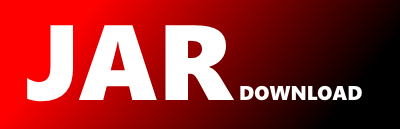
org.yaoqiang.graph.model.YGraphModel Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of yaoqiang-bpmn-editor Show documentation
Show all versions of yaoqiang-bpmn-editor Show documentation
an Open Source BPMN 2.0 Modeler
package org.yaoqiang.graph.model;
import java.awt.Color;
import java.awt.print.PageFormat;
import java.awt.print.Paper;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.List;
import java.util.Map;
import org.yaoqiang.graph.util.Constants;
import com.mxgraph.model.mxGraphModel;
import com.mxgraph.model.mxICell;
/**
* YGraphModel
*
* @author Shi Yaoqiang([email protected])
*/
public class YGraphModel extends mxGraphModel {
private static final long serialVersionUID = 1L;
protected PageFormat pageFormat;
protected Color backgroundColor = Color.WHITE;
protected int pageCount = Integer.parseInt(Constants.SETTINGS.getProperty("pageNumV", "1"));
protected int horizontalPageCount = Integer.parseInt(Constants.SETTINGS.getProperty("pageNumH", "1"));
public PageFormat getPageFormat() {
return pageFormat;
}
public void setPageFormat(PageFormat pageFormat) {
this.pageFormat = pageFormat;
}
public PageFormat setDefaultPageFormat() {
PageFormat pageFormat = new PageFormat();
Paper paper = pageFormat.getPaper();
paper.setSize(Constants.PAGE_HEIGHT, Constants.PAGE_WIDTH);
double margin = 5;
paper.setImageableArea(margin, margin, paper.getWidth() - margin * 2, paper.getHeight() - margin * 2);
pageFormat.setOrientation(Integer.parseInt(Constants.SETTINGS.getProperty("pageOrientation", "0")));
pageFormat.setPaper(paper);
this.pageFormat = pageFormat;
return pageFormat;
}
public int getPageCount() {
return pageCount;
}
public void setPageCount(int pageCount) {
this.pageCount = pageCount;
}
public int getHorizontalPageCount() {
return horizontalPageCount;
}
public void setHorizontalPageCount(int horizontalPageCount) {
this.horizontalPageCount = horizontalPageCount;
}
public Color getBackgroundColor() {
return backgroundColor;
}
public void setBackgroundColor(Color backgroundColor) {
this.backgroundColor = backgroundColor;
}
public List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy