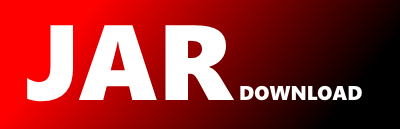
net.smartlab.web.EnterpriseDomain Maven / Gradle / Ivy
/*
* The SmartWeb Framework
* Copyright (C) 2004-2006
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA
*
* For further informations on the SmartWeb Framework please visit
*
* http://smartweb.sourceforge.net
*/
package net.smartlab.web;
import java.io.Serializable;
import java.lang.reflect.InvocationTargetException;
import java.rmi.RemoteException;
import java.util.Collection;
import java.util.HashMap;
import java.util.Set;
import javax.ejb.EJBObject;
import javax.ejb.SessionBean;
/**
* @TODO documentation
* @author [email protected]
*/
public abstract class EnterpriseDomain implements SessionBean {
private static final long serialVersionUID = -2893852982428251155L;
public static class RequestContext implements Serializable {
private static final long serialVersionUID = 2724059707578588014L;
/**
* Attributes container.
*/
private HashMap attributes = new HashMap();
public boolean contains(String key) {
return attributes.containsKey(key);
}
public Set getAttributeNames() {
return attributes.keySet();
}
public Serializable setAttribute(String key, Serializable value) {
return (Serializable)attributes.put(key, value);
}
public Serializable removeAttribute(String key) {
return (Serializable)attributes.remove(key);
}
public int size() {
return attributes.size();
}
public Collection getAttributesMap() {
return attributes.values();
}
}
public Object execute(String method, Object[] arguments, String[] types, EnterpriseDomain.RequestContext context)
throws RemoteException, InvocationTargetException {
try {
Domain.context.set(context);
return this.getClass().getMethod(method, EnterpriseDomain.forNames(types)).invoke(this, arguments);
} catch (IllegalArgumentException iae) {
throw new InvocationTargetException(iae);
} catch (IllegalAccessException iae) {
throw new InvocationTargetException(iae);
} catch (NoSuchMethodException nsme) {
throw new InvocationTargetException(nsme);
} catch (ClassNotFoundException cnfe) {
throw new InvocationTargetException(cnfe);
} finally {
try {
BusinessObjectFactory.close();
} catch (DAOException daoe) {
throw new InvocationTargetException(daoe);
}
}
}
protected static RequestContext getContext() {
return (RequestContext)Domain.context.get();
}
/**
* Get the classes from names. argTypes is not null.
*
* @param argTypes The method argument classes' names
* @return ClassNotFoundException if any class can not be located
*/
private static Class[] forNames(String[] argTypes) throws ClassNotFoundException {
if (argTypes == null) {
return null;
}
Class[] result = new Class[argTypes.length];
for (int i = 0; i < argTypes.length; i++) {
if (EnterpriseDomainBuilder.primitives.containsKey(argTypes[i])) {
result[i] = (Class)EnterpriseDomainBuilder.primitives.get(argTypes[i]);
} else {
result[i] = Class.forName(argTypes[i]);
}
}
return result;
}
/**
* @TODO documentation
* @author [email protected]
*/
public static interface Remote extends EJBObject {
/**
* @TODO documentation
* @param method
* @param arguments
* @param types
* @param context
* @return
* @throws RemoteException
*/
public Object execute(String method, Object[] arguments, String[] types,
EnterpriseDomain.RequestContext context) throws RemoteException;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy