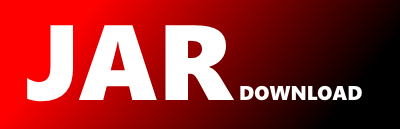
org.yaoqiang.graph.io.YModelCodec Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of yaoqiang-bpmn-editor Show documentation
Show all versions of yaoqiang-bpmn-editor Show documentation
an Open Source BPMN 2.0 Modeler
package org.yaoqiang.graph.io;
import java.awt.Color;
import java.awt.print.PageFormat;
import java.awt.print.Paper;
import java.util.Map;
import org.w3c.dom.Element;
import org.w3c.dom.NamedNodeMap;
import org.w3c.dom.Node;
import org.yaoqiang.graph.model.YGraphModel;
import org.yaoqiang.graph.util.Constants;
import com.mxgraph.io.mxCodec;
import com.mxgraph.io.mxCodecRegistry;
import com.mxgraph.io.mxObjectCodec;
import com.mxgraph.model.mxICell;
import com.mxgraph.util.mxUtils;
/**
* YModelCodec
*
* @author Shi Yaoqiang([email protected])
*/
public class YModelCodec extends mxObjectCodec {
/**
* Constructs a new model codec.
*/
public YModelCodec()
{
this(new YGraphModel());
}
/**
* Constructs a new model codec for the given template.
*/
public YModelCodec(Object template)
{
this(template, null, null, null);
}
/**
* Constructs a new model codec for the given arguments.
*/
public YModelCodec(Object template, String[] exclude, String[] idrefs,
Map mapping)
{
super(template, exclude, idrefs, mapping);
}
/**
* Encodes the given YGraphModel by writing a (flat) XML sequence
* of cell nodes as produced by the mxCellCodec. The sequence is
* wrapped-up in a node with the name root.
*/
public Node encode(mxCodec enc, Object obj) {
Node node = null;
if (obj instanceof YGraphModel) {
YGraphModel model = (YGraphModel) obj;
String name = mxCodecRegistry.getName(obj);
node = enc.getDocument().createElement(name);
// ==============start==============
PageFormat pageFormat = model.getPageFormat();
Node pageNode = enc.getDocument().createElement("page");
mxCodec.setAttribute(pageNode, "background", mxUtils.hexString(model.getBackgroundColor()));
mxCodec.setAttribute(pageNode, "count", model.getPageCount());
mxCodec.setAttribute(pageNode, "horizontalcount", model.getHorizontalPageCount());
mxCodec.setAttribute(pageNode, "orientation", pageFormat.getOrientation());
mxCodec.setAttribute(pageNode, "width", pageFormat.getWidth());
mxCodec.setAttribute(pageNode, "height", pageFormat.getHeight());
node.appendChild(pageNode);
// ==============end================
Node rootNode = enc.getDocument().createElement("root");
enc.encodeCell((mxICell) model.getRoot(), rootNode, true);
node.appendChild(rootNode);
}
return node;
}
/**
* Reads the cells into the graph model. All cells are children of the root
* element in the node.
*/
public Node beforeDecode(mxCodec dec, Node node, Object into)
{
if (node instanceof Element)
{
Element elt = (Element) node;
YGraphModel model = null;
if (into instanceof YGraphModel)
{
model = (YGraphModel) into;
}
else
{
model = new YGraphModel();
}
// ==============start==============
Node pageNode = elt.getElementsByTagName("page").item(0);
if (pageNode != null) {
NamedNodeMap attrs = pageNode.getAttributes();
if (attrs.getNamedItem("background") != null) {
Color backgroundColor = mxUtils.parseColor(attrs.getNamedItem("background").getNodeValue());
model.setBackgroundColor(backgroundColor);
}
model.setPageCount(Integer.parseInt(attrs.getNamedItem("count").getNodeValue()));
if (attrs.getNamedItem("horizontalcount") != null) {
model.setHorizontalPageCount(Integer.parseInt(attrs.getNamedItem("horizontalcount").getNodeValue()));
}
PageFormat pageFormat = new PageFormat();
int orientation = Integer.parseInt(attrs.getNamedItem("orientation").getNodeValue());
double width = Double.parseDouble(attrs.getNamedItem("width").getNodeValue());
double height = Double.parseDouble(attrs.getNamedItem("height").getNodeValue());
pageFormat.setOrientation(orientation);
Paper paper = new Paper();
if (orientation == PageFormat.PORTRAIT) {
paper.setSize(width, height);
} else {
paper.setSize(height, width);
}
pageFormat.setPaper(paper);
model.setPageFormat(pageFormat);
pageNode.getParentNode().removeChild(pageNode);
Constants.SWIMLANE_WIDTH = (int) (model.getPageFormat().getWidth() * 1.25 + (model.getHorizontalPageCount() - 1)
* (Constants.SWIMLANE_START_POINT + model.getPageFormat().getWidth() * 1.25));
Constants.SWIMLANE_HEIGHT = (int) (model.getPageFormat().getHeight() * 1.2 + (model.getPageCount() - 1)
* (Constants.SWIMLANE_START_POINT + model.getPageFormat().getHeight() * 1.2));
}
// ==============end================
// Reads the cells into the graph model. All cells
// are children of the root element in the node.
Node root = elt.getElementsByTagName("root").item(0);
mxICell rootCell = null;
if (root != null)
{
Node tmp = root.getFirstChild();
while (tmp != null)
{
mxICell cell = dec.decodeCell(tmp, true);
if (cell != null && cell.getParent() == null)
{
rootCell = cell;
}
tmp = tmp.getNextSibling();
}
root.getParentNode().removeChild(root);
}
// Sets the root on the model if one has been decoded
if (rootCell != null)
{
model.setRoot(rootCell);
}
}
return node;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy