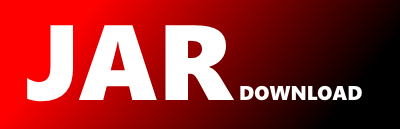
net.snowflake.client.jdbc.SnowflakeConnection Maven / Gradle / Ivy
/*
* Copyright (c) 2012-2019 Snowflake Computing Inc. All rights reserved.
*/
package net.snowflake.client.jdbc;
import java.io.InputStream;
import java.sql.ResultSet;
import java.sql.SQLException;
/** This interface defines Snowflake specific APIs for Connection */
public interface SnowflakeConnection {
/**
* Method to compress data from a stream and upload it at a stage location. The data will be
* uploaded as one file. No splitting is done in this method.
*
* caller is responsible for releasing the inputStream after the method is called.
*
* @param stageName stage name: e.g. ~ or table name or stage name
* @param destPrefix path prefix under which the data should be uploaded on the stage
* @param inputStream input stream from which the data will be uploaded
* @param destFileName destination file name to use
* @param compressData compress data or not before uploading stream
* @throws SQLException failed to compress and put data from a stream at stage
*/
void uploadStream(
String stageName,
String destPrefix,
InputStream inputStream,
String destFileName,
boolean compressData)
throws SQLException;
/**
* Download file from the given stage and return an input stream
*
* @param stageName stage name
* @param sourceFileName file path in stage
* @param decompress true if file compressed
* @return an input stream
* @throws SnowflakeSQLException if any SQL error occurs.
*/
InputStream downloadStream(String stageName, String sourceFileName, boolean decompress)
throws SQLException;
/**
* Return unique session ID from current session generated by making connection
*
* @return a unique alphanumeric value representing current session ID
* @throws SQLException
*/
String getSessionID() throws SQLException;
/**
* Create a new instance of a ResultSet object based off query ID. ResultSet will contain results
* of corresponding query. Used when original ResultSet object is no longer available, such as
* when original connection has been closed.
*
* @param queryID
* @return
* @throws SQLException
*/
ResultSet createResultSet(String queryID) throws SQLException;
/** Returns the SnowflakeConnectionImpl from the connection object. */
SFConnectionHandler getHandler();
}