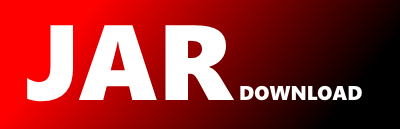
net.smartlab.web.AbstractArchiveAction Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of smartweb Show documentation
Show all versions of smartweb Show documentation
SmartWeb is a web application development meta framework based on Jakarta Struts, Hibernate and other open source frameworks and libraries.
/*
* The SmartWeb Framework
* Copyright (C) 2004-2006
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA
*
* For further informations on the SmartWeb Framework please visit
*
* http://smartweb.sourceforge.net
*/
package net.smartlab.web;
import java.beans.Introspector;
import java.beans.PropertyDescriptor;
import java.lang.reflect.Method;
import java.util.ArrayList;
import java.util.List;
import javax.servlet.ServletRequest;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import net.smartlab.web.DataAccessObject.SearchInfo;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import org.apache.struts.action.ActionForm;
import org.apache.struts.action.ActionMapping;
import org.apache.struts.action.DynaActionForm;
import org.apache.struts.util.RequestUtils;
/**
* This is the base action for generic archive management. The class provides
* template methods for common archive management operations and provides
* support methods to handle common problems like multiple selections and search
* criteria.
*
* @author rlogiacco
* @uml.dependency supplier="net.smartlab.web.EmptyMarkerTag"
* @uml.dependency supplier="net.smartlab.web.Validator"
*/
public abstract class AbstractArchiveAction extends DynaAction {
private final static Log LOGGER = LogFactory.getLog(AbstractArchiveAction.class);
/**
* The standard name for a multiple selection request parameter.
*/
public final static String SELECTION = "selection";
/**
* This action method should be invoked to list items matching a set of
* specified criteria.
*
* @param form the html form submitted with this request.
* @param request the user request.
* @param response the representation of the response channel.
* @param mapping the system control mapping.
* @return the name of a defined global or local forward.
* @throws Exception if something unexpected happend during the request
* execution.
*/
public abstract String search(ActionForm form, HttpServletRequest request, HttpServletResponse response,
ActionMapping mapping) throws Exception;
/**
* This action method should be invoked to retrieve a specific item.
*
* @param form the html form submitted with this request.
* @param request the user request.
* @param response the representation of the response channel.
* @param mapping the system control mapping.
* @return the name of a defined global or local forward.
* @throws Exception if something unexpected happend during the request
* execution.
*/
public abstract String select(ActionForm form, HttpServletRequest request, HttpServletResponse response,
ActionMapping mapping) throws Exception;
/**
* This action method should be invoked to save or update an item.
*
* @param form the html form submitted with this request.
* @param request the user request.
* @param response the representation of the response channel.
* @param mapping the system control mapping.
* @return the name of a defined global or local forward.
* @throws Exception if something unexpected happend during the request
* execution.
*/
public abstract String update(ActionForm form, HttpServletRequest request, HttpServletResponse response,
ActionMapping mapping) throws Exception;
/**
* This action method should be invoked to permanently remove an item.
*
* @param form the html form submitted with this request.
* @param request the user request.
* @param response the representation of the response channel.
* @param mapping the system control mapping.
* @return the name of a defined global or local forward.
* @throws Exception if something unexpected happend during the request
* execution.
*/
public abstract String remove(ActionForm form, HttpServletRequest request, HttpServletResponse response,
ActionMapping mapping) throws Exception;
/**
* This action method should be invoked to permanently remove a set of
* items.
*
* @param form the html form submitted with this request.
* @param request the user request.
* @param response the representation of the response channel.
* @param mapping the system control mapping.
* @return the name of a defined global or local forward.
* @throws Exception if something unexpected happend during the request
* execution.
*/
public abstract String removeAll(ActionForm form, HttpServletRequest request, HttpServletResponse response,
ActionMapping mapping) throws Exception;
/**
* TODO documentation
*
* @param request the user request.
* @return
*/
public static String[] getListSelection(ServletRequest request) {
return request.getParameterValues("selection");
}
/**
* TODO documentation
*
* @param request the user request.
* @param form
* @param name
* @return
*/
public static String[] getSelection(ActionForm form, String name) {
String[] values = null;
if (form instanceof DynaActionForm) {
values = (String[])((DynaActionForm)form).get(name);
} else {
try {
PropertyDescriptor[] descriptors = Introspector.getBeanInfo(form.getClass()).getPropertyDescriptors();
for (int i = 0; i < descriptors.length; i++) {
if (descriptors[i].getName().equals(name)) {
values = (String[])descriptors[i].getReadMethod().invoke(form, null);
break;
}
}
} catch (Exception e) {
LOGGER.warn("getSelection()", e);
}
}
if (values.length == 0) {
return null;
} else if (values.length == 1 && EmptyMarkerTag.EMPTY_MARKER.equals(values[0])) {
return new String[0];
} else {
List selection = new ArrayList();
for (int i = 0; i < values.length; i++) {
if (!EmptyMarkerTag.EMPTY_MARKER.equals(values[i])) {
selection.add(values[i]);
}
}
return (String[])selection.toArray(new String[selection.size()]);
}
}
/**
* TODO documentation
*
* @param form
* @param name
* @param values
*/
public static void setSelection(ActionForm form, String name, String[] values) {
if (form instanceof DynaActionForm) {
((DynaActionForm)form).set(name, values);
} else {
try {
PropertyDescriptor[] descriptors = Introspector.getBeanInfo(form.getClass()).getPropertyDescriptors();
for (int i = 0; i < descriptors.length; i++) {
if (descriptors[i].getName().equals(name)) {
Method setter = descriptors[i].getWriteMethod();
Class[] types = setter.getParameterTypes();
if (types.length == 1 && types[0].isArray() && types[0] == String.class) {
setter.invoke(form, values);
break;
}
}
}
} catch (Exception e) {
LOGGER.warn("getSelection()", e);
}
}
}
/**
* TODO documentation
*
* @param form
* @param name
*/
public static void resetSelection(ActionForm form, String name) {
if (form instanceof DynaActionForm) {
((DynaActionForm)form).set(name, new String[0]);
} else {
try {
PropertyDescriptor[] descriptors = Introspector.getBeanInfo(form.getClass()).getPropertyDescriptors();
for (int i = 0; i < descriptors.length; i++) {
if (descriptors[i].getName().equals(name)) {
Method setter = descriptors[i].getWriteMethod();
Class[] types = setter.getParameterTypes();
if (types.length == 1 && types[0].isArray() && types[0] == String.class) {
setter.invoke(form, new String[0]);
break;
}
}
}
} catch (Exception e) {
LOGGER.warn("getSelection()", e);
}
}
}
/**
* Parses the request searching for standard filtering and ordering
* parameters.
*
* @param request the received request.
* @return filtering and ordering parameters.
*/
public static SearchInfo getSearchInfo(HttpServletRequest request) {
SearchInfo info = new SearchInfo();
info.setLocale(RequestUtils.getUserLocale(request, null));
info.setFilters(request.getParameterValues("filter"));
info.setUnion(request.getParameter("union"));
info.setOrder(request.getParameter("order"));
return info;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy