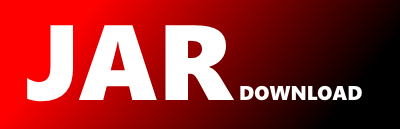
net.smartlab.web.StringEnumeration Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of smartweb Show documentation
Show all versions of smartweb Show documentation
SmartWeb is a web application development meta framework based on Jakarta Struts, Hibernate and other open source frameworks and libraries.
/*
* The SmartWeb Framework
* Copyright (C) 2004-2006
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA
*
* For further informations on the SmartWeb Framework please visit
*
* http://smartweb.sourceforge.net
*/
package net.smartlab.web;
import java.io.Serializable;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Types;
import org.hibernate.Hibernate;
import org.hibernate.HibernateException;
import org.hibernate.usertype.UserType;
/**
* This class allows to use string to define enumeration elements identifier but
* needs the definition of two constructors. Implementors of this class need to
* define two constructors: one without parameters which simply calls
* super()
and an additional one taking two strings and calling
* super(String, String)
.
*
* @author rlogiacco
*/
public abstract class StringEnumeration implements Serializable, UserType, Comparable {
private final static long serialVersionUID = -1298346029203487615L;
/**
* The SQL column type.
*/
private final static int[] TYPES = new int[] {Types.VARCHAR};
/**
* TODO documentation
*
* @uml.property name="code"
*/
protected String code;
private String display;
/**
* Default constructor used for internal purposes only.
*/
protected StringEnumeration() {
super();
}
/**
* Constructs an available choice on a unique identifier and a brief, human
* understandable, description.
*
* @param id the unique identifier for this choice.
* @param display a short, human understandable, description of the choice.
*/
protected StringEnumeration(String code, String display) {
this.code = code;
this.display = display;
}
/**
* Returns the enumeration element code.
*
* @return the enumeration element code.
* @uml.property name="code"
*/
public String getCode() {
return code;
}
/**
* Returns the enumeration element display name.
*
* @return the enumeration element display name.
*/
public String getDisplay() {
return display;
}
/**
* Decodes a unique code into an instance of this class. This method can
* not return null
values, instead it should define a
* default value.
*
* @param code the code to decode.
* @return an instance of this class.
*/
public abstract StringEnumeration decode(String code);
/**
* @see java.lang.Object#equals(java.lang.Object)
*/
public boolean equals(Object other) {
if (other != null && this.getClass().equals(other.getClass())) {
if (((StringEnumeration)other).code.equals(code)) {
return true;
}
}
return false;
}
/**
* @see java.lang.Object#hashCode()
*/
public int hashCode() {
return code.hashCode();
}
/**
* @see org.hibernate.usertype.UserType#sqlTypes()
*/
public int[] sqlTypes() {
return TYPES;
}
/**
* @see org.hibernate.usertype.UserType#returnedClass()
*/
public Class returnedClass() {
return this.getClass();
}
/**
* @see org.hibernate.usertype.UserType#equals(java.lang.Object,
* java.lang.Object)
*/
public boolean equals(Object src, Object dst) throws HibernateException {
if (src == dst) {
return true;
}
if (src == null || dst == null) {
return false;
}
return Hibernate.STRING.isEqual(src, dst);
}
/**
* @see org.hibernate.usertype.UserType#nullSafeGet(java.sql.ResultSet,
* java.lang.String[], java.lang.Object)
*/
public Object nullSafeGet(ResultSet rows, String[] names, Object owner) throws HibernateException, SQLException {
String code = ((String)Hibernate.STRING.nullSafeGet(rows, names[0]));
return this.decode(code);
}
/**
* @see org.hibernate.usertype.UserType#nullSafeSet(java.sql.PreparedStatement,
* java.lang.Object, int)
*/
public void nullSafeSet(PreparedStatement statement, Object value, int index) throws HibernateException,
SQLException {
if (value == null) {
statement.setNull(index, TYPES[0]);
} else {
try {
statement.setString(index, ((StringEnumeration)value).getCode());
} catch (ClassCastException cce) {
throw new HibernateException(cce);
}
}
}
/**
* @see org.hibernate.usertype.UserType#deepCopy(java.lang.Object)
*/
public Object deepCopy(Object value) throws HibernateException {
return value;
}
/**
* @see org.hibernate.usertype.UserType#isMutable()
*/
public boolean isMutable() {
return false;
}
/**
* @see org.hibernate.usertype.UserType#assemble(java.io.Serializable,
* java.lang.Object) TODO implement
*/
public Object assemble(Serializable arg0, Object arg1) throws HibernateException {
// TODO Auto-generated method stub
return null;
}
/**
* @see org.hibernate.usertype.UserType#disassemble(java.lang.Object) TODO
* implement
*/
public Serializable disassemble(Object arg0) throws HibernateException {
// TODO Auto-generated method stub
return null;
}
/**
* @see org.hibernate.usertype.UserType#hashCode(java.lang.Object)
*/
public int hashCode(Object obj) throws HibernateException {
return ((Enumeration)obj).hashCode();
}
/**
* @see org.hibernate.usertype.UserType#replace(java.lang.Object,
* java.lang.Object, java.lang.Object) TODO implement
*/
public Object replace(Object arg0, Object arg1, Object arg2) throws HibernateException {
return null;
}
/**
* @see java.lang.Comparable#compareTo(T)
*/
public int compareTo(Object obj) {
try {
return this.getCode().compareTo(((StringEnumeration)obj).code);
} catch (ClassCastException cce) {
return 0;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy