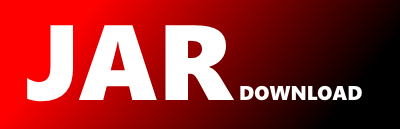
net.smartlab.web.bean.Valorizer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of smartweb Show documentation
Show all versions of smartweb Show documentation
SmartWeb is a web application development meta framework based on Jakarta Struts, Hibernate and other open source frameworks and libraries.
/*
* The SmartWeb Framework
* Copyright (C) 2004-2006
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA
*
* For further informations on the SmartWeb Framework please visit
*
* http://smartweb.sourceforge.net
*/
package net.smartlab.web.bean;
import java.beans.PropertyDescriptor;
import java.lang.reflect.InvocationTargetException;
import java.util.Iterator;
import java.util.Locale;
import java.util.Map;
import org.apache.commons.beanutils.DynaBean;
import org.apache.commons.beanutils.DynaClass;
import org.apache.commons.beanutils.DynaProperty;
import org.apache.commons.beanutils.PropertyUtils;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
/**
* TODO class documentation
*
* @author rlogiacco
* @uml.dependency supplier="net.smartlab.web.bean.ValorizationException"
*/
public class Valorizer {
/* # net.smartlab.web.bean.ValorizationException Dependency_Link1 */
/* # net.smartlab.web.bean.ValorizationException ve */
private static Log logger = LogFactory.getLog(Valorizer.class);
private static ConverterManager converter = ConverterManager.getDefault();
/**
* TODO documentation
*
* @param bean
* @param name
* @param value
* @param locale
* @throws ValorizationException
* @throws ConversionException
*/
protected static void set(Object bean, String name, Object value, Locale locale) throws ValorizationException,
ConversionException {
if (logger.isTraceEnabled()) {
logger.trace("setProperty(" + bean + ", " + name + ", " + value + ", " + locale + ")");
}
try {
// Resolve any nested expression to get the actual target bean
Object target = bean;
int delim = name.lastIndexOf(PropertyUtils.NESTED_DELIM);
if (delim >= 0) {
try {
target = PropertyUtils.getProperty(bean, name.substring(0, delim));
} catch (NoSuchMethodException e) {
// Skip this property setter
return;
}
name = name.substring(delim + 1);
if (logger.isTraceEnabled()) {
logger.trace(" target bean = " + target);
logger.trace(" target name = " + name);
}
}
// Simple name of target property
String property = null;
// Java type of target property
Class type = null;
// Indexed subscript value (if any)
int index = -1;
// Mapped key value (if any)
String key = null;
// Calculate the target property name, index, and key values
property = name;
int i = property.indexOf(PropertyUtils.INDEXED_DELIM);
if (i >= 0) {
int k = property.indexOf(PropertyUtils.INDEXED_DELIM2);
try {
index = Integer.parseInt(property.substring(i + 1, k));
} catch (NumberFormatException nfe) {
logger.debug(nfe);
}
property = property.substring(0, i);
}
int j = property.indexOf(PropertyUtils.MAPPED_DELIM);
if (j >= 0) {
int k = property.indexOf(PropertyUtils.MAPPED_DELIM2);
try {
key = property.substring(j + 1, k);
} catch (IndexOutOfBoundsException ioobe) {
logger.debug(ioobe);
}
property = property.substring(0, j);
}
// Calculate the target property type
if (target instanceof DynaBean) {
DynaClass dynaClass = ((DynaBean)target).getDynaClass();
DynaProperty dynaProperty = dynaClass.getDynaProperty(property);
if (dynaProperty == null) {
// Skip this property setter
return;
}
type = dynaProperty.getType();
} else {
PropertyDescriptor descriptor = null;
try {
descriptor = PropertyUtils.getPropertyDescriptor(target, name);
if (descriptor == null) {
// Skip this property setter
return;
}
} catch (NoSuchMethodException nsme) {
// Skip this property setter
return;
}
type = descriptor.getPropertyType();
if (type == null) {
// Most likely an indexed setter on a POJB only
if (logger.isTraceEnabled()) {
logger.trace(" target type for property '" + property + "' is null, so skipping this setter");
}
return;
}
}
if (logger.isTraceEnabled()) {
logger.trace(" target property = " + property + ", type = " + type + ", index = " + index
+ ", key = " + key);
}
// Convert the specified value to the required type and store it
if (index >= 0) {
converter.convert(type, value, locale);
try {
PropertyUtils.setIndexedProperty(target, property, index, value);
} catch (NoSuchMethodException e) {
throw new InvocationTargetException(e, "Cannot set " + property);
}
} else if (key != null) {
// Map based destination
// Maps do not know what the preferred data type is,
// so perform no conversions at all
try {
PropertyUtils.setMappedProperty(target, property, key, value);
} catch (NoSuchMethodException e) {
throw new InvocationTargetException(e, "Cannot set " + property);
}
} else {
// Bean destination
value = converter.convert(type, value, locale);
try {
PropertyUtils.setSimpleProperty(target, property, value);
} catch (NoSuchMethodException e) {
throw new InvocationTargetException(e, "Cannot set " + property);
}
}
} catch (IllegalAccessException iae) {
throw new ValorizationException(iae);
} catch (InvocationTargetException ite) {
throw new ValorizationException(ite.getTargetException());
}
}
/**
* TODO method documentation
*
* @param src
* @param dst
* @param locale
* @throws ConversionException
* @throws ValorizationException
*/
public static void copy(Object src, Object dst, Locale locale) throws ConversionException, ValorizationException {
if (dst == null) {
throw new IllegalArgumentException("No destination specified");
}
if (src == null) {
throw new IllegalArgumentException("No origin specified");
}
if (logger.isDebugEnabled()) {
logger.debug("populate(" + dst + ", " + src + ")");
}
if (src instanceof DynaBean) {
DynaProperty descriptors[] = ((DynaBean)src).getDynaClass().getDynaProperties();
for (int i = 0; i < descriptors.length; i++) {
String name = descriptors[i].getName();
if (PropertyUtils.isWriteable(dst, name)) {
Object value = ((DynaBean)src).get(name);
Valorizer.set(dst, name, value, locale);
}
}
} else if (src instanceof Map) {
Iterator names = ((Map)src).keySet().iterator();
while (names.hasNext()) {
String name = (String)names.next();
if (PropertyUtils.isWriteable(dst, name)) {
Object value = ((Map)src).get(name);
Valorizer.set(dst, name, value, locale);
}
}
} else {
PropertyDescriptor descriptors[] = PropertyUtils.getPropertyDescriptors(src);
for (int i = 0; i < descriptors.length; i++) {
String name = descriptors[i].getName();
if (!"class".equals(name) && PropertyUtils.isReadable(src, name)
&& PropertyUtils.isWriteable(dst, name)) {
try {
Object value = PropertyUtils.getSimpleProperty(src, name);
Valorizer.set(dst, name, value, locale);
} catch (NoSuchMethodException nsme) {
logger.warn(nsme);
} catch (IllegalAccessException iae) {
logger.warn(iae);
} catch (InvocationTargetException ite) {
logger.warn(ite);
}
}
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy