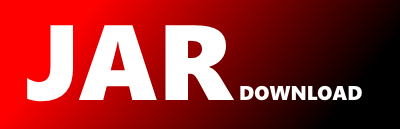
net.smartlab.web.Action Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of smartweb Show documentation
Show all versions of smartweb Show documentation
SmartWeb is a web application development meta framework based on Jakarta Struts, Hibernate and other open source frameworks and libraries.
/*
* The SmartWeb Framework
* Copyright (C) 2004-2006
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA
*
* For further informations on the SmartWeb Framework please visit
*
* http://smartweb.sourceforge.net
*/
package net.smartlab.web;
import java.io.InputStream;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
import java.util.Locale;
import java.util.MissingResourceException;
import java.util.Properties;
import javax.servlet.ServletContext;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import net.smartlab.web.bean.Valorizer;
import org.apache.commons.beanutils.locale.LocaleBeanUtilsBean;
import org.apache.commons.beanutils.locale.LocaleConvertUtilsBean;
import org.apache.commons.collections.FastHashMap;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import org.apache.struts.Globals;
import org.apache.struts.action.ActionErrors;
import org.apache.struts.action.ActionForm;
import org.apache.struts.action.ActionForward;
import org.apache.struts.action.ActionMapping;
import org.apache.struts.action.ActionMessage;
import org.apache.struts.action.ActionMessages;
import org.apache.struts.action.DynaActionForm;
/**
* This class represents the controller part of the MVC pattern.
* Extend this class to provide a specific set of operations to be performed in
* response of a user selection.
*
* @author rlogiacco
* @see org.apache.struts.action.Action
* @uml.dependency supplier="net.smartlab.web.ActionException"
* @uml.dependency supplier="net.smartlab.web.bean.Valorizer"
*/
public abstract class Action extends org.apache.struts.action.Action {
/**
* Identifies the default resource the request will be forwarded.
*/
public final static ActionForward DEFAULT_FORWARD = new ActionForward("default");
/**
* Provides logging capabilities to the action.
*/
protected final Log logger = LogFactory.getLog(this.getClass());
/**
* This method performs some common operations then redirects control to the
* abstract execute
.
*
* @see org.apache.struts.action.Action#execute(org.apache.struts.action.ActionMapping,
* org.apache.struts.action.ActionForm,
* javax.servlet.http.HttpServletRequest,
* javax.servlet.http.HttpServletResponse)
*/
public ActionForward execute(ActionMapping mapping, ActionForm form, HttpServletRequest request,
HttpServletResponse response) throws Exception {
if (logger.isDebugEnabled()) {
logger.debug("execute(" + mapping.getPath() + ") - start");
}
ActionForward forward = null;
if (super.isCancelled(request)) {
if (logger.isDebugEnabled()) {
logger.debug("execute(" + mapping.getPath() + ") - cancel");
}
forward = this.cancel(form, request, response, mapping);
if (forward == DEFAULT_FORWARD) {
return mapping.getInputForward();
} else {
return forward;
}
}
forward = this.execute(form, request, response, mapping);
try {
if (request.getAttribute(Globals.ERROR_KEY) != null && forward == DEFAULT_FORWARD) {
if (logger.isDebugEnabled()) {
logger.debug("execute(" + mapping.getPath() + ") - errors");
if (logger.isTraceEnabled()) {
logger.trace(" " + request.getAttribute(Globals.ERROR_KEY));
}
}
return mapping.getInputForward();
}
return forward;
} finally {
if (logger.isTraceEnabled()) {
logger.trace(" forward = " + forward);
}
}
}
/**
* Implement this method to provide a custom response to a user input.
*
* @param form the html form submitted with this request.
* @param request the user request.
* @param response the representation of the response channel.
* @param mapping the system control mapping.
* @return the name of a defined global or local forward.
* @throws Exception if something unexpected happend during the request
* execution.
*/
protected abstract ActionForward execute(ActionForm form, HttpServletRequest request, HttpServletResponse response,
ActionMapping mapping) throws Exception;
/**
* Describes the operations sequence to be performed upon cancellation of a
* form or wizard returning the mapping to redirect to. By default this
* method simply does nothing more than redirecting to the
* input
path.
*
* @param form the html form submitted with this request.
* @param request the user request.
* @param response the representation of the response channel.
* @param mapping the system control mapping.
* @return the name of a defined global or local forward.
* @throws Exception if something unexpected happend during the request
* execution.
*/
protected ActionForward cancel(ActionForm form, HttpServletRequest request, HttpServletResponse response,
ActionMapping mapping) throws Exception {
return DEFAULT_FORWARD;
}
/**
* Valorizes a bean
instance with the values providen with
* the user submitted html form. This method uses an improved version of the
* introspection paradigm to discover properties bindings.
*
* @param form the user submitted html form containing the values to be
* read.
* @param bean the bean instance to be valorized.
* @param locale the user locale for values parsing.
* @throws ActionException if the valorization fails, usually due to
* inconsistencies between the bean and the submitted form.
*/
protected void valorize(ActionForm form, Object bean, Locale locale) throws ActionException {
try {
if (logger.isDebugEnabled()) {
logger.debug("valorize(" + form + ", " + bean + ") - start");
}
// FIXME
// this.getConverter(locale).copyProperties(bean, form);
Valorizer.copy(form, bean, locale);
} catch (Exception e) {
logger.debug("valorize(" + form + ", " + bean + ") - error", e);
throw new ActionException("action.error.valorize", e);
}
}
/**
* Populates, or prevalorizes, an html form with the values of a
* bean
instance . This method uses an improved version of
* the introspection paradigm to discover properties bindings.
*
* @param form the form to be populated.
* @param bean the bean instance containing the values to be written in the
* form.
* @param locale the user locale for values parsing.
* @throws ActionException if the valorization fails, usually due to
* inconsistencies between the bean and the submitted form.
*/
protected void populate(ActionForm form, Object bean, Locale locale) throws ActionException {
try {
if (logger.isDebugEnabled()) {
logger.debug("populate(" + form + ", " + bean + ") - start");
}
// FIXME
// this.getConverter(locale).copyProperties(form,bean);
Valorizer.copy(bean, form, locale);
} catch (Exception e) {
logger.debug("populate(" + form + ", " + bean + ") - error", e);
throw new ActionException("action.error.populate", e);
}
}
/**
* @TODO documentation
* @param locale
* @return
*/
private LocaleBeanUtilsBean getConverter(Locale locale) {
LocaleConvertUtilsBean converter = new LocaleConvertUtilsBean() {
protected FastHashMap create(Locale locale) {
FastHashMap converter = super.create(locale);
converter.setFast(false);
// FIXME add converters
converter.setFast(true);
return converter;
}
};
converter.setDefaultLocale(locale);
return new LocaleBeanUtilsBean(converter);
}
/**
* Ensures the specified html form has all its fields resetted to their
* initial value.
*
* @param form the form to be cleaned up.
* @param request the user request.
* @param mapping the system control mapping.
*/
public void reset(ActionForm form, HttpServletRequest request, ActionMapping mapping) {
if (form instanceof DynaActionForm) {
((DynaActionForm)form).initialize(mapping);
} else {
form.reset(mapping, request);
}
}
/**
* TODO documentation
*
* @param request
* @return
*/
protected boolean hasErrors(HttpServletRequest request) {
return request.getAttribute(Globals.ERROR_KEY) != null;
}
/**
* TODO documentation
*
* @param message
* @param request
*/
protected void addError(ActionMessage message, HttpServletRequest request) {
this.addError(ActionMessages.GLOBAL_MESSAGE, message, request);
}
/**
* TODO documentation
*
* @param property
* @param message
* @param request
*/
protected void addError(String property, ActionMessage message, HttpServletRequest request) {
ActionErrors errors = (ActionErrors)request.getAttribute(Globals.ERROR_KEY);
if (errors == null) {
errors = new ActionErrors();
request.setAttribute(Globals.ERROR_KEY, errors);
}
errors.add(property, message);
}
/**
* TODO documentation
*
* @param name
* @param request
* @return
*/
public boolean isChecked(String name, HttpServletRequest request) {
if ("on".equalsIgnoreCase(request.getParameter("group"))
|| "true".equalsIgnoreCase(request.getParameter("group"))
|| "yes".equalsIgnoreCase(request.getParameter("group"))) {
return true;
} else {
return false;
}
}
/**
* TODO documentation
*
* @return
*/
protected ServletContext getServletContext() {
return super.getServlet().getServletContext();
}
/**
* TODO documentation
*
* @param path
* @return
*/
protected String getRealPath(String path) {
return this.getServletContext().getRealPath(path);
}
/**
* TODO documentation
*
* @return
*/
protected Properties getProperties() {
Class type = this.getClass();
List inputs = new ArrayList();
Properties result = new Properties();
while (type.getSuperclass().isAssignableFrom(Action.class)) {
try {
InputStream in = this.getClass().getResourceAsStream(this.getClass().getName() + ".prop");
inputs.add(in);
} catch (Exception e) {
if (logger.isDebugEnabled()) {
logger.debug("getProperties() - descend", e);
}
}
type = type.getSuperclass();
}
Iterator iterator = inputs.iterator();
while (iterator.hasNext()) {
try {
InputStream in = (InputStream)iterator.next();
Properties properties = new Properties();
properties.load(in);
result.putAll(properties);
} catch (Exception e) {
if (logger.isDebugEnabled()) {
logger.debug("getProperties() - load", e);
}
}
}
throw new MissingResourceException("No properties defined for action", this.getClass().getName(), null);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy