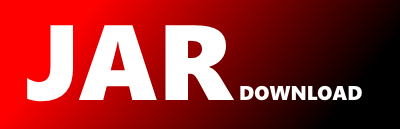
net.snowflake.common.util.GSCommonLogUtil Maven / Gradle / Ivy
/*
* Copyright (c) 2016 Snowflake Computing Inc. All right reserved.
*/
package net.snowflake.common.util;
import java.lang.reflect.InvocationTargetException;
/**
* Simple log utility for GSCommon.
*
* @author mkember
*/
public class GSCommonLogUtil {
/**
* Creates a named logger based on a class name.
*
* @param klass a class.
* @return GSCommon logger instance.
*/
public static GenericGSCommonLogger getLogger(Class klass) {
return getLogger(klass.getName());
}
/**
* Creates a named logger.
*
* Uses reflection to determine if we are in GS or Client. In GS, it uses the org.slf4j.Logger
* wrapper. In Client, it uses the java.util.logging.Logger wrapper instead.
*
* @param name a logger name.
* @return GSCommon logger instance.
*/
public static GenericGSCommonLogger getLogger(String name) {
Class gsClass;
// Check if we are in GS.
try {
gsClass = Class.forName("com.snowflake.util.Slf4jGSCommonLogger");
} catch (ClassNotFoundException ex) {
// We aren't in GS, so use the Java logger.
return new JavaGSCommonLogger(name);
}
// Create an SLF4J logger for GS logging.
try {
Object instance = gsClass.getConstructor(String.class).newInstance(name);
return (GenericGSCommonLogger) instance;
} catch (NoSuchMethodException
| InstantiationException
| IllegalAccessException
| InvocationTargetException ex) {
throw new RuntimeException("Failed to instantiate Slf4jGSCommonLogger: " + ex);
}
}
}