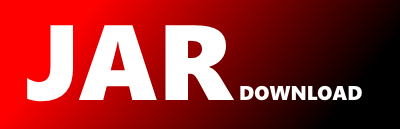
net.snowflake.common.util.Wildcard Maven / Gradle / Ivy
package net.snowflake.common.util;
import java.util.regex.Pattern;
/**
* This class wraps wildcard related functions and regular expression.
*
* @author hyu
*/
public class Wildcard
{
/** Any single character */
private static char SINGLE_CHAR = '_';
/** O or more number of any characters */
private static char MULTIPLE_CHAR = '%';
/** escaped string */
private static char ESCAPED_CHAR = '\\';
private static String NON_ESCAPED_SINGLE_CHAR_REGEX = String.format("(? 0)
{
String strippedBlock = stripEscapedChar(block);
regexBuilder.append(Pattern.quote(strippedBlock));
}
currentIndex += len;
if (currentIndex < wildcardPattern.length())
{
char charToConvert = wildcardPattern.charAt(currentIndex);
assert charToConvert == SINGLE_CHAR || charToConvert == MULTIPLE_CHAR;
regexBuilder.append((charToConvert == SINGLE_CHAR) ? "." : ".*");
currentIndex++;
}
}
/* Deal with the special case that "abc" and "abc_" will both return only one
* String by Pattern.split method (separator.split("abc_") will not return ["abc", ""])
* But interestingly, separator.split("_abc") will return ["", "abc"]
*
* Also, separator.split("%%_") will return empty array.
*/
while (currentIndex < wildcardPattern.length())
{
char charToConvert = wildcardPattern.charAt(currentIndex);
assert charToConvert == SINGLE_CHAR || charToConvert == MULTIPLE_CHAR;
regexBuilder.append((charToConvert == SINGLE_CHAR) ? "." : ".*");
currentIndex ++;
}
return regexBuilder.toString();
}
/**
* Converts a wild card pattern to regex
* @param wildcardPattern a wild card pattern
* @param isCaseSensitive is case insensitive?
* @return a regex pattern
*/
public static Pattern toRegexPattern(String wildcardPattern, boolean isCaseSensitive)
{
if (wildcardPattern == null)
return null;
return isCaseSensitive ?
Pattern.compile(toRegexStr(wildcardPattern)) :
Pattern.compile(toRegexStr(wildcardPattern), Pattern.CASE_INSENSITIVE);
}
/**
* Used to determine if the input string is wildcard pattern
* By wildcard pattern, we means a string contains '%' but not '\%'
* or '_' but not '\_'
* @param inputString a string
* @return true if the string includes a wild card pattern
*/
public static boolean isWildcardPatternStr(String inputString)
{
if (inputString == null)
return false;
return WILDCARD_STRING_PATTERN.matcher(inputString).matches();
}
/**
* A helper function within toRegexStr(String) method.
* For each substring, remove the escaped char '\'
* @param inputString a string
* @return a string without escaped chars
*/
private static String stripEscapedChar(String inputString)
{
StringBuilder sb = new StringBuilder();
boolean escaped = false;
for (int i=0; i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy