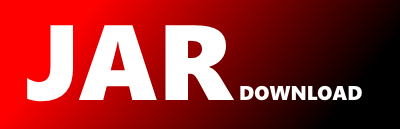
org.yaoqiang.graph.shape.SubProcessShape Maven / Gradle / Ivy
package org.yaoqiang.graph.shape;
import java.awt.BasicStroke;
import java.awt.Color;
import java.awt.Graphics2D;
import java.awt.Rectangle;
import java.util.Map;
import org.yaoqiang.graph.util.Constants;
import com.mxgraph.canvas.mxGraphics2DCanvas;
import com.mxgraph.shape.mxRectangleShape;
import com.mxgraph.util.mxConstants;
import com.mxgraph.util.mxUtils;
import com.mxgraph.view.mxCellState;
/**
* SubProcessShape
*
* @author Shi Yaoqiang([email protected])
*/
public class SubProcessShape extends mxRectangleShape {
public void paintShape(mxGraphics2DCanvas canvas, mxCellState state) {
Map style = state.getStyle();
double scale = canvas.getScale();
Rectangle rect = state.getRectangle();
String type = mxUtils.getString(style, Constants.STYLE_TYPE);
// Event Sub-Process
if (type.equals(Constants.SUBPROCESS_STYLE_EVENT)) {
style.put(mxConstants.STYLE_DASHED, "1");
canvas.getGraphics().setStroke(canvas.createStroke(style));
} else {
style.put(mxConstants.STYLE_DASHED, "0");
canvas.getGraphics().setStroke(canvas.createStroke(style));
}
super.paintShape(canvas, state);
if (type.equals(Constants.SUBPROCESS_STYLE_EVENT)) {
String trigger = mxUtils.getString(style, Constants.STYLE_TRIGGER, "");
if (trigger.length() != 0 && (rect.width <= Constants.FOLDED_SUBPROCESS_WIDTH * scale + 1)) {
float pw = (float) (mxUtils.getFloat(style, mxConstants.STYLE_STROKEWIDTH, 1) * scale);
Graphics2D g = canvas.getGraphics();
Color color = getStrokeColor(canvas, state);
g.setColor(color);
if (trigger.lastIndexOf("NonInterrupting") == -1) {
g.setStroke(new BasicStroke(pw));
}
int x = rect.x + 2;
int y = rect.y + 2;
int w = (int) (25 * scale);
int h = (int) (25 * scale);
g.drawOval(x, y, w, h);
String img = "";
if (trigger.startsWith("Message")) {
img = "/org/yaoqiang/graph/images/event_message.png";
} else if (trigger.startsWith("Timer")) {
img = "/org/yaoqiang/graph/images/event_timer.png";
} else if (trigger.startsWith("Conditional")) {
img = "/org/yaoqiang/graph/images/event_conditional.png";
} else if (trigger.startsWith("Signal")) {
img = "/org/yaoqiang/graph/images/event_signal.png";
} else if (trigger.startsWith("Multiple")) {
img = "/org/yaoqiang/graph/images/event_multiple.png";
} else if (trigger.startsWith("ParallelMultiple")) {
img = "/org/yaoqiang/graph/images/event_parallelmultiple.png";
} else if (trigger.startsWith("Escalation")) {
img = "/org/yaoqiang/graph/images/event_escalation.png";
} else if (trigger.startsWith("Error")) {
img = "/org/yaoqiang/graph/images/event_error.png";
} else if (trigger.startsWith("Compensation")) {
img = "/org/yaoqiang/graph/images/event_compensation.png";
}
canvas.drawImage(new Rectangle(x + (int) (3 * scale),y + (int) (3 * scale),(int) (20 * scale),(int) (20 * scale)) , img);
}
}
// Transaction Sub-Process
if (type.equals(Constants.SUBPROCESS_STYLE_TRANSACTION)) {
int inset = (int) Math.round((mxUtils.getFloat(style, mxConstants.STYLE_STROKEWIDTH, 1) + 3) * canvas.getScale());
int x = rect.x + inset;
int y = rect.y + inset;
int w = rect.width - 2 * inset;
int h = rect.height - 2 * inset;
canvas.getGraphics().drawRoundRect(x, y, w, h, Constants.RECTANGLE_ARCSIZE - 2, Constants.RECTANGLE_ARCSIZE - 2);
}
int imgWidth = (int) (32 * scale);
int imgHeight = (int) (16 * scale);
Rectangle imageBounds = state.getRectangle();
String marker = canvas.getImageForStyle(style);
String loop = mxUtils.getString(style, Constants.STYLE_LOOP_IMAGE, " ");
String compensation = mxUtils.getString(style, Constants.STYLE_COMPENSATION_IMAGE, " ");
String adHoc = mxUtils.getString(style, Constants.STYLE_ADHOC_IMAGE, " ");
if (!mxUtils.isTrue(style, Constants.STYLE_CALL, false) && (rect.width > Constants.FOLDED_SUBPROCESS_WIDTH * scale + 1)) { // expanded
if (type.equals(Constants.SUBPROCESS_STYLE_ADHOC)) {
if (!loop.equals(" ") || !compensation.equals(" ")) {
if (!loop.equals(" ") && !compensation.equals(" ")) {
imageBounds.setRect(imageBounds.getX() + (2 * imageBounds.getWidth() - 3 * imgWidth) / 4, imageBounds.getY() + imageBounds.getHeight()
- imgHeight, imgWidth / 2, imgHeight);
canvas.drawImage(imageBounds, loop);
imageBounds.setRect(imageBounds.getX() + imgWidth / 2, imageBounds.getY() + imageBounds.getHeight() - imgHeight, imgWidth / 2,
imgHeight);
canvas.drawImage(imageBounds, compensation);
imageBounds.setRect(imageBounds.getX() + imgWidth / 2, imageBounds.getY() + imageBounds.getHeight() - imgHeight, imgWidth / 2,
imgHeight);
canvas.drawImage(imageBounds, adHoc);
} else {
imageBounds.setRect(imageBounds.getX() + (imageBounds.getWidth() - imgWidth) / 2, imageBounds.getY() + imageBounds.getHeight()
- imgHeight, imgWidth / 2, imgHeight);
if (!loop.equals(" ")) {
canvas.drawImage(imageBounds, loop);
} else {
canvas.drawImage(imageBounds, compensation);
}
imageBounds.setRect(imageBounds.getX() + imgWidth / 2, imageBounds.getY() + imageBounds.getHeight() - imgHeight, imgWidth / 2,
imgHeight);
canvas.drawImage(imageBounds, adHoc);
}
} else {
imageBounds.setRect(imageBounds.getX() + (2 * imageBounds.getWidth() - imgWidth) / 4, imageBounds.getY() + imageBounds.getHeight()
- imgHeight, imgWidth / 2, imgHeight);
canvas.drawImage(imageBounds, adHoc);
}
} else {
if (!loop.equals(" ") || !compensation.equals(" ")) {
if (!loop.equals(" ") && !compensation.equals(" ")) {
imageBounds.setRect(imageBounds.getX() + (imageBounds.getWidth() - imgWidth) / 2, imageBounds.getY() + imageBounds.getHeight()
- imgHeight, imgWidth / 2, imgHeight);
canvas.drawImage(imageBounds, loop);
imageBounds.setRect(imageBounds.getX() + imgWidth / 2, imageBounds.getY() + imageBounds.getHeight() - imgHeight, imgWidth / 2,
imgHeight);
canvas.drawImage(imageBounds, compensation);
} else {
imageBounds.setRect(imageBounds.getX() + (2 * imageBounds.getWidth() - imgWidth) / 4, imageBounds.getY() + imageBounds.getHeight()
- imgHeight, imgWidth / 2, imgHeight);
if (!loop.equals(" ")) {
canvas.drawImage(imageBounds, loop);
} else {
canvas.drawImage(imageBounds, compensation);
}
}
}
}
} else { // Call Activity (Process) or Collapsed Sub-Process
if (type.equals(Constants.SUBPROCESS_STYLE_ADHOC)) {
if (!loop.equals(" ") || !compensation.equals(" ")) {
if (!loop.equals(" ") && !compensation.equals(" ")) {
imageBounds.setRect(imageBounds.getX() + (imageBounds.getWidth() / 2 - imgWidth), imageBounds.getY() + imageBounds.getHeight()
- imgHeight, imgWidth / 2, imgHeight);
canvas.drawImage(imageBounds, loop);
imageBounds.setRect(imageBounds.getX() + imgWidth / 2, imageBounds.getY() + imageBounds.getHeight() - imgHeight, imgWidth / 2,
imgHeight);
canvas.drawImage(imageBounds, compensation);
imageBounds.setRect(imageBounds.getX() + imgWidth / 2, imageBounds.getY() + imageBounds.getHeight() - imgHeight, imgWidth / 2,
imgHeight);
canvas.drawImage(imageBounds, marker);
imageBounds.setRect(imageBounds.getX() + imgWidth / 2, imageBounds.getY() + imageBounds.getHeight() - imgHeight, imgWidth / 2,
imgHeight);
canvas.drawImage(imageBounds, adHoc);
} else {
imageBounds.setRect(imageBounds.getX() + (2 * imageBounds.getWidth() - 3 * imgWidth) / 4, imageBounds.getY() + imageBounds.getHeight()
- imgHeight, imgWidth / 2, imgHeight);
if (!loop.equals(" ")) {
canvas.drawImage(imageBounds, loop);
} else {
canvas.drawImage(imageBounds, compensation);
}
imageBounds.setRect(imageBounds.getX() + imgWidth / 2, imageBounds.getY() + imageBounds.getHeight() - imgHeight, imgWidth / 2,
imgHeight);
canvas.drawImage(imageBounds, marker);
imageBounds.setRect(imageBounds.getX() + imgWidth / 2, imageBounds.getY() + imageBounds.getHeight() - imgHeight, imgWidth / 2,
imgHeight);
canvas.drawImage(imageBounds, adHoc);
}
} else {
imageBounds.setRect(imageBounds.getX() + (imageBounds.getWidth() - imgWidth) / 2, imageBounds.getY() + imageBounds.getHeight()
- imgHeight, imgWidth / 2, imgHeight);
canvas.drawImage(imageBounds, marker);
imageBounds.setRect(imageBounds.getX() + imgWidth / 2, imageBounds.getY() + imageBounds.getHeight() - imgHeight, imgWidth / 2,
imgHeight);
canvas.drawImage(imageBounds, adHoc);
}
} else {
if (!loop.equals(" ") || !compensation.equals(" ")) {
if (!loop.equals(" ") && !compensation.equals(" ")) {
imageBounds.setRect(imageBounds.getX() + (2 * imageBounds.getWidth() - 3 * imgWidth) / 4, imageBounds.getY() + imageBounds.getHeight()
- imgHeight, imgWidth / 2, imgHeight);
canvas.drawImage(imageBounds, loop);
imageBounds.setRect(imageBounds.getX() + imgWidth / 2, imageBounds.getY() + imageBounds.getHeight() - imgHeight, imgWidth / 2,
imgHeight);
canvas.drawImage(imageBounds, compensation);
imageBounds.setRect(imageBounds.getX() + imgWidth / 2, imageBounds.getY() + imageBounds.getHeight() - imgHeight, imgWidth / 2,
imgHeight);
canvas.drawImage(imageBounds, marker);
} else {
imageBounds.setRect(imageBounds.getX() + (imageBounds.getWidth() - imgWidth) / 2, imageBounds.getY() + imageBounds.getHeight()
- imgHeight, imgWidth / 2, imgHeight);
if (!loop.equals(" ")) {
canvas.drawImage(imageBounds, loop);
} else {
canvas.drawImage(imageBounds, compensation);
}
imageBounds.setRect(imageBounds.getX() + imgWidth / 2, imageBounds.getY() + imageBounds.getHeight() - imgHeight, imgWidth / 2,
imgHeight);
canvas.drawImage(imageBounds, marker);
}
} else {
imageBounds.setRect(imageBounds.getX() + (2 * imageBounds.getWidth() - imgWidth) / 4, imageBounds.getY() + imageBounds.getHeight()
- imgHeight, imgWidth / 2, imgHeight);
canvas.drawImage(imageBounds, marker);
}
}
}
}
public int getArcSize(int w, int h) {
return Constants.RECTANGLE_ARCSIZE;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy