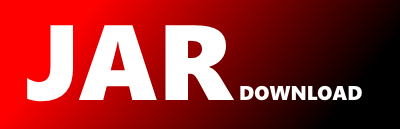
net.smartlab.web.history.Interceptor Maven / Gradle / Ivy
/*
* The SmartWeb Framework
* Copyright (C) 2004-2006
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA
*
* For further informations on the SmartWeb Framework please visit
*
* http://smartweb.sourceforge.net
*/
package net.smartlab.web.history;
import java.io.Serializable;
import java.sql.Timestamp;
import java.util.Iterator;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import org.hibernate.EntityMode;
import org.hibernate.HibernateException;
import org.hibernate.MappingException;
import org.hibernate.Transaction;
import org.hibernate.property.PropertyAccessor;
import org.hibernate.property.PropertyAccessorFactory;
import org.hibernate.property.Setter;
import org.hibernate.type.Type;
/**
* TODO documentation
*
* @author rlogiacco
*/
public class Interceptor implements org.hibernate.Interceptor {
/**
* Logger for this class
*/
protected final Log logger = LogFactory.getLog(Interceptor.class);
/**
* @see org.hibernate.Interceptor#onLoad(java.lang.Object, java.io.Serializable, java.lang.Object[], java.lang.String[], org.hibernate.type.Type[])
* TODO implement
*/
public boolean onLoad(Object arg0, Serializable arg1, Object[] arg2, String[] arg3, org.hibernate.type.Type[] arg4) throws org.hibernate.CallbackException {
// TODO Auto-generated method stub
return false;
}
/**
* @see org.hibernate.Interceptor#onFlushDirty(java.lang.Object, java.io.Serializable, java.lang.Object[], java.lang.Object[], java.lang.String[], org.hibernate.type.Type[])
* TODO implement
*/
public boolean onFlushDirty(Object entity, Serializable key, Object[] current, Object[] previous, String[] properties, Type[] types) throws org.hibernate.CallbackException {
if (logger.isDebugEnabled()) {
logger.debug("onFlushDirty(entity = " + entity + ", key = " + key + ", current = " + current + ", previous = " + previous
+ ", properties = " + properties + ", types = " + types + ") - start");
}
boolean modified = false;
if (entity instanceof HistoricizableBusinessObject) {
// instantiate historized object
HistorizedBusinessObject historized = ((HistoricizableBusinessObject)entity).getHistorized();
historized.setLastModified(new Timestamp(System.currentTimeMillis()));
try {
// put values into historized object
PropertyAccessor accessor = PropertyAccessorFactory.getPropertyAccessor(null);
for (int i = 0; i < properties.length; i++) {
if (!current[i].equals(previous[i])) {
modified = true;
Setter setter = accessor.getSetter(historized.getClass(), properties[i]);
// FIXME
//setter.set(historized, current[i]);
} else if (!(historized instanceof DifferenciallyHistorizedBusinessObject)) {
Setter setter = accessor.getSetter(historized.getClass(), properties[i]);
// FIXME
//setter.set(historized, current[i]);
}
}
if (modified) {
//TODO save the historized object
}
} catch (MappingException me) {
logger.error("onFlushDirty(entity = " + entity + ", key = " + key + ", current = " + current + ", previous = " + previous
+ ", properties = " + properties + ", types = " + types + ") - error", me);
} catch (HibernateException he) {
logger.error("onFlushDirty(entity = " + entity + ", key = " + key + ", current = " + current + ", previous = " + previous
+ ", properties = " + properties + ", types = " + types + ") - error", he);
}
}
return modified;
}
/**
* @see org.hibernate.Interceptor#onSave(java.lang.Object, java.io.Serializable, java.lang.Object[], java.lang.String[], org.hibernate.type.Type[])
* TODO implement
*/
public boolean onSave(Object arg0, Serializable arg1, Object[] arg2, String[] arg3, org.hibernate.type.Type[] arg4) throws org.hibernate.CallbackException {
// TODO Auto-generated method stub
return false;
}
/**
* @see org.hibernate.Interceptor#onDelete(java.lang.Object, java.io.Serializable, java.lang.Object[], java.lang.String[], org.hibernate.type.Type[])
* TODO implement
*/
public void onDelete(Object arg0, Serializable arg1, Object[] arg2, String[] arg3, org.hibernate.type.Type[] arg4) throws org.hibernate.CallbackException {
// TODO Auto-generated method stub
}
/**
* @see org.hibernate.Interceptor#onCollectionRecreate(java.lang.Object, java.io.Serializable)
* TODO implement
*/
public void onCollectionRecreate(Object arg0, Serializable arg1) throws org.hibernate.CallbackException {
// TODO Auto-generated method stub
}
/**
* @see org.hibernate.Interceptor#onCollectionRemove(java.lang.Object, java.io.Serializable)
* TODO implement
*/
public void onCollectionRemove(Object arg0, Serializable arg1) throws org.hibernate.CallbackException {
// TODO Auto-generated method stub
}
/**
* @see org.hibernate.Interceptor#onCollectionUpdate(java.lang.Object, java.io.Serializable)
* TODO implement
*/
public void onCollectionUpdate(Object arg0, Serializable arg1) throws org.hibernate.CallbackException {
// TODO Auto-generated method stub
}
/**
* @see org.hibernate.Interceptor#preFlush(java.util.Iterator)
* TODO implement
*/
public void preFlush(Iterator arg0) throws org.hibernate.CallbackException {
// TODO Auto-generated method stub
}
/**
* @see org.hibernate.Interceptor#postFlush(java.util.Iterator)
* TODO implement
*/
public void postFlush(Iterator arg0) throws org.hibernate.CallbackException {
// TODO Auto-generated method stub
}
/**
* @see org.hibernate.Interceptor#isTransient(java.lang.Object)
* TODO implement
*/
public Boolean isTransient(Object arg0) {
// TODO Auto-generated method stub
return null;
}
/**
* @see org.hibernate.Interceptor#findDirty(java.lang.Object, java.io.Serializable, java.lang.Object[], java.lang.Object[], java.lang.String[], org.hibernate.type.Type[])
* TODO implement
*/
public int[] findDirty(Object arg0, Serializable arg1, Object[] arg2, Object[] arg3, String[] arg4, org.hibernate.type.Type[] arg5) {
// TODO Auto-generated method stub
return null;
}
/**
* @see org.hibernate.Interceptor#instantiate(java.lang.String, org.hibernate.EntityMode, java.io.Serializable)
* TODO implement
*/
public Object instantiate(String arg0, EntityMode arg1, Serializable arg2) throws org.hibernate.CallbackException {
// TODO Auto-generated method stub
return null;
}
/**
* @see org.hibernate.Interceptor#getEntityName(java.lang.Object)
* TODO implement
*/
public String getEntityName(Object arg0) throws org.hibernate.CallbackException {
// TODO Auto-generated method stub
return null;
}
/**
* @see org.hibernate.Interceptor#getEntity(java.lang.String, java.io.Serializable)
* TODO implement
*/
public Object getEntity(String arg0, Serializable arg1) throws org.hibernate.CallbackException {
// TODO Auto-generated method stub
return null;
}
/**
* @see org.hibernate.Interceptor#afterTransactionBegin(org.hibernate.Transaction)
* TODO implement
*/
public void afterTransactionBegin(Transaction arg0) {
// TODO Auto-generated method stub
}
/**
* @see org.hibernate.Interceptor#beforeTransactionCompletion(org.hibernate.Transaction)
* TODO implement
*/
public void beforeTransactionCompletion(Transaction arg0) {
// TODO Auto-generated method stub
}
/**
* @see org.hibernate.Interceptor#afterTransactionCompletion(org.hibernate.Transaction)
* TODO implement
*/
public void afterTransactionCompletion(Transaction arg0) {
// TODO Auto-generated method stub
}
/**
* @see org.hibernate.Interceptor#onPrepareStatement(java.lang.String)
* TODO implement
*/
public String onPrepareStatement(String arg0) {
// TODO Auto-generated method stub
return null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy