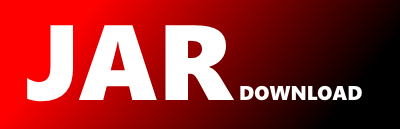
org.yaoqiang.graph.util.TooltipBuilder Maven / Gradle / Ivy
package org.yaoqiang.graph.util;
import java.util.Iterator;
import java.util.LinkedHashMap;
import java.util.Map;
import org.w3c.dom.Element;
import org.yaoqiang.graph.view.YGraph;
import com.mxgraph.model.mxCell;
import com.mxgraph.util.mxUtils;
/**
* TooltipBuilder
*
* @author Shi Yaoqiang([email protected])
*/
public class TooltipBuilder {
public static final String EMPTY_STRING = "";
public static final String EMPTY_VALUE = "empty";
public static final String HTML_OPEN = "";
public static final String HTML_CLOSE = "";
public static final String DIV_OPEN = "";
public static final String DIV_CLOSE = "";
public static final String STRONG_OPEN = "";
public static final String STRONG_CLOSE = "";
public static final String LINE_BREAK = "
";
public static final String COLON_SPACE = ": ";
public String getTooltip(YGraph graph, mxCell cell) {
Map toDisplay = new LinkedHashMap();
String title = "Element";
String process = cell.getAttribute("process");
String attached = cell.getAttribute("attached");
String call = cell.getAttribute("call");
String cancelActivity = "";
if (graph.isAttachedIntermediateEvent(cell)) {
cancelActivity = graph.cancelActivity(cell) ? "true" : "false";
}
String compensation = "";
if ((graph.isTask(cell) || graph.isSubProcess(cell) || graph.isCallActivityProcess(cell) || graph.isCallActivity(cell))
&& !graph.isChoreographySubprocess(cell) && !graph.isChoreographyTask(cell)) {
compensation = graph.isForCompensation(cell) ? "true" : "false";
}
String loop = "";
String sequential = "";
String looptype = mxUtils.getString(graph.getCellStyle(cell), Constants.STYLE_LOOP, "");
if (looptype.equals(Constants.ACTIVITY_STYLE_LOOP_STANDARD)) {
loop = "Standard";
} else if (looptype.equals(Constants.ACTIVITY_STYLE_LOOP_MI)) {
if (graph.isChoreographyTask(cell) || graph.isChoreographySubprocess(cell)) {
loop = "Parallel Multi-Instance";
} else {
loop = "Multi-Instance";
sequential = "false";
}
} else if (looptype.equals(Constants.ACTIVITY_STYLE_LOOP_MI_SEQUENTIAL)) {
if (graph.isChoreographyTask(cell) || graph.isChoreographySubprocess(cell)) {
loop = "Sequential Multi-Instance";
} else {
loop = "Multi-Instance";
sequential = "true";
}
}
String instantiate = "";
String eventGatewayType = "";
if (graph.isEventGateway(cell)) {
instantiate = Boolean.toString(graph.isInstantiateEventGateway(cell));
eventGatewayType = graph.isParallelEventGateway(cell) ? "Parallel" : "Exclusive";
}
if (graph.isReceiveTask(cell)) {
instantiate = Boolean.toString(graph.isInstantiateReceiveTask(cell));
}
String collection = graph.isDataObject(cell) ? Boolean.toString(graph.isCollectionDataObject(cell)) : "";
String source = cell.getSource() != null ? cell.getSource().getId() : "";
String target = cell.getTarget() != null ? cell.getTarget().getId() : "";
String value = cell.getValue() instanceof Element ? cell.getAttribute("value") : cell.getValue() != null ? cell.getValue().toString() : "";
if (graph.isPool(cell)) {
title = "Participant (Pool)";
} else if (graph.isLane(cell)) {
title = "Lane";
} else if (graph.isStartEvent(cell)) {
title = "Start Event";
if (graph.isMessageEvent(cell)) {
title = "Message Start Event";
} else if (graph.isTimerEvent(cell)) {
title = "Timer Start Event";
} else if (graph.isEscalationEvent(cell)) {
title = "Escalation Start Event";
} else if (graph.isConditionalEvent(cell)) {
title = "Conditional Start Event";
} else if (graph.isErrorEvent(cell)) {
title = "Error Start Event";
} else if (graph.isCompensationEvent(cell)) {
title = "Compensation Start Event";
} else if (graph.isSignalEvent(cell)) {
title = "Signal Start Event";
} else if (graph.isMultipleEvent(cell)) {
title = "Multiple Start Event";
} else if (graph.isParallelMultipleEvent(cell)) {
title = "Parallel Multiple Start Event";
}
} else if (graph.isIntermediateEvent(cell)) {
if (graph.isAttachedIntermediateEvent(cell)) {
title = "Boundary Event";
if (graph.isMessageEvent(cell)) {
title = "Boundary Message Event";
} else if (graph.isTimerEvent(cell)) {
title = "Boundary Timer Event";
} else if (graph.isEscalationEvent(cell)) {
title = "Boundary Escalation Event";
} else if (graph.isConditionalEvent(cell)) {
title = "Boundary Conditional Event";
} else if (graph.isErrorEvent(cell)) {
title = "Boundary Error Event";
} else if (graph.isCancelEvent(cell)) {
title = "Boundary Cancel Event";
} else if (graph.isCompensationEvent(cell)) {
title = "Boundary Compensation Event";
} else if (graph.isSignalEvent(cell)) {
title = "Boundary Signal Event";
} else if (graph.isMultipleEvent(cell)) {
title = "Boundary Multiple Event";
} else if (graph.isParallelMultipleEvent(cell)) {
title = "Boundary Parallel Multiple Event";
}
} else {
title = "Intermediate Event";
if (graph.isMessageEvent(cell)) {
title = "Message Intermediate Event";
} else if (graph.isTimerEvent(cell)) {
title = "Timer Intermediate Event";
} else if (graph.isEscalationEvent(cell)) {
title = "Escalation Intermediate Event";
} else if (graph.isConditionalEvent(cell)) {
title = "Conditional Intermediate Event";
} else if (graph.isLinkEvent(cell)) {
title = "Link Intermediate Event";
} else if (graph.isCompensationEvent(cell)) {
title = "Compensation Intermediate Event";
} else if (graph.isSignalEvent(cell)) {
title = "Signal Intermediate Event";
} else if (graph.isMultipleEvent(cell)) {
title = "Multiple Intermediate Event";
} else if (graph.isParallelMultipleEvent(cell)) {
title = "Parallel Multiple Intermediate Event";
}
}
} else if (graph.isEndEvent(cell)) {
title = "End Event";
if (graph.isMessageEvent(cell)) {
title = "Message End Event";
} else if (graph.isEscalationEvent(cell)) {
title = "Escalation End Event";
} else if (graph.isErrorEvent(cell)) {
title = "Error End Event";
} else if (graph.isCancelEvent(cell)) {
title = "Cancel End Event";
} else if (graph.isCompensationEvent(cell)) {
title = "Compensation End Event";
} else if (graph.isSignalEvent(cell)) {
title = "Signal End Event";
} else if (graph.isMultipleEvent(cell)) {
title = "Multiple End Event";
} else if (graph.isTerminateEvent(cell)) {
title = "Terminate End Event";
}
} else if (graph.isGateway(cell)) {
title = "Exclusive Gateway";
if (graph.isExclusiveGateway(cell)) {
title = "Exclusive Gateway";
} else if (graph.isInclusiveGateway(cell)) {
title = "Inclusive Gateway";
} else if (graph.isParallelGateway(cell)) {
title = "Parallel Gateway";
} else if (graph.isComplexGateway(cell)) {
title = "Complex Gateway";
} else if (graph.isEventGateway(cell)) {
title = "Event-Based Gateway";
}
} else if (graph.isTask(cell)) {
title = "Task";
if (graph.isCallActivity(cell)) {
title = "Call Activity";
if (graph.isChoreographyTask(cell)) {
title = "Call Choreography";
}
} else {
if (graph.isChoreographyTask(cell)) {
title = "Choreography Task";
} else if (graph.isSendTask(cell)) {
title = "Send Task";
} else if (graph.isReceiveTask(cell)) {
title = "Receive Task";
} else if (graph.isServiceTask(cell)) {
title = "Service Task";
} else if (graph.isUserTask(cell)) {
title = "User Task";
} else if (graph.isScriptTask(cell)) {
title = "Script Task";
} else if (graph.isManualTask(cell)) {
title = "Manual Task";
} else if (graph.isBusinessRuleTask(cell)) {
title = "Business Rule Task";
}
}
} else if (graph.isSubProcess(cell)) {
title = "Sub-Process";
if (graph.isChoreographySubprocess(cell)) {
title = "Sub-Choreography";
}
} else if (graph.isCallActivityProcess(cell)) {
title = "Call Activity";
if (graph.isChoreographySubprocess(cell)) {
title = "Call Choreography";
}
} else if (graph.isChoreographyParticipant(cell)) {
title = "Participant";
} else if (graph.isConversation(cell)) {
title = "Conversation";
if (graph.isSubConversation(cell)) {
title = "Sub Conversation";
}
if (graph.isCallConversation(cell)) {
title = "Call Conversation";
}
} else if (graph.isGroupArtifact(cell)) {
title = "Group";
} else if (graph.isAnnotation(cell)) {
title = "Text Annotation";
} else if (graph.isDataObject(cell)) {
title = "Data Object";
if (graph.isDataStore(cell)) {
title = "Data Store";
} else if (graph.isDataInput(cell)) {
title = "Data Input";
} else if (graph.isDataOutput(cell)) {
title = "Data Output";
}
} else if (graph.isAssociation(cell)) {
title = "Association";
} else if (graph.isDataAssociation(cell)) {
title = "Data Association";
} else if (graph.isSequenceFlow(cell)) {
title = "Sequence Flow";
} else if (graph.isMessageFlow(cell)) {
title = "Message Flow";
} else if (graph.isMessage(cell)) {
title = "Message";
} else if (graph.isConversationLink(cell)) {
title = "Conversation Link";
} else if (graph.isCompensationAssociation(cell)) {
title = "Compensation Association";
}
toDisplay.put("title", title);
if (graph.isChoreographyParticipant(cell)) {
toDisplay.put("id", cell.getId().substring(cell.getId().indexOf("_part_") + 6));
} else {
toDisplay.put("id", cell.getId());
}
if (graph.isAnnotation(cell)) {
toDisplay.put("text", value);
} else if (graph.isDataObject(cell) && !graph.isDataStore(cell)) {
int index = value.lastIndexOf("\n[");
String state = "";
if (index > 0) {
toDisplay.put("name", value.substring(0, index));
state = value.substring(index + 2, value.length() - 1);
} else {
toDisplay.put("name", value);
}
toDisplay.put("state", state);
} else {
toDisplay.put("name", value);
}
if (process != null && process.length() != 0) {
toDisplay.put("processRef", process);
}
if (attached != null && attached.length() != 0) {
toDisplay.put("attachedToRef", attached);
}
if (cancelActivity != null && cancelActivity.length() != 0) {
toDisplay.put("cancelActivity", cancelActivity);
}
if (eventGatewayType != null && eventGatewayType.length() != 0) {
toDisplay.put("event gateway type", eventGatewayType);
}
if (instantiate != null && instantiate.length() != 0) {
toDisplay.put("instantiate", instantiate);
}
if (collection != null && collection.length() != 0) {
toDisplay.put("isCollection", collection);
}
if (compensation != null && compensation.length() != 0) {
toDisplay.put("isForCompensation", compensation);
}
if (loop != null && loop.length() != 0) {
toDisplay.put("loop type", loop);
if (sequential != null && sequential.length() != 0) {
toDisplay.put("isSequential", sequential);
}
}
if (call != null && call.length() != 0) {
toDisplay.put("calledElement", call);
}
if (source != null && source.length() != 0) {
toDisplay.put("sourceRef", source);
}
if (target != null && target.length() != 0) {
toDisplay.put("targetRef", target);
}
if (graph.isConditionalSequenceFlow(cell)) {
toDisplay.put("conditionExpression", mxUtils.getString(graph.getCellStyle(cell), Constants.STYLE_EXPRESSION, ""));
}
return makeTooltip(toDisplay);
}
/**
* Neat little thing. Makes HTML formated string for tooltip (made of property names and coresponding values).
*/
protected static String makeTooltip(Map elements) {
if (elements == null)
return "";
String s = HTML_OPEN;
Iterator> it = elements.entrySet().iterator();
Map.Entry me = it.next();
s += makeHtmlTitleLine(me.getValue());
while (it.hasNext()) {
me = it.next();
s += makeAnotherHtmlLine(me.getKey(), me.getValue());
}
s = s.substring(0, s.length() - LINE_BREAK.length());
s += HTML_CLOSE;
return s;
}
/** Helps when generating tooltip for some element title. */
protected static String makeHtmlTitleLine(String title) {
String textToAppend = "";
textToAppend += DIV_OPEN;
textToAppend += STRONG_OPEN;
textToAppend += title;
textToAppend += STRONG_CLOSE;
textToAppend += DIV_CLOSE;
return textToAppend;
}
/** Helps when generating tooltip for some element. */
protected static String makeAnotherHtmlLine(String label, String text) {
int MAX_LENGTH = 45;
int MAX_LINES_PER_TEXT = 0;
String textToAppend = "";
textToAppend += STRONG_OPEN;
textToAppend += label + COLON_SPACE;
textToAppend += STRONG_CLOSE;
String val = text;
val = val.replaceAll("<", "<");
val = val.replaceAll(">", ">");
int vl = val.length();
if (vl > MAX_LENGTH) {
String newVal = "";
int hm = vl / MAX_LENGTH;
for (int i = 0; i <= hm; i++) {
int startI = i * MAX_LENGTH;
int endI = (i + 1) * MAX_LENGTH;
if (endI > vl) {
endI = vl;
}
newVal = newVal + val.substring(startI, endI);
if (i == MAX_LINES_PER_TEXT) {
newVal = newVal + " ...";
break;
}
if (i < hm) {
newVal += LINE_BREAK;
newVal += makeEmptyHTMLText((label + COLON_SPACE).length());
}
}
val = newVal;
} else if (vl == 0) {
val = EMPTY_VALUE;
}
textToAppend += val;
textToAppend += LINE_BREAK;
return textToAppend;
}
protected static String makeEmptyHTMLText(int length) {
if (length < 0)
return null;
String es = "";
for (int i = 0; i < length; i++) {
es += " ";
}
return es;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy