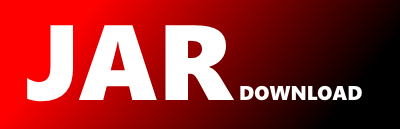
net.snowflake.common.util.Power10 Maven / Gradle / Ivy
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package net.snowflake.common.util;
import java.math.BigInteger;
/** Powers of 10 */
public class Power10 {
// Integer (signed 32 bits)
public static final int intSize = 10;
public static final int[] intTable;
static {
intTable = new int[intSize];
int n = 1;
intTable[0] = n;
for (int i = 1; i < intSize; i++) {
n *= 10;
intTable[i] = n;
}
}
// Long (signed 64 bits)
public static final int longSize = 19;
public static final long[] longTable;
static {
longTable = new long[longSize];
long n = 1;
longTable[0] = n;
for (int i = 1; i < longSize; i++) {
n *= 10;
longTable[i] = n;
}
}
// SB16 (BigInteger)
public static final int sb16Size = 39;
public static final BigInteger[] sb16Table;
static {
sb16Table = new BigInteger[sb16Size];
BigInteger n = BigInteger.ONE;
sb16Table[0] = n;
for (int i = 1; i < sb16Size; i++) {
n = n.multiply(BigInteger.TEN);
sb16Table[i] = n;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy