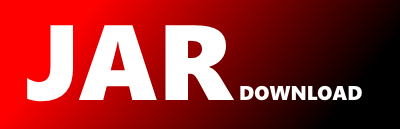
net.smartlab.web.PropertiesEnumeration Maven / Gradle / Ivy
/*
* The SmartWeb Framework
* Copyright (C) 2004-2006
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA
*
* For further informations on the SmartWeb Framework please visit
*
* http://smartweb.sourceforge.net
*/
package net.smartlab.web;
import java.lang.reflect.Constructor;
import java.net.URL;
import java.util.HashMap;
import java.util.Iterator;
import java.util.Map;
import java.util.Properties;
import java.util.TreeMap;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
/**
* This class allows to use a properties file to define enumeration elements but
* needs the definition of two public constructors. Implementors of this class need to
* define two constructors: one without parameters which simply calls
* super()
and an additional one taking two strings and calling
* super(String, String)
.
*
* @author rlogiacco
*/
public abstract class PropertiesEnumeration extends StringEnumeration {
private static final long serialVersionUID = 228095287903246251L;
private static final Class[] TYPES = new Class[] {String.class, String.class};
private static Map enumerations = new HashMap();
/**
* Provides logging capabilities.
*/
protected final static Log logger = LogFactory.getLog(PropertiesEnumeration.class);
/**
* The empty public constructor which MUST be exposed by subclasses.
*/
public PropertiesEnumeration() {
super();
}
/**
* The parametrized public constructor which MUST be exposed by subclasses.
*
* @param code the enumeration element code
* @param display the enumeration element display name
*/
public PropertiesEnumeration(String code, String display) {
super(code, display);
}
/**
* @see net.smartlab.web.StringEnumeration#decode(java.lang.String)
*/
public StringEnumeration decode(String code) {
if (logger.isDebugEnabled()) {
logger.debug("decode(\"" + code + "\") - start");
}
Map values = (Map)enumerations.get(this.getClass());
if (values == null) {
synchronized (this.getClass()) {
try {
String classname = this.getClass().getName();
URL url = this.getClass().getResource(
classname.substring(classname.lastIndexOf('.') + 1) + ".properties");
Properties properties = new Properties();
properties.load(url.openStream());
Iterator entries = properties.entrySet().iterator();
Constructor instantiator = this.getClass().getConstructor(TYPES);
values = new TreeMap();
while (entries.hasNext()) {
Map.Entry entry = (Map.Entry)entries.next();
if (logger.isTraceEnabled()) {
logger.trace(" adding (" + entry.getKey() + ", " + entry.getValue() + ")");
}
values.put(entry.getKey(), instantiator.newInstance(new Object[] {entry.getKey(),
entry.getValue()}));
}
enumerations.put(this.getClass(), values);
} catch (NoSuchMethodException nsme) {
logger.fatal("missing constructor " + this.getClass().getName() + "(String, String)", nsme);
} catch (Exception e) {
logger.error("decode() - failed", e);
}
}
}
return (StringEnumeration)values.get(code);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy