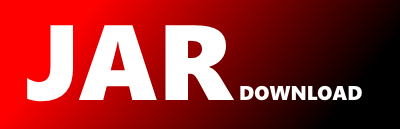
org.yaoqiang.graph.action.GraphActions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of yaoqiang-bpmn-editor Show documentation
Show all versions of yaoqiang-bpmn-editor Show documentation
an Open Source BPMN 2.0 Modeler
package org.yaoqiang.graph.action;
import java.awt.Color;
import java.awt.Component;
import java.awt.event.ActionEvent;
import java.awt.print.PageFormat;
import java.awt.print.PrinterException;
import java.awt.print.PrinterJob;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
import javax.swing.AbstractAction;
import javax.swing.JColorChooser;
import javax.swing.JOptionPane;
import javax.swing.TransferHandler;
import org.yaoqiang.graph.layout.BPMNLayout;
import org.yaoqiang.graph.model.YGraphModel;
import org.yaoqiang.graph.swing.YGraphComponent;
import org.yaoqiang.graph.util.Constants;
import org.yaoqiang.graph.util.Utils;
import org.yaoqiang.graph.view.YGraph;
import com.mxgraph.layout.mxIGraphLayout;
import com.mxgraph.model.mxCell;
import com.mxgraph.model.mxGeometry;
import com.mxgraph.model.mxGraphModel;
import com.mxgraph.model.mxICell;
import com.mxgraph.swing.util.mxMorphing;
import com.mxgraph.util.mxConstants;
import com.mxgraph.util.mxEvent;
import com.mxgraph.util.mxEventObject;
import com.mxgraph.util.mxEventSource.mxIEventListener;
import com.mxgraph.util.mxResources;
import com.mxgraph.util.mxUtils;
/**
* GraphActions
*
* @author Shi Yaoqiang([email protected])
*/
public class GraphActions extends AbstractAction {
private static final long serialVersionUID = 1L;
public static final int ZOOM = 001;
public static final int ZOOM_IN = 002;
public static final int ZOOM_OUT = 003;
public static final int ZOOM_ACTUAL = 004;
public static final int ZOOM_FIT_PAGE = 005;
public static final int ZOOM_FIT_WIDTH = 006;
public static final int ZOOM_CUSTOM = 007;
public static final int EDIT = 101;
public static final int PASTE = 102;
public static final int DUPLICATE = 103;
public static final int DELETE = 104;
public static final int PAGE_SETUP = 105;
public static final int PRINT = 106;
public static final int SELECT_ALL = 201;
public static final int SELECT_NONE = 202;
public static final int SELECT_VERTICES = 203;
public static final int SELECT_EDGES = 204;
public static final int SELECT_PREVIOUS = 205;
public static final int SELECT_NEXT = 206;
public static final int SELECT_PARENT = 207;
public static final int SELECT_CHILD = 208;
public static final int ALIGN_LEFT = 301;
public static final int ALIGN_CENTER = 302;
public static final int ALIGN_RIGHT = 303;
public static final int ALIGN_TOP = 304;
public static final int ALIGN_MIDDLE = 305;
public static final int ALIGN_BOTTOM = 306;
public static final int DISTRIBUTE_HORIZONTALLY = 307;
public static final int DISTRIBUTE_VERTICALLY = 308;
public static final int SAME = 309;
public static final int SAME_HEIGHT = 310;
public static final int SAME_WIDTH = 311;
public static final int MOVE_UP = 312;
public static final int MOVE_DOWN = 313;
public static final int MOVE_RIGHT = 314;
public static final int MOVE_LEFT = 315;
public static final int BACKGROUND = 408;
public static final int ADD_PAGE = 409;
public static final int REMOVE_PAGE = 410;
public static final int DEBUG_STYLE = 500;
public static final int FOLD_CELLS = 502;
public static final int ROTATE_SWIMLANE = 503;
public static final int MOVE_LANE = 504;
public static final int PROMPT_VALUE = 508;
public static final int TOGGLE = 509;
public static final int STYLE = 510;
public static final int INIT_PART = 511;
public static final int MULTI_PART = 512;
public static final int ADD_PART = 513;
public static final int DEL_PART = 514;
public static final int SWAP_PART = 515;
public static final int DEF_SF = 516;
public static final int RESET_BACKGROUND = 517;
public static final int AUTO_LAYOUT = 518;
public static final int HOME = 701;
public static final int ENTER_GROUP = 702;
public static final int EXIT_GROUP = 703;
public static final int GROUP = 704;
public static final int UNGROUP = 705;
private int type;
private boolean booleanValue;
private double doubleValue;
private String stringValue;
private String stringValue2;
private Object objectValue;
private Object objectValue2;
public GraphActions(int type) {
this.type = type;
}
public static GraphActions getAction(int type) {
return new GraphActions(type);
}
public static GraphActions getScaleAction(double value) {
return new GraphActions(ZOOM).setDoubleValue(value);
}
public static GraphActions getMoveLaneAction(double value) {
return new GraphActions(MOVE_LANE).setDoubleValue(value);
}
public static GraphActions getAddPageAction(boolean horizontal) {
return new GraphActions(ADD_PAGE).setBooleanValue(horizontal);
}
public static GraphActions getRemovePageAction(boolean horizontal) {
return new GraphActions(REMOVE_PAGE).setBooleanValue(horizontal);
}
public static GraphActions getPromptValueAction(String key, String message) {
return new GraphActions(PROMPT_VALUE).setStringValue(key).setStringValue2(message);
}
public static GraphActions getToggleAction(String key) {
return getToggleAction(key, false);
}
public static GraphActions getToggleAction(String key, boolean defaultValue) {
return new GraphActions(TOGGLE).setStringValue(key).setBooleanValue(defaultValue);
}
public static GraphActions getStyleAction(String value) {
return new GraphActions(STYLE).setStringValue(value);
}
public static GraphActions getStyleAction(Object cell, String value) {
return new GraphActions(STYLE).setObjectValue(cell).setStringValue(value);
}
public static GraphActions getInitPartAction(Object parent, String id) {
return new GraphActions(INIT_PART).setObjectValue(parent).setStringValue(id);
}
public static GraphActions getMultiPartAction(String id, String multi) {
return new GraphActions(MULTI_PART).setStringValue(id).setStringValue2(multi);
}
public static GraphActions getAddPartAction(Object parent, boolean toTop) {
return new GraphActions(ADD_PART).setObjectValue(parent).setBooleanValue(toTop);
}
public static GraphActions getDelPartAction(Object parent, Object cell) {
return new GraphActions(DEL_PART).setObjectValue(parent).setObjectValue2(cell);
}
public static GraphActions getSwapPartAction() {
return new GraphActions(SWAP_PART);
}
public static GraphActions getDefaultSequenceFlowAction(Object cell, Object edge) {
return new GraphActions(DEF_SF).setObjectValue(cell).setObjectValue2(edge);
}
public static GraphActions getResetBackgroundAction() {
return new GraphActions(RESET_BACKGROUND);
}
public static GraphActions getAutoLayoutAction() {
return new GraphActions(AUTO_LAYOUT);
}
public static GraphActions getAutoLayoutAction(String value) {
return new GraphActions(AUTO_LAYOUT).setStringValue(value);
}
public void actionPerformed(ActionEvent e) {
YGraphComponent graphComponent = (YGraphComponent) e.getSource();
final YGraph graph = graphComponent.getGraph();
YGraphModel model = graph.getModel();
Object cell = graph.getSelectionCell();
if (type == ZOOM) {
if (doubleValue > 0) {
graphComponent.zoomTo(doubleValue, graphComponent.isCenterZoom());
}
} else if (type == ZOOM_IN) {
graphComponent.zoomIn();
} else if (type == ZOOM_OUT) {
graphComponent.zoomOut();
} else if (type == ZOOM_ACTUAL) {
graphComponent.zoomActual();
} else if (type == ZOOM_FIT_PAGE) {
graphComponent.setZoomPolicy(YGraphComponent.ZOOM_POLICY_PAGE);
} else if (type == ZOOM_FIT_WIDTH) {
graphComponent.setZoomPolicy(YGraphComponent.ZOOM_POLICY_WIDTH);
} else if (type == ZOOM_CUSTOM) {
double scale = 0;
String value = (String) JOptionPane.showInputDialog(graphComponent, mxResources.get("value"), mxResources.get("scale") + " (%)",
JOptionPane.PLAIN_MESSAGE, null, null, "100");
if (value != null) {
scale = Double.parseDouble(value.replace("%", "")) / 100;
}
if (scale > 0) {
graphComponent.zoomTo(scale, graphComponent.isCenterZoom());
}
} else if (type == EDIT) {
graphComponent.startEditing();
} else if (type == PASTE) {
graphComponent.setPasteToPoint(graphComponent.getPopupPoint());
TransferHandler.getPasteAction().actionPerformed(e);
graphComponent.setPasteToPoint(null);
} else if (type == DUPLICATE) {
TransferHandler.getCopyAction().actionPerformed(e);
TransferHandler.getPasteAction().actionPerformed(e);
} else if (type == DELETE) {
graph.removeCells();
} else if (type == PAGE_SETUP) {
PrinterJob pj = PrinterJob.getPrinterJob();
PageFormat format = pj.pageDialog(graphComponent.getPageFormat());
if (format != null) {
Constants.SWIMLANE_WIDTH = (int) (format.getWidth() * 1.25 + (model.getHorizontalPageCount() - 1)
* (Constants.SWIMLANE_START_POINT + format.getWidth() * 1.25));
Constants.SWIMLANE_HEIGHT = (int) (format.getHeight() * 1.2 + (model.getPageCount() - 1)
* (Constants.SWIMLANE_START_POINT + format.getHeight() * 1.2));
graphComponent.setPageFormat(format);
model.setPageFormat(format);
Utils.arrangeAllSwimlaneLength(graph, true);
graphComponent.zoomAndCenter();
}
} else if (type == PRINT) {
PrinterJob pj = PrinterJob.getPrinterJob();
if (pj.printDialog()) {
PageFormat pf = graphComponent.getPageFormat();
pj.setPrintable(graphComponent, pf);
try {
pj.print();
} catch (PrinterException e2) {
System.out.println(e2);
}
}
} else if (type == SELECT_ALL) {
graph.selectAll();
} else if (type == SELECT_NONE) {
graph.clearSelection();
} else if (type == SELECT_VERTICES) {
graph.selectVertices();
} else if (type == SELECT_EDGES) {
graph.selectEdges();
} else if (type == SELECT_PREVIOUS) {
graph.selectPreviousCell();
} else if (type == SELECT_NEXT) {
graph.selectNextCell();
} else if (type == SELECT_PARENT) {
graph.selectParentCell();
} else if (type == SELECT_CHILD) {
graph.selectChildCell();
} else if (type == MOVE_UP) {
graph.moveCells("up");
} else if (type == MOVE_DOWN) {
graph.moveCells("down");
} else if (type == MOVE_RIGHT) {
graph.moveCells("right");
} else if (type == MOVE_LEFT) {
graph.moveCells("left");
} else if (type == ALIGN_LEFT) {
graph.alignCells(mxConstants.ALIGN_LEFT);
} else if (type == ALIGN_CENTER) {
graph.alignCells(mxConstants.ALIGN_CENTER);
} else if (type == ALIGN_RIGHT) {
graph.alignCells(mxConstants.ALIGN_RIGHT);
} else if (type == ALIGN_TOP) {
graph.alignCells(mxConstants.ALIGN_TOP);
} else if (type == ALIGN_MIDDLE) {
graph.alignCells(mxConstants.ALIGN_MIDDLE);
} else if (type == ALIGN_BOTTOM) {
graph.alignCells(mxConstants.ALIGN_BOTTOM);
} else if (type == DISTRIBUTE_HORIZONTALLY) {
graph.distributeCells(mxConstants.ALIGN_CENTER);
} else if (type == DISTRIBUTE_VERTICALLY) {
graph.distributeCells(mxConstants.ALIGN_MIDDLE);
} else if (type == SAME) {
graph.sameCells("");
} else if (type == SAME_HEIGHT) {
graph.sameCells(Constants.HEIGHT);
} else if (type == SAME_WIDTH) {
graph.sameCells(Constants.WIDTH);
} else if (type == BACKGROUND) {
Color newColor = JColorChooser.showDialog(graphComponent, mxResources.get("background"), null);
if (newColor != null) {
graphComponent.getViewport().setOpaque(true);
graphComponent.getViewport().setBackground(newColor);
graphComponent.getGraph().getModel().setBackgroundColor(newColor);
}
graphComponent.repaint();
} else if (type == ADD_PAGE || type == REMOVE_PAGE) {
int verticalCount = graphComponent.getVerticalPageCount();
int horizontalCount = graphComponent.getHorizontalPageCount();
if (type == ADD_PAGE) {
if (booleanValue) {
graphComponent.setHorizontalPageCount(horizontalCount + 1);
} else {
graphComponent.setVerticalPageCount(verticalCount + 1);
}
} else {
if (booleanValue) {
if (horizontalCount > 1)
graphComponent.setHorizontalPageCount(horizontalCount - 1);
} else {
if (verticalCount > 1)
graphComponent.setVerticalPageCount(verticalCount - 1);
}
}
if (booleanValue) {
model.setHorizontalPageCount(graphComponent.getHorizontalPageCount());
} else {
model.setPageCount(graphComponent.getVerticalPageCount());
}
PageFormat format = model.getPageFormat();
Constants.SWIMLANE_WIDTH = (int) (format.getWidth() * 1.25 + (model.getHorizontalPageCount() - 1)
* (Constants.SWIMLANE_START_POINT + format.getWidth() * 1.25));
Constants.SWIMLANE_HEIGHT = (int) (format.getHeight() * 1.2 + (model.getPageCount() - 1)
* (Constants.SWIMLANE_START_POINT + format.getHeight() * 1.2));
Utils.arrangeAllSwimlaneLength(graphComponent.getGraph(), true);
graphComponent.zoomAndCenter();
} else if (type == DEBUG_STYLE) {
String initial = graph.getModel().getStyle(cell);
String value = (String) JOptionPane.showInputDialog(graphComponent, mxResources.get("style"), mxResources.get("style"), JOptionPane.PLAIN_MESSAGE,
null, null, initial);
if (value != null) {
graph.setCellStyle(value);
}
} else if (type == FOLD_CELLS) {
if (graph != null && graph.getSelectionCount() == 1) {
if (graph.isSubChoreography(cell)) {
cell = Utils.getChoreographyActivity(graph, cell);
}
graph.foldCells(!graph.isCellCollapsed(cell), false, new Object[] { cell });
}
} else if (type == ROTATE_SWIMLANE) {
Utils.rotateSwimlane(graphComponent);
} else if (type == HOME) {
graph.home();
graph.fireEvent(new mxEventObject(Constants.EXIT_GROUP, "cell", cell));
} else if (type == ENTER_GROUP) {
graph.enterGroup();
graph.fireEvent(new mxEventObject(Constants.ENTER_GROUP, "cell", cell));
} else if (type == EXIT_GROUP) {
graph.exitGroup();
graph.fireEvent(new mxEventObject(Constants.EXIT_GROUP, "cell", cell));
} else if (type == GROUP) {
graph.setSelectionCell(graph.groupCells(null, 20));
} else if (type == UNGROUP) {
graph.ungroupCells();
} else if (type == TOGGLE) {
graph.toggleCellStyles(stringValue, booleanValue);
} else if (type == PROMPT_VALUE) {
String value = (String) JOptionPane.showInputDialog((Component) e.getSource(), mxResources.get("value"), stringValue2, JOptionPane.PLAIN_MESSAGE,
null, null, "");
if (value != null) {
if (value.equals(mxConstants.NONE)) {
value = null;
}
graph.setCellStyles(stringValue, value);
}
} else if (type == DEF_SF) {
model.beginUpdate();
if (objectValue2 != null) {
model.setStyle(objectValue2, Constants.EDGE_TYPE_DEFAULT_SEQUENCE_FLOW);
}
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy