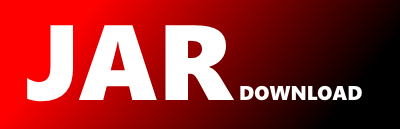
net.snowflake.client.core.SFSqlInput Maven / Gradle / Ivy
/*
* Copyright (c) 2012-2024 Snowflake Computing Inc. All right reserved.
*/
package net.snowflake.client.core;
import java.sql.SQLException;
import java.sql.SQLInput;
import java.util.List;
import java.util.Map;
import java.util.TimeZone;
/** This interface extends the standard {@link SQLInput} interface to provide additional methods. */
@SnowflakeJdbcInternalApi
public interface SFSqlInput extends SQLInput {
/**
* Method unwrapping object of class SQLInput to object of class SfSqlInput.
*
* @param sqlInput SQLInput to consider.
* @return Object unwrapped to SFSqlInput class.
*/
static SFSqlInput unwrap(SQLInput sqlInput) {
return (SFSqlInput) sqlInput;
}
/**
* Reads the next attribute in the stream and returns it as a java.sql.Timestamp
* object.
*
* @param tz timezone to consider.
* @return the attribute; if the value is SQL NULL
, returns null
* @exception SQLException if a database access error occurs
*/
java.sql.Timestamp readTimestamp(TimeZone tz) throws SQLException;
/**
* Reads the next attribute in the stream and returns it as a Object
object.
*
* @param the type of the class modeled by this Class object
* @param type Class representing the Java data type to convert the attribute to.
* @return the attribute at the head of the stream as an {@code Object} in the Java programming
* language;{@code null} if the attribute is SQL {@code NULL}
* @exception SQLException if a database access error occurs
*/
T readObject(Class type, TimeZone tz) throws SQLException;
/**
* Reads the next attribute in the stream and returns it as a List
object.
*
* @param the type of the class modeled by this Class object
* @param type Class representing the Java data type to convert the attribute to.
* @return the attribute at the head of the stream as an {@code List} in the Java programming
* language;{@code null} if the attribute is SQL {@code NULL}
* @exception SQLException if a database access error occurs
*/
List readList(Class type) throws SQLException;
/**
* Reads the next attribute in the stream and returns it as a Map
object.
*
* @param the type of the class modeled by this Class object
* @param type Class representing the Java data type to convert the attribute to.
* @return the attribute at the head of the stream as an {@code Map} in the Java programming
* language;{@code null} if the attribute is SQL {@code NULL}
* @exception SQLException if a database access error occurs
*/
Map readMap(Class type) throws SQLException;
/**
* Reads the next attribute in the stream and returns it as a Array
object.
*
* @param the type of the class modeled by this Class object
* @param type Class representing the Java data type to convert the attribute to.
* @return the attribute at the head of the stream as an {@code Array} in the Java programming
* language;{@code null} if the attribute is SQL {@code NULL}
* @exception SQLException if a database access error occurs
*/
T[] readArray(Class type) throws SQLException;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy