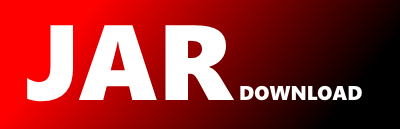
org.hibernate.impl.QueryImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of smartweb Show documentation
Show all versions of smartweb Show documentation
SmartWeb is a web application development meta framework based on Jakarta Struts, Hibernate and other open source frameworks and libraries.
//$Id: QueryImpl.java 8524 2005-11-04 21:28:49Z steveebersole $
package org.hibernate.impl;
import java.util.HashMap;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Set;
import org.hibernate.FlushMode;
import org.hibernate.HibernateException;
import org.hibernate.LockMode;
import org.hibernate.Query;
import org.hibernate.ScrollMode;
import org.hibernate.ScrollableResults;
import org.hibernate.Session;
import org.hibernate.engine.QueryParameters;
import org.hibernate.engine.SessionImplementor;
import org.hibernate.engine.TypedValue;
import org.hibernate.engine.query.ParameterMetadata;
import org.hibernate.type.Type;
/**
* default implementation of the Query interface, for "ordinary" HQL
* queries (not collection filters)
*
* @see CollectionFilterImpl
* @author gperrone
*/
public class QueryImpl extends AbstractQueryImpl {
private Map lockModes = new HashMap(2);
public QueryImpl(String queryString, FlushMode flushMode, SessionImplementor session, ParameterMetadata parameterMetadata) {
super(queryString, flushMode, session, parameterMetadata);
}
public QueryImpl(String queryString, SessionImplementor session, ParameterMetadata parameterMetadata) {
this(queryString, null, session, parameterMetadata);
}
public Iterator iterate() throws HibernateException {
verifyParameters();
Map namedParams = getNamedParams();
before();
try {
return getSession().iterate(expandParameterLists(namedParams), getQueryParameters(namedParams));
} finally {
after();
}
}
public ScrollableResults scroll() throws HibernateException {
return scroll(ScrollMode.SCROLL_INSENSITIVE);
}
public ScrollableResults scroll(ScrollMode scrollMode) throws HibernateException {
verifyParameters();
Map namedParams = getNamedParams();
before();
QueryParameters qp = getQueryParameters(namedParams);
qp.setScrollMode(scrollMode);
try {
return getSession().scroll(expandParameterLists(namedParams), qp);
} finally {
after();
}
}
public List list() throws HibernateException {
verifyParameters();
Map namedParams = getNamedParams();
before();
try {
return getSession().list(expandParameterLists(namedParams), getQueryParameters(namedParams));
} finally {
after();
}
}
public int executeUpdate() throws HibernateException {
verifyParameters();
Map namedParams = getNamedParams();
before();
try {
return getSession().executeUpdate(expandParameterLists(namedParams), getQueryParameters(namedParams));
} finally {
after();
}
}
public Query setLockMode(String alias, LockMode lockMode) {
lockModes.put(alias, lockMode);
return this;
}
protected Map getLockModes() {
return lockModes;
}
/**
* TODO documentation
*
* @return
*/
public int count() {
StringBuffer hqlQuery = new StringBuffer(this.getQueryString().toUpperCase());
StringBuffer hqlQueryBuffer = new StringBuffer();
hqlQueryBuffer.append("SELECT COUNT(*) ");
int start = hqlQuery.indexOf("FROM");
int stop = hqlQuery.indexOf("ORDER BY");
if (stop >= 0) {
hqlQueryBuffer.append(this.getQueryString().substring(start, stop));
} else {
hqlQueryBuffer.append(this.getQueryString().substring(start));
}
Query countQuery = ((Session)this.session).createQuery(hqlQueryBuffer.toString());
// SET NAMED PARAMS
Map parameters = this.getNamedParams();
Set parameterNames = parameters.keySet();
Iterator iter = parameterNames.iterator();
while (iter.hasNext()) {
String name = (String)iter.next();
TypedValue typedValue = (TypedValue)parameters.get(name);
countQuery.setParameter(name, typedValue.getValue(), typedValue.getType());
}
// SET POSITIONAL PARAMS
List paramTypes = this.getTypes();
List paramValues = this.getValues();
if (paramTypes != null && paramTypes.size() > 0) {
for (int i = 0; i < paramTypes.size(); i++) {
countQuery.setParameter(i, paramValues.get(i), (Type)paramTypes.get(i));
}
}
int count = ((Long)countQuery.uniqueResult()).intValue();
return count;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy