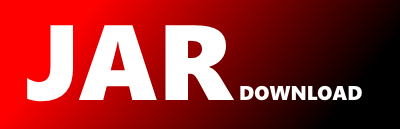
net.smartlab.web.Domain Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of smartweb Show documentation
Show all versions of smartweb Show documentation
SmartWeb is a web application development meta framework based on Jakarta Struts, Hibernate and other open source frameworks and libraries.
/*
* The SmartWeb Framework
* Copyright (C) 2004-2006
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA
*
* For further informations on the SmartWeb Framework please visit
*
* http://smartweb.sourceforge.net
*/
package net.smartlab.web;
import java.net.URL;
import net.smartlab.config.Configuration;
import net.smartlab.config.ConfigurationException;
import net.smartlab.web.config.DomainConfigurationStrategy;
import net.smartlab.web.config.FileDomainConfigurationStrategy;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import org.hibernate.HibernateException;
import org.hibernate.StaleObjectStateException;
import org.hibernate.Transaction;
/**
* @author rlogiacco
* @uml.dependency supplier="net.smartlab.web.config.DomainConfigurationStrategy"
* @uml.dependency supplier="net.smartlab.web.BusinessException"
* @uml.dependency supplier="net.smartlab.web.BusinessObjectFactory"
*/
public abstract class Domain implements ManageableDomain {
/**
* Provides the execution context through which additional informations can
* be transparently transmitted in a multiple tier architecture.
*/
final static ThreadLocal context = new ThreadLocal();
/**
* Provides logging capabilities to the domain.
*/
protected final Log logger = LogFactory.getLog(this.getClass());
/**
* The internal configuration.
*/
private Configuration config;
/**
* Strategy to retrieve the configuration file.
*/
private static DomainConfigurationStrategy strategy = new FileDomainConfigurationStrategy();
static {
try {
String strategy = System.getProperty("smartweb.domain.strategy");
if (strategy != null) {
Domain.strategy = (DomainConfigurationStrategy)Class.forName(strategy).newInstance();
} else {
LogFactory.getLog(Domain.class).warn("No configuration found: falling back to default configuration");
}
} catch (Exception e) {
LogFactory.getLog(Domain.class).fatal("Error configuring SmartWeb", e);
}
}
/**
* Starts a new transaction, allowing all the operations performed in the
* same context to be atomically applied (commit) or reverted (rollback).
* The context is identifyied by a BusinessObjectFactory
* instance but the context spans over all the instances sharing the same
* configuration.
*
* @param factory the identifier for the context
* @return an object representation of the transaction.
* @throws BusinessException if something wrong occurs during the operation.
*/
protected Transaction begin(BusinessObjectFactory factory) throws BusinessException {
try {
return factory.current().beginTransaction();
} catch (HibernateException he) {
logger.error("[ smartweb ] failed to begin transaction");
throw new BusinessException("persistence.error.begin", he);
} catch (DAOException boe) {
throw new BusinessException(boe);
}
}
/**
* Commits the specified transaction applying all the changes happened since
* its beginning.
*
* @param transaction the transaction to commit.
* @throws BusinessException if something wrong occurs during the operation.
*/
protected void commit(Transaction transaction) throws BusinessException {
try {
transaction.commit();
} catch (StaleObjectStateException sose) {
logger.info("[ smartweb ] optimistical locking collision");
throw new BusinessException("persistence.locking.collision", sose);
} catch (HibernateException he) {
logger.error("[ smartweb ] failed to commit transaction");
throw new BusinessException("persistence.error.commit", he);
}
}
/**
* Rollbacks the specified transaction reverting all the changes happened
* since its beginning.
*
* @param transaction the transaction to rollback.
* @throws BusinessException if something wrong occurs during the operation.
*/
protected void rollback(Transaction transaction) throws BusinessException {
try {
transaction.rollback();
} catch (HibernateException he) {
logger.warn("[ smartweb ] failed to rollback transaction");
}
}
/**
* Changes the strategy used to configure the domain.
*
* @param strategy an implementation of the
* DomainConfigurationStrategy
interface.
*/
public static void setConfigurationStrategy(DomainConfigurationStrategy strategy) {
Domain.strategy = strategy;
}
/**
* Subclasses should make their own constructors private and behave like
* singletons.
*/
protected Domain() {
if (logger.isDebugEnabled()) {
logger.debug(this.getClass().getName() + " instantiated.");
}
}
/**
* Returns the Configuration
instance used by this instance.
*
* @return the Configuration
instance used by this instance.
* @throws ConfigurationException if something wrong occurs while reading
* the configuration file, usually meaning the configuration file is
* missing.
*/
public Configuration getConfiguration() throws ConfigurationException {
if (config == null) {
this.config = strategy.getConfiguration(this);
}
return config;
}
/**
* Returns the Configuration
instance used by this instance.
*
* @param filename the configuration file name.
* @return the Configuration
instance used by this instance.
* @throws ConfigurationException if something wrong occurs while reading
* the configuration file, usually meaning the configuration file is
* missing.
*/
public Configuration getConfiguration(String filename) throws ConfigurationException {
if (config == null) {
config = strategy.getConfiguration(this, filename);
}
return config;
}
/**
* Returns the first resource in the list available in the specified
* context.
*
* @param context the class identifying the search context.
* @param names the list, ordered from first to last, of resources to search
* for.
* @return the first resource in the list available in the context.
*/
public static URL getResource(Class context, String[] names) {
Log logger = LogFactory.getLog(context);
URL resource = null;
for (int i = 0; i < names.length; i++) {
resource = context.getResource(names[i]);
logger.trace(" trying `" + names[i] + "`");
if (resource != null) {
break;
}
}
logger.debug(" resource is `" + resource + "`");
return resource;
}
/**
* Returns the name of the last archive containing the class or an
* empty
string if the class is in no archive.
*
* @param type the class to search for.
* @return the name of the last archive containing the class or an
* empty
string if the class is in no archive.
*/
public static String getLastArchiveName(Class type) {
Log logger = LogFactory.getLog(type);
String path = type.getProtectionDomain().getCodeSource().getLocation().getFile();
logger.debug(" archive path is `" + path + "`");
return path.substring(path.lastIndexOf('/') + 1);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy