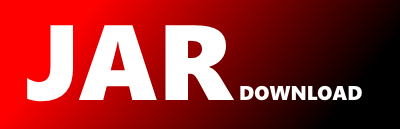
net.snowflake.client.jdbc.SnowflakeConnectString Maven / Gradle / Ivy
/*
* Copyright (c) 2012-2019 Snowflake Computing Inc. All rights reserved.
*/
package net.snowflake.client.jdbc;
import com.google.common.base.Strings;
import java.io.Serializable;
import java.io.UnsupportedEncodingException;
import java.net.URI;
import java.net.URISyntaxException;
import java.net.URLDecoder;
import java.net.URLEncoder;
import java.util.*;
import net.snowflake.client.core.SFSessionProperty;
import net.snowflake.client.log.SFLogger;
import net.snowflake.client.log.SFLoggerFactory;
public class SnowflakeConnectString implements Serializable {
private static final long serialVersionUID = 1L;
static final SFLogger logger = SFLoggerFactory.getLogger(SnowflakeConnectString.class);
private final String scheme;
private final String host;
private final int port;
private final Map parameters;
private final String account;
private static SnowflakeConnectString INVALID_CONNECT_STRING =
new SnowflakeConnectString("", "", -1, Collections.emptyMap(), "");
private static final String PREFIX = "jdbc:snowflake://";
public static boolean hasSupportedPrefix(String url) {
return url.startsWith(PREFIX);
}
public static SnowflakeConnectString parse(String url, Properties info) {
if (url == null) {
logger.debug("Connect strings must be non-null");
return INVALID_CONNECT_STRING;
}
int pos = url.indexOf(PREFIX);
if (pos != 0) {
logger.debug("Connect strings must start with jdbc:snowflake://");
return INVALID_CONNECT_STRING; // not start with jdbc:snowflake://
}
String afterPrefix = url.substring(pos + PREFIX.length());
String scheme;
String host = null;
int port = -1;
Map parameters = new HashMap<>();
try {
URI uri;
if (!afterPrefix.startsWith("http://") && !afterPrefix.startsWith("https://")) {
// not explicitly specified
afterPrefix = url.substring(url.indexOf("snowflake:"));
}
uri = new URI(afterPrefix);
scheme = uri.getScheme();
String authority = uri.getRawAuthority();
String[] hostAndPort = authority.split(":");
if (hostAndPort.length == 2) {
host = hostAndPort[0];
port = Integer.parseInt(hostAndPort[1]);
} else if (hostAndPort.length == 1) {
host = hostAndPort[0];
}
String queryData = uri.getRawQuery();
if (!scheme.equals("snowflake") && !scheme.equals("http") && !scheme.equals("https")) {
logger.debug("Connect strings must have a valid scheme: 'snowflake' or 'http' or 'https'");
return INVALID_CONNECT_STRING;
}
if (Strings.isNullOrEmpty(host)) {
logger.debug("Connect strings must have a valid host: found null or empty host");
return INVALID_CONNECT_STRING;
}
if (port == -1) {
port = 443;
}
String path = uri.getPath();
if (!Strings.isNullOrEmpty(path) && !"/".equals(path)) {
logger.debug("Connect strings must have no path: expecting empty or null or '/'");
return INVALID_CONNECT_STRING;
}
String account = null;
if (!Strings.isNullOrEmpty(queryData)) {
String[] params = queryData.split("&");
for (String p : params) {
String[] keyVals = p.split("=");
if (keyVals.length != 2) {
continue; // ignore invalid pair of parameters.
}
try {
String k = URLDecoder.decode(keyVals[0], "UTF-8");
String v = URLDecoder.decode(keyVals[1], "UTF-8");
if ("ssl".equalsIgnoreCase(k) && !getBooleanTrueByDefault(v)) {
scheme = "http";
} else if ("account".equalsIgnoreCase(k)) {
account = v;
}
parameters.put(k.toUpperCase(Locale.US), v);
} catch (UnsupportedEncodingException ex0) {
logger.info("Failed to decode a parameter {}. Ignored.", p);
}
}
}
if ("snowflake".equals(scheme)) {
scheme = "https"; // by default
}
if (info.size() > 0) {
// NOTE: value in info could be any data type.
// overwrite the properties
for (Map.Entry
© 2015 - 2024 Weber Informatics LLC | Privacy Policy