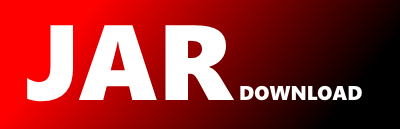
net.smartlab.web.Enumeration Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of smartweb Show documentation
Show all versions of smartweb Show documentation
SmartWeb is a web application development meta framework based on Jakarta Struts, Hibernate and other open source frameworks and libraries.
/*
* The SmartWeb Framework
* Copyright (C) 2004-2006
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA
*
* For further informations on the SmartWeb Framework please visit
*
* http://smartweb.sourceforge.net
*/
package net.smartlab.web;
import java.io.Serializable;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Types;
import org.hibernate.Hibernate;
import org.hibernate.HibernateException;
import org.hibernate.usertype.UserType;
/**
* Represents a meta type used to constraint a property to accept only
* pre-defined admitted values. Whenever a property needs some sort of
* constraint on assignable values, like person gender or process status, a
* subclass of Enumeration
can be used to enumerate all possible
* values.
*
* @author rlogiacco
*/
public abstract class Enumeration implements UserType, Serializable {
private final static long serialVersionUID = 8849967605617496075L;
/**
* Type of the persisted field reference.
*/
private final static int[] TYPES = new int[] {Types.SMALLINT};
/**
* The unique identifier used to represent the choice onto the persisted
* field.
*
* @uml.property name="id"
*/
private final int id;
/**
* A brief, human understandable, description of the choice.
*
* @uml.property name="display"
*/
private final String display;
/**
* Default constructor used for internal purposes only.
*/
protected Enumeration() {
// Default constructor
this.id = -1;
this.display = null;
}
/**
* Constructs an available choice on a unique identifier and a brief, human
* understandable, description.
*
* @param id the unique identifier for this choice.
* @param display a short, human understandable, description of the choice.
*/
protected Enumeration(int id, String display) {
this.id = id;
this.display = display;
}
/**
* Returns the unique identifier for the choice.
*
* @return the unique identifier for the choice.
* @uml.property name="id"
*/
public int getId() {
return id;
}
/**
* Returns the brief description of the choice.
*
* @return the brief description of the choice.
* @uml.property name="display"
*/
public String getDisplay() {
return display;
}
/**
* Decodes a unique identifier into an instance of this class. This method
* can not return null
values, instead it should define
* a default value.
*
* @param id the identifier to decode.
* @return an instance of this class.
*/
public abstract Enumeration decode(int id);
/**
* @see java.lang.Object#equals(java.lang.Object)
*/
public boolean equals(Object other) {
if (other != null && this.getClass().equals(other.getClass())) {
if (((Enumeration)other).id == id) {
return true;
}
}
return false;
}
/**
* @see java.lang.Object#hashCode()
*/
public int hashCode() {
return id;
}
/**
* @see org.hibernate.usertype.UserType#deepCopy(java.lang.Object)
*/
public Object deepCopy(Object value) throws HibernateException {
return value;
}
/**
* @see org.hibernate.usertype.UserType#equals(java.lang.Object,
* java.lang.Object)
*/
public boolean equals(Object src, Object dst) throws HibernateException {
if (src == dst) {
return true;
}
if (src == null || dst == null) {
return false;
}
return Hibernate.INTEGER.isEqual(src, dst);
}
/**
* @see org.hibernate.usertype.UserType#isMutable()
*/
public boolean isMutable() {
return false;
}
/**
* @see org.hibernate.usertype.UserType#nullSafeGet(java.sql.ResultSet,
* java.lang.String[], java.lang.Object)
*/
public Object nullSafeGet(ResultSet rows, String[] names, Object owner) throws HibernateException, SQLException {
try {
int id = ((Integer)Hibernate.INTEGER.nullSafeGet(rows, names[0])).intValue();
return this.decode(id);
} catch (NullPointerException npe) {
return null;
}
}
/**
* @see org.hibernate.usertype.UserType#nullSafeSet(java.sql.PreparedStatement,
* java.lang.Object, int)
*/
public void nullSafeSet(PreparedStatement statement, Object value, int index) throws HibernateException,
SQLException {
if (value == null) {
statement.setNull(index, TYPES[0]);
} else if (value instanceof String) {
statement.setString(index, (String)value);
} else if (value instanceof Number) {
statement.setInt(index, ((Number)value).intValue());
} else {
try {
statement.setInt(index, ((Enumeration)value).getId());
} catch (ClassCastException cce) {
throw new HibernateException(cce);
}
}
}
/**
* @see org.hibernate.usertype.UserType#returnedClass()
*/
public Class returnedClass() {
return this.getClass();
}
/**
* @see org.hibernate.usertype.UserType#sqlTypes()
*/
public int[] sqlTypes() {
return TYPES;
}
/**
* @see org.hibernate.usertype.UserType#assemble(java.io.Serializable,
* java.lang.Object)
*/
public Object assemble(Serializable arg0, Object arg1) throws HibernateException {
return null;
// FIXME: may be an HibernateException is better
}
/**
* @see org.hibernate.usertype.UserType#disassemble(java.lang.Object)
*/
public Serializable disassemble(Object arg0) throws HibernateException {
return null;
// FIXME: may be an HibernateException is better
}
/**
* @see org.hibernate.usertype.UserType#hashCode(java.lang.Object)
*/
public int hashCode(Object obj) throws HibernateException {
return ((Enumeration)obj).getId();
}
/**
* @see org.hibernate.usertype.UserType#replace(java.lang.Object,
* java.lang.Object, java.lang.Object)
*/
public Object replace(Object arg0, Object arg1, Object arg2) throws HibernateException {
return null;
// FIXME: may be an HibernateException is better
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy