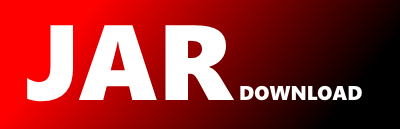
com.mxgraph.shape.mxMarkerRegistry Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of yaoqiang-bpmn-editor Show documentation
Show all versions of yaoqiang-bpmn-editor Show documentation
an Open Source BPMN 2.0 Modeler
package com.mxgraph.shape;
import java.awt.Polygon;
import java.awt.Shape;
import java.awt.geom.Ellipse2D;
import java.awt.geom.Line2D;
import java.util.Hashtable;
import java.util.Map;
import org.yaoqiang.graph.util.Constants;
import com.mxgraph.canvas.mxGraphics2DCanvas;
import com.mxgraph.util.mxConstants;
import com.mxgraph.util.mxPoint;
import com.mxgraph.util.mxUtils;
import com.mxgraph.view.mxCellState;
public class mxMarkerRegistry {
/**
*
*/
protected static Map markers = new Hashtable();
static {
registerMarker(mxConstants.ARROW_CLASSIC, new mxIMarker() {
public mxPoint paintMarker(mxGraphics2DCanvas canvas, mxCellState state, String type, mxPoint pe, double nx, double ny, double size, boolean source) {
Polygon poly = new Polygon();
poly.addPoint((int) Math.round(pe.getX()), (int) Math.round(pe.getY()));
poly.addPoint((int) Math.round(pe.getX() - nx - ny / 2), (int) Math.round(pe.getY() - ny + nx / 2));
poly.addPoint((int) Math.round(pe.getX() - nx * 3 / 4), (int) Math.round(pe.getY() - ny * 3 / 4));
poly.addPoint((int) Math.round(pe.getX() + ny / 2 - nx), (int) Math.round(pe.getY() - ny - nx / 2));
if (mxUtils.isTrue(state.getStyle(), (source) ? "startFill" : "endFill", true)) {
canvas.fillShape(poly);
}
canvas.getGraphics().draw(poly);
return new mxPoint(-nx, -ny);
}
});
mxMarkerRegistry.registerMarker(Constants.ARROW_STYLE_OPEN_BLOCK, new mxIMarker() {
public mxPoint paintMarker(mxGraphics2DCanvas canvas, mxCellState state, String type, mxPoint pe, double nx, double ny, double size, boolean source) {
Polygon poly = new Polygon();
poly.addPoint((int) Math.round(pe.getX()), (int) Math.round(pe.getY()));
poly.addPoint((int) Math.round(pe.getX() - nx - ny / 2), (int) Math.round(pe.getY() - ny + nx / 2));
poly.addPoint((int) Math.round(pe.getX() + ny / 2 - nx), (int) Math.round(pe.getY() - ny - nx / 2));
canvas.getGraphics().draw(poly);
return new mxPoint(-nx, -ny);
}
});
mxMarkerRegistry.registerMarker(mxConstants.ARROW_OPEN, new mxIMarker() {
public mxPoint paintMarker(mxGraphics2DCanvas canvas, mxCellState state, String type, mxPoint pe, double nx, double ny, double size, boolean source) {
canvas.getGraphics().draw(
new Line2D.Float((int) Math.round(pe.getX() - nx - ny / 2), (int) Math.round(pe.getY() - ny + nx / 2), (int) Math.round(pe.getX() - nx
/ 6), (int) Math.round(pe.getY() - ny / 6)));
canvas.getGraphics().draw(
new Line2D.Float((int) Math.round(pe.getX() - nx / 6), (int) Math.round(pe.getY() - ny / 6), (int) Math.round(pe.getX() + ny / 2 - nx),
(int) Math.round(pe.getY() - ny - nx / 2)));
return new mxPoint(-nx / 2, -ny / 2);
}
});
mxMarkerRegistry.registerMarker(Constants.ARROW_STYLE_OPEN_OVAL, new mxIMarker() {
public mxPoint paintMarker(mxGraphics2DCanvas canvas, mxCellState state, String type, mxPoint pe, double nx, double ny, double size, boolean source) {
double cx = pe.getX() - nx / 2;
double cy = pe.getY() - ny / 2;
double a = size / 2;
Shape shape = new Ellipse2D.Double(cx - a, cy - a, size * 1.2, size * 1.2);
canvas.getGraphics().draw(shape);
return new mxPoint(-nx, -ny);
}
});
mxMarkerRegistry.registerMarker(Constants.ARROW_STYLE_OPEN_DIAMOND, new mxIMarker() {
public mxPoint paintMarker(mxGraphics2DCanvas canvas, mxCellState state, String type, mxPoint pe, double nx, double ny, double size, boolean source) {
nx *= 1.8;
ny *= 1.8;
int d = (int) (8 * canvas.getScale());
if (nx > 0 || ny > 0) {
d = (int) (-8 * canvas.getScale());
}
Polygon poly = new Polygon();
if (ny == 0) {
poly.addPoint((int) Math.round(pe.getX() + nx / 2 - d), (int) Math.round(pe.getY()));
poly.addPoint((int) Math.round(pe.getX() - d), (int) Math.round(pe.getY() + nx / 3));
poly.addPoint((int) Math.round(pe.getX() - nx / 2 - d), (int) Math.round(pe.getY()));
poly.addPoint((int) Math.round(pe.getX() - d), (int) Math.round(pe.getY() - nx / 3));
} else {
poly.addPoint((int) Math.round(pe.getX() + ny / 3), (int) Math.round(pe.getY()) - d);
poly.addPoint((int) Math.round(pe.getX()), (int) Math.round(pe.getY() + ny / 2) - d);
poly.addPoint((int) Math.round(pe.getX() - ny / 3), (int) Math.round(pe.getY()) - d);
poly.addPoint((int) Math.round(pe.getX()), (int) Math.round(pe.getY() - ny / 2) - d);
}
canvas.getGraphics().draw(poly);
return new mxPoint(0, 0);
}
});
mxMarkerRegistry.registerMarker(Constants.ARROW_STYLE_SLASH, new mxIMarker() {
public mxPoint paintMarker(mxGraphics2DCanvas canvas, mxCellState state, String type, mxPoint pe, double nx, double ny, double size, boolean source) {
nx *= 1.8;
ny *= 1.8;
int d = (int) (-8 * canvas.getScale());
if (nx > 0 || ny > 0) {
d = (int) (8 * canvas.getScale());
}
Polygon poly = new Polygon();
if (ny == 0) {
poly.addPoint((int) Math.round(pe.getX() - d), (int) Math.round(pe.getY() + nx / 3));
poly.addPoint((int) Math.round(pe.getX() - 2 * d), (int) Math.round(pe.getY() - nx / 3));
poly.addPoint((int) Math.round(pe.getX() - d), (int) Math.round(pe.getY() + nx / 3));
poly.addPoint((int) Math.round(pe.getX() - 2 * d), (int) Math.round(pe.getY() - nx / 3));
} else {
poly.addPoint((int) Math.round(pe.getX() + ny / 3), (int) Math.round(pe.getY()) - d);
poly.addPoint((int) Math.round(pe.getX() - ny / 3), (int) Math.round(pe.getY()) - 2 * d);
poly.addPoint((int) Math.round(pe.getX() + ny / 3), (int) Math.round(pe.getY()) - d);
poly.addPoint((int) Math.round(pe.getX() - ny / 3), (int) Math.round(pe.getY()) - 2 * d);
}
canvas.getGraphics().draw(poly);
return new mxPoint(1, 1);
}
});
}
/**
*
*/
public static mxIMarker getMarker(String name) {
return markers.get(name);
}
/**
*
*/
public static void registerMarker(String name, mxIMarker marker) {
markers.put(name, marker);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy