need to account for the IssueManagement url not being the exact
// base path where the trac wiki starts. Maybe add a mojo parameter that
// either gives the alternate path or an offset to the supplied path.
// Trac trac = new Trac( project.getIssueManagement().getUrl() );
// ConfigurationOptions.webBugTracker = trac;
// ConfigurationOptions.webRepository = trac;
}
}
}
// General Info.
if ( project.getName() != null )
{
setProjectName( project.getName() );
}
// set developer properties.
if (project.getDevelopers() != null) {
java.util.List developers = project.getDevelopers();
for ( java.util.Iterator i = developers.iterator(); i.hasNext(); )
{
Developer developer = (Developer) i.next();
if (developer.getName() != null)
{
System.setProperty("user." + developer.getId() + ".realName", developer.getName());
}
if (developer.getUrl() != null)
{
System.setProperty("user." + developer.getId() + ".url", developer.getUrl() );
}
if (developer.getEmail() != null)
{
System.setProperty("user." + developer.getId() + ".email", developer.getEmail());
}
}
}
//ConfigurationOptions.setSymbolicNamesPattern(".*");
}
/**
* Returns the type of SCM system.
*
* @return String represeting an ID for type of connection.
*/
protected String getConnectionType()
{
return connectionType;
}
/**
* Is the Maven Project using SVN as a SCM system?
*
* @return true if SVN
*/
public boolean isStatSVN()
{
return CONNECTION_TYPE_SVN.equals( getConnectionType() );
}
/**
* Is the Maven Project using CVS as a SCM system?
*
* @return true if CVS
*/
public boolean isStatCVS()
{
return CONNECTION_TYPE_CVS.equals( getConnectionType() );
}
/**
* Try and extract the connection type for the url from the the Maven SCM Connection URL.
*
* @param connectionUrl
* Maven SCM Connection URL.
* @return String represeting an ID for type of connection or null if can not be extracted.
*/
protected static String extractConnectionType( String connectionUrl )
{
if ( connectionUrl == null )
{
return null;
}
else
{
String con = connectionUrl.toLowerCase( Locale.getDefault() );
String[] scmTypes = { CONNECTION_TYPE_SVN, CONNECTION_TYPE_CVS };
for ( int i = 0; i < scmTypes.length; i++ )
{
if ( con.indexOf( scmTypes[i] + ":" ) > -1 )
{
return scmTypes[i];
}
}
return null;
}
}
/**
* Method to access the Absolute location of the log file.
*
* @return Absolute path of log file.
*/
public static String getLogFileName()
{
return getOutputDir() + "scm.log";
}
/**
* Getter for the Base Directory.
*
* @return root folder for project.
*/
public File getBaseDirectory()
{
return baseDirectory;
}
public Locale getLocale()
{
return locale;
}
public void setLocale( Locale locale )
{
this.locale = locale;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy