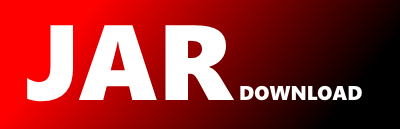
org.apache.axis.message.addressing.handler.AxisClientSideAddressingHandler Maven / Gradle / Ivy
/*
* Copyright (c) 2008 Rodrigo Ruiz
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.axis.message.addressing.handler;
import org.apache.axis.AxisFault;
import org.apache.axis.Handler;
import org.apache.axis.MessageContext;
import org.apache.ws.addressing.handler.ClientSideAddressingHandler;
import org.w3c.dom.Document;
import org.w3c.dom.Element;
import javax.xml.namespace.QName;
import java.util.Hashtable;
import java.util.List;
/**
* Axis-specific client-side WS-Addressing handler.
*
* @author Davanum Srinivas
* @author Ian P. Springer
* @version $Revision: 14 $
*/
public class AxisClientSideAddressingHandler extends ClientSideAddressingHandler
implements Handler {
/**
* serialVersionUID
attribute.
*/
private static final long serialVersionUID = 340258144585488893L;
/**
* Wrapped handler.
*/
private GenericAxisHandler axisHelperHandler = new GenericAxisHandler(this);
/**
* @see org.apache.axis.Handler#init()
*/
public void init() {
axisHelperHandler.init();
}
/**
* @see org.apache.axis.Handler#cleanup()
*/
public void cleanup() {
axisHelperHandler.cleanup();
}
/**
* {@inheritDoc}
*/
public boolean canHandleBlock(QName qname) {
return (axisHelperHandler.canHandleBlock(qname));
}
/**
* {@inheritDoc}
*/
public void setOption(String name, Object value) {
axisHelperHandler.setOption(name, value);
}
/**
* Set a default value for the given option: if the option is not already set,
* then set it. if the option is already set, then do not set it.
*
* If this is called multiple times, the first with a non-null value if 'value'
* will set the default, remaining calls will be ignored.
*
* @param name Option name
* @param value Option default value
* @return true if value set (by this call), otherwise false
*/
public boolean setOptionDefault(String name, Object value) {
return axisHelperHandler.setOptionDefault(name, value);
}
/**
* {@inheritDoc}
*/
public Object getOption(String name) {
return axisHelperHandler.getOption(name);
}
/**
* {@inheritDoc}
*/
public Hashtable, ?> getOptions() {
return axisHelperHandler.getOptions();
}
/**
* {@inheritDoc}
*/
public void setOptions(Hashtable opts) {
axisHelperHandler.setOptions(opts);
}
/**
* {@inheritDoc}
*/
public void setName(String name) {
axisHelperHandler.setName(name);
}
/**
* {@inheritDoc}
*/
public String getName() {
return axisHelperHandler.getName();
}
/**
* {@inheritDoc}
*/
public Element getDeploymentData(Document doc) {
return axisHelperHandler.getDeploymentData(doc);
}
/**
* {@inheritDoc}
*/
public List> getUnderstoodHeaders() {
return axisHelperHandler.getUnderstoodHeaders();
}
/**
* {@inheritDoc}
*/
public void generateWSDL(MessageContext msgContext) throws AxisFault {
axisHelperHandler.generateWSDL(msgContext);
}
/**
* {@inheritDoc}
*/
public void invoke(MessageContext msgContext) throws AxisFault {
axisHelperHandler.invoke(msgContext);
}
/**
* {@inheritDoc}
*/
public void onFault(MessageContext msgContext) {
axisHelperHandler.onFault(msgContext);
}
/**
* {@inheritDoc}
*/
@Override
protected String generateUUId() {
return axisHelperHandler.generateUUId();
}
/**
* {@inheritDoc}
*/
@Override
protected String getSOAPAction(javax.xml.rpc.handler.MessageContext jaxRpcMsgContext) {
MessageContext msgContext = (MessageContext) jaxRpcMsgContext;
return msgContext.getSOAPActionURI();
}
/**
* {@inheritDoc}
*/
@Override
protected void setSOAPAction(javax.xml.rpc.handler.MessageContext jaxRpcMsgContext,
String actionURI) {
MessageContext msgContext = (MessageContext) jaxRpcMsgContext;
msgContext.setUseSOAPAction(true);
msgContext.setSOAPActionURI(actionURI);
}
/**
* {@inheritDoc}
*/
@Override
protected String getEndpointURL(javax.xml.rpc.handler.MessageContext jaxRpcMsgContext) {
return (String) jaxRpcMsgContext
.getProperty(org.apache.axis.MessageContext.TRANS_URL);
}
}