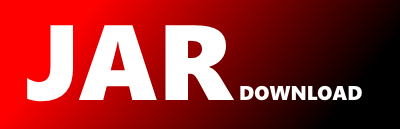
org.yaoqiang.graph.shape.SwimlaneShape Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of yaoqiang-bpmn-editor Show documentation
Show all versions of yaoqiang-bpmn-editor Show documentation
an Open Source BPMN 2.0 Modeler
package org.yaoqiang.graph.shape;
import java.awt.Rectangle;
import org.yaoqiang.graph.util.Constants;
import com.mxgraph.canvas.mxGraphics2DCanvas;
import com.mxgraph.shape.mxBasicShape;
import com.mxgraph.util.mxConstants;
import com.mxgraph.util.mxRectangle;
import com.mxgraph.util.mxUtils;
import com.mxgraph.view.mxCellState;
/**
* SwimlaneShape
*
* @author Shi Yaoqiang([email protected])
*/
public class SwimlaneShape extends mxBasicShape {
public void paintShape(mxGraphics2DCanvas canvas, mxCellState state) {
int start = (int) Math.round(mxUtils.getInt(state.getStyle(), mxConstants.STYLE_STARTSIZE, mxConstants.DEFAULT_STARTSIZE) * canvas.getScale());
Rectangle tmp = state.getRectangle();
if (mxUtils.isTrue(state.getStyle(), mxConstants.STYLE_HORIZONTAL, true)) {
if (configureGraphics(canvas, state, true)) {
if (mxUtils.isTrue(state.getStyle(), Constants.SWIMLANE_STYLE_LANE, false)) {
canvas.fillShape(new Rectangle(tmp.x, tmp.y, tmp.width, tmp.height));
} else {
canvas.fillShape(new Rectangle(tmp.x, tmp.y, tmp.width, Math.min(tmp.height, start)));
}
}
if (configureGraphics(canvas, state, false)) {
if (mxUtils.isTrue(state.getStyle(), Constants.SWIMLANE_STYLE_LANE, false)) {
//canvas.getGraphics().drawLine(tmp.x, tmp.y, tmp.x + tmp.width, tmp.y);
canvas.getGraphics().drawRect(tmp.x, tmp.y, tmp.width, tmp.height);
} else {
canvas.getGraphics().drawRect(tmp.x, tmp.y, tmp.width, Math.min(tmp.height, start));
canvas.getGraphics().drawRect(tmp.x, tmp.y + start, tmp.width, tmp.height - start);
}
}
} else {
if (configureGraphics(canvas, state, true)) {
if (mxUtils.isTrue(state.getStyle(), Constants.SWIMLANE_STYLE_LANE, false)) {
canvas.fillShape(new Rectangle(tmp.x, tmp.y, tmp.width, tmp.height));
} else {
canvas.fillShape(new Rectangle(tmp.x, tmp.y, Math.min(tmp.width, start), tmp.height));
}
}
if (configureGraphics(canvas, state, false)) {
if (mxUtils.isTrue(state.getStyle(), Constants.SWIMLANE_STYLE_LANE, false)) {
//canvas.getGraphics().drawLine(tmp.x, tmp.y, tmp.x + tmp.width, tmp.y);
canvas.getGraphics().drawRect(tmp.x, tmp.y, tmp.width, tmp.height);
} else {
canvas.getGraphics().drawRect(tmp.x, tmp.y, Math.min(tmp.width, start), tmp.height);
canvas.getGraphics().drawRect(tmp.x + start, tmp.y, tmp.width - start, tmp.height);
}
}
}
if (mxUtils.isTrue(state.getStyle(), Constants.ACTIVITY_STYLE_LOOP_MI, false)) {
double scale = canvas.getScale();
double imgWidth = 16 * scale;
double imgHeight = 16 * scale;
Rectangle imageBounds = state.getRectangle();
imageBounds.setRect(imageBounds.getX() + (imageBounds.getWidth() - imgWidth) / 2, imageBounds.getY() + imageBounds.getHeight() - imgHeight,
imgWidth, imgHeight);
canvas.drawImage(imageBounds, canvas.getImageForStyle(state.getStyle()));
}
}
protected mxRectangle getGradientBounds(mxGraphics2DCanvas canvas, mxCellState state) {
int start = (int) Math.round(mxUtils.getInt(state.getStyle(), mxConstants.STYLE_STARTSIZE, mxConstants.DEFAULT_STARTSIZE) * canvas.getScale());
mxRectangle result = new mxRectangle(state);
if (mxUtils.isTrue(state.getStyle(), mxConstants.STYLE_HORIZONTAL, true)) {
result.setHeight(Math.min(result.getHeight(), start));
} else {
result.setWidth(Math.min(result.getWidth(), start));
}
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy