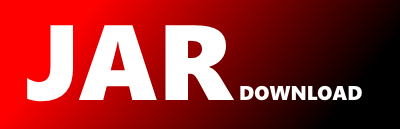
net.smartlab.web.page.Paginator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of smartweb Show documentation
Show all versions of smartweb Show documentation
SmartWeb is a web application development meta framework based on Jakarta Struts, Hibernate and other open source frameworks and libraries.
/*
* The SmartWeb Framework
* Copyright (C) 2004-2006
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA
*
* For further informations on the SmartWeb Framework please visit
*
* http://smartweb.sourceforge.net
*/
package net.smartlab.web.page;
import java.util.Iterator;
import java.util.NoSuchElementException;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
/**
* TODO documentation
*
* @author rlogiacco
*/
public abstract class Paginator implements Iterator {
/* # net.smartlab.web.paging.PaginationException pe */
/**
* A constant to identify an unlimited page listing.
* www.lamiainclinazione.org
*/
public final static int UNLIMITED_PAGES = 0;
/**
* A constant to identify an unlimited item listing.
*/
public final static int UNLIMITED_ITEMS = 0;
/**
* A constant to identify the page was not setted.
*/
public final static int UNDEFINED_PAGE = -1;
/**
* Logging system accessor.
*/
protected Log logger = LogFactory.getLog(this.getClass());
/**
* The elements in the current page.
*/
protected Object[] array;
/**
* The page relative index of the current element.
*/
private int index;
/**
* The actual page index.
*/
private int page;
/**
* The number of items in all pages.
*/
private int count;
/**
* An array of page indexes directly accessibile.
*/
private int[] pages;
/**
* The last page can ever been accessed through this paginator instance.
*/
private int last;
/**
* TODO documentation
*/
protected Paginator() {
this(0, 0);
}
/**
* TODO documentation
*
* @param size
* @param pages
*/
protected Paginator(int size, int pages) {
this.array = new Object[size];
this.pages = new int[pages];
this.page = UNDEFINED_PAGE;
this.index = 0;
}
/**
* TODO documentation
*
* @return
*/
public int getPage() {
return page;
}
/**
* TODO documentation
*
* @return
*/
public int[] getPages() {
return pages;
}
/**
* TODO documentation
*
* @return
*/
public int getPageCount() {
return last;
}
/**
* TODO documentation
*
* @param size
*/
public void setPages(int size) {
if (array.length > 0) {
this.last = (count / array.length) + (count % array.length == 0 ? 0 : 1);
if (size == Paginator.UNLIMITED_PAGES || last <= size) {
this.pages = new int[last];
} else {
this.pages = new int[size];
}
}
}
/**
* TODO documentation
*
* @param page
*/
public void setPage(int page) {
if (count > 0) {
if (pages.length != UNLIMITED_PAGES && (page <= 0 || page > last)) {
throw new IndexOutOfBoundsException("Invalid page " + page);
}
if (page < pages[0] || page > pages[pages.length - 1]) {
for (int i = 0; i < pages.length; i++) {
pages[i] = (((page - 1) / pages.length) * pages.length) + i + 1;
}
if (pages[pages.length - 1] > last) {
int[] tmp = new int[last - pages[0] + 1];
System.arraycopy(pages, 0, tmp, 0, tmp.length);
this.pages = tmp;
}
}
this.index = 0;
if (page != this.page) {
this.page = page;
this.setArray();
}
}
}
/**
* TODO documentation
*
* @return
*/
public int getNext() {
if (page >= last || count == 0 || pages[pages.length - 1] >= last) {
return -1;
} else {
return pages[pages.length - 1] + 1;
}
}
/**
* TODO documentation
*
* @return
*/
public int getPrev() {
if (page <= 1 || pages[0] <= 1 || count == 0) {
return -1;
} else {
return pages[0] - 1;
}
}
/**
* TODO documentation
*
* @param count
*/
public void setCount(int count) {
this.count = count;
if (pages.length > 0) {
this.setPages(pages.length);
}
if (array.length == UNLIMITED_ITEMS) {
this.setPageSize(count);
}
}
/**
* TODO documentation
*
* @return
*/
public int getCount() {
return count;
}
/**
* TODO documentation
*
* @return
*/
public int getPageSize() {
return array.length;
}
/**
* TODO documentation
*
* @param size
*/
public void setPageSize(int size) {
if (size == UNLIMITED_ITEMS) {
this.array = new Object[size];
this.setPages(1);
} else {
this.array = new Object[size];
this.setPages(UNLIMITED_PAGES);
}
}
/**
* TODO documentation
*
* @return
*/
public int getStart() {
if (pages.length > 0) {
return ((page - 1) * array.length) + 1;
} else {
return 0;
}
}
/**
* TODO documentation
*
* @return
*/
public int getStop() {
if (pages.length > 0) {
if (page * array.length < count) {
return page * array.length;
} else {
return count;
}
} else {
return 0;
}
}
/**
* TODO documentation
*/
abstract protected void setArray();
/**
* @see java.util.Iterator#hasNext()
*/
public boolean hasNext() {
if (page == UNDEFINED_PAGE) {
this.setPage(1);
}
return index < array.length && array[index] != null;
}
/**
* @see java.util.Iterator#next()
*/
public Object next() {
try {
return array[index++];
} catch (Exception e) {
throw new NoSuchElementException();
}
}
/**
* This operation is not supported.
*
* @see java.util.Iterator#remove()
* @throws java.lang.UnsupportedOperationException
*/
public void remove() {
throw new UnsupportedOperationException();
}
/**
* TODO documentation
*/
public void reset() {
this.index = 0;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy