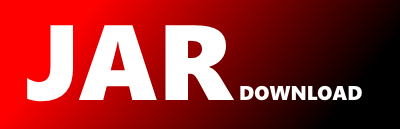
net.sf.statscm.Trac Maven / Gradle / Ivy
package net.sf.statscm;
import java.util.HashSet;
import java.util.Set;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
import net.sf.statcvs.Messages;
import net.sf.statcvs.model.Directory;
import net.sf.statcvs.model.Revision;
import net.sf.statcvs.model.VersionedFile;
import net.sf.statcvs.output.WebRepositoryIntegration;
import net.sf.statcvs.pages.HTML;
import net.sf.statcvs.pages.ReportSuiteMaker;
import net.sf.statcvs.weblinks.bugs.BugTracker;
public class Trac extends BugTracker implements WebRepositoryIntegration
{
protected static Pattern bugRegex = Pattern.compile( "(?:#\\s*)?(\\d+)" );
private Set atticFileNames = new HashSet();
public Trac(final String baseURL)
{
super( baseURL );
}
public String getName()
{
return "Trac";
}
public String bugURL( String bugNumber )
{
return baseURL() + "ticket/" + bugNumber;
}
/**
* Filters a String, e.g. a commit message, replacing bug references with links to the tracker.
*
* @param plainTextInput String to examine for bug references
* @return A copy of input
, with bug references replaced with HTML links
*/
public String toHTMLWithLinks( String plainTextInput )
{
if ( baseURL() == null || baseURL().length() == 0 )
{
return HTML.escape( plainTextInput );
}
StringBuffer result = new StringBuffer();
final Matcher m = bugRegex.matcher( plainTextInput );
int offset = 0;
while ( m.find() )
{
String linkLabel = m.group();
String bugNumber = m.group( 1 );
String bugURL = bugURL( bugNumber );
result.append( HTML.escape( plainTextInput.substring( offset, m.start() ) ) );
if ( bugURL == null )
{
result.append( HTML.escape( linkLabel ) );
}
else
{
result.append( HTML.getLink( bugURL, linkLabel, HTML.getIcon( ReportSuiteMaker.BUG_ICON, Messages
.getString( "BUG_ICON" ) ), "" ) );
}
offset = m.end();
}
result.append( HTML.escape( plainTextInput.substring( offset, plainTextInput.length() ) ) );
return result.toString();
}
protected String getFileUrl( VersionedFile file )
{
String filename;
if ( isInAttic( file ) )
{
String path = file.getDirectory().getPath();
filename = "/" + path + "Attic/" + file.getFilename();
}
else
{
filename = "/" + file.getFilenameWithPath();
}
return filename;
}
public String getDiffUrl( Revision oldRevision, Revision newRevision )
{
return baseURL() + //
"/changeset/" + oldRevision.getRevisionNumber() + "/" + //
oldRevision.getFile() + //
"?old=" + newRevision.getRevisionNumber() + //
"&oldPath=" + newRevision.getFile();
}
public String getDirectoryUrl( Directory directory )
{
return baseURL() + "/browser" + directory.getPath();
}
public String getFileHistoryUrl( VersionedFile file )
{
return baseURL() + "log/" + getFileUrl( file );
}
public String getFileViewUrl( VersionedFile file )
{
return baseURL() + getFileUrl( file );
}
public String getFileViewUrl( Revision revision )
{
return baseURL() + getFileUrl( revision.getFile() ) + "?rev=" + revision.getRevisionNumber();
}
public void setAtticFileNames( Set atticFileNames )
{
this.atticFileNames = atticFileNames;
}
protected boolean isInAttic( VersionedFile file )
{
return atticFileNames.contains( file.getFilenameWithPath() );
}
public String getBaseUrl() {
return super.baseURL();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy